C++ String Exercises: Check a string is a title cased string or not
C++ String: Exercise-26 with Solution
The string where words start with an uppercase character and the remaining characters are lowercase is called the title cased version of the string
Write a C++ program to check if a given string is a title-cased string or not. When the string is title cased, return "True", otherwise return "False".
Sample Data:
(“The Quick Brown Fox.”) -> "True"
("C++ String exercises") -> "False"
("red, green, white") -> "False"
Sample Solution-1:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
string test(string text) { // Function to check if the given string is title cased or not
for (int i = 0; i < text.length(); i++) { // Loop through each character in the string
if (text[i - 1] == ' ' || i == 0) { // If the current character is the first character or preceded by a space
if (text[i] < 'A' || text[i] > 'Z') { // If the character is not an uppercase letter
return "False"; // Return "False" as it's not title cased
}
}
}
return "True"; // If all characters fit the conditions, return "True" as it's title cased
}
int main() {
string text = "The Quick Brown Fox."; // Declare and initialize a string
// string text = "C++ String exercises";
// string text = "red, green, white";
cout << "Original String:\n";
cout << text; // Output the original string
cout << "\n\nCheck the said string is a title cased string or not!\n";
cout << test(text) << endl; // Output the result of whether the string is title cased or not
}
Sample Output:
Original String: The Quick Brown Fox. Check the said string is a title cased string or not! True
Flowchart:
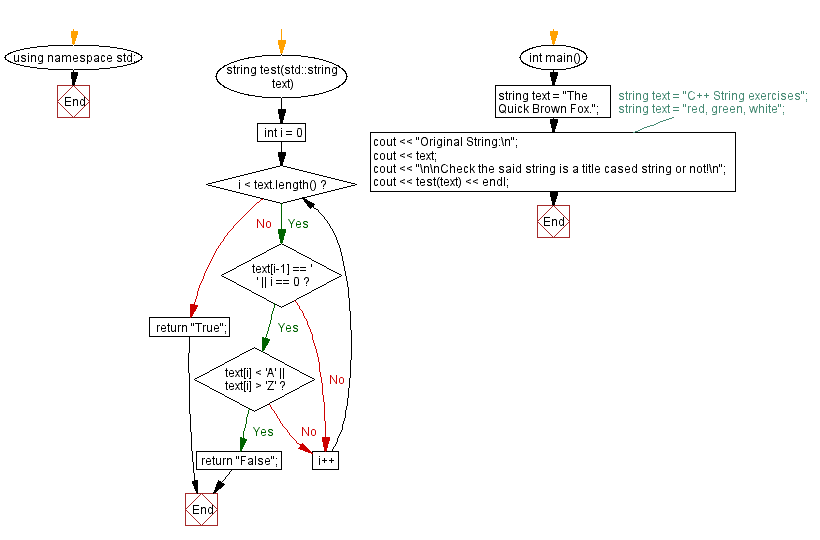
Sample Solution-2:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
string test(string text) { // Function to check if the given string is title cased or not
for (int i = 1; i < text.length(); i++) { // Loop through each character starting from the second character
if (!isupper(text[i]) && text[i - 1] == ' ') // If the current character is not uppercase and the previous character is a space
return "False"; // Return "False" as it's not title cased
}
return "True"; // If all characters fit the conditions, return "True" as it's title cased
}
int main() {
//string text = "The Quick Brown Fox.";
string text = "C++ String exercises"; // Declare and initialize a string
//string text = "red, green, white";
cout << "Original String:\n";
cout << text; // Output the original string
cout << "\n\nCheck the said string is a title cased string or not!\n";
cout << test(text) << endl; // Output the result of whether the string is title cased or not
}
Sample Output:
Original String: C++ String exercises Check the said string is a title cased string or not! False
Flowchart:
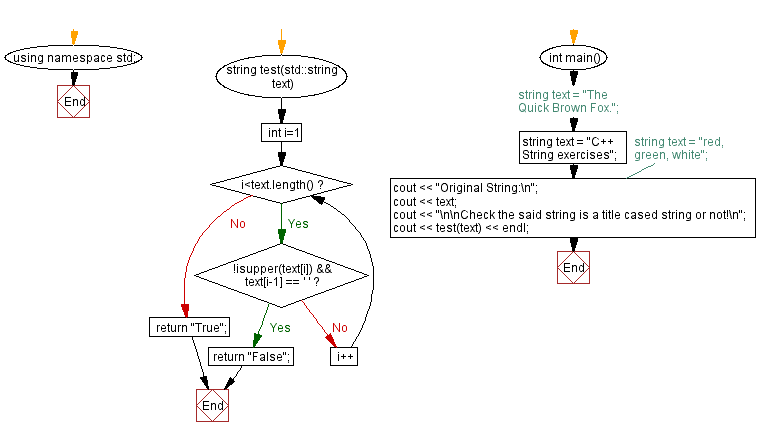
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Longest consecutive ones in a binary string.
Next C++ Exercise: Insert white spaces between lower and uppercase Letters.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics