C++ String Exercises: Extract the first n number of vowels from a string
C++ String: Exercise-28 with Solution
A vowel is a syllabic speech sound pronounced without any stricture in the vocal tract. Vowels are one of the two principal classes of speech sounds, the other being the consonant.
Write a C++ program to extract the first specified number of vowels from a given string. If the specified number is less than the number of vowels present in the text, display "n is less than the number of vowels present in the string".
Sample Data:
(“The quick brown fox.”, 2) -> eu
(“CPP Exercises”, 3) -> eie
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to extract the first 'n' number of vowels from the input string
string test(string text, int n) {
string b; // Initialize an empty string 'b' to store extracted vowels
int k = 0; // Initialize a counter 'k' for the number of extracted vowels
// Loop through the string to find the first 'n' vowels
for (int i = 0; i < text.size() && k < n; i++) {
// Check if the current character is a vowel (a, e, i, o, u)
if (text[i] == 'a' || text[i] == 'e' || text[i] == 'i' || text[i] == 'o' || text[i] == 'u') {
k++; // Increment the counter for each found vowel
b.push_back(text[i]); // Append the vowel to the 'b' string
}
}
// Check if 'n' is less than the number of vowels found in the string
if (k < n) {
return "n is less than the number of vowels present in the string!";
} else {
return b; // Return the extracted vowel string 'b'
}
}
int main() {
string text; // Declare a string variable to store user input
int n; // Declare an integer variable to store user input for 'n'
cout << "Input a string: ";
getline(cin, text); // Input a string from the user
cout << "Input a number: ";
cin >> n; // Input an integer from the user
cout << "\nExtract the first n number of vowels from the said string:\n";
cout << test(text, n) << endl; // Output the extracted vowels or a message indicating insufficient vowels
}
Sample Output:
Input a string: Input a number: Extract the first n number of vowels from the said string: n is less than number of vowels present in the string!
Flowchart:
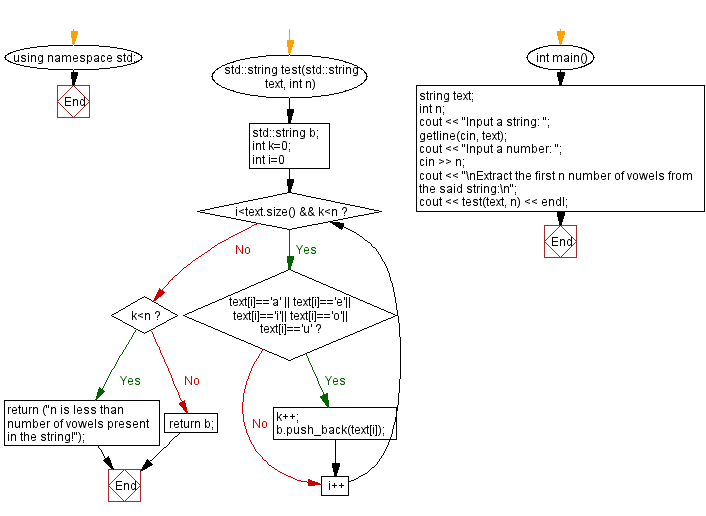
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Insert white spaces between lower and uppercase Letters.
Next C++ Exercise: Print an integer with commas as thousands separators.What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics