C#: Accept two integers and return true if either one is 5 or their sum or difference is 5
C# Sharp Basic Algorithm: Exercise-41 with Solution
Check for 5 or Sum/Difference Equals 5
Write a C# Sharp program that accepts two integers and returns true if either is 5 or their sum or difference is 5.
Visual Presentation:
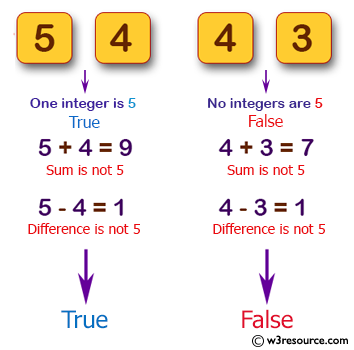
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and printing the results
Console.WriteLine(test(5, 4)); // Output: True
Console.WriteLine(test(4, 3)); // Output: False
Console.WriteLine(test(1, 4)); // Output: True
Console.ReadLine(); // Keeping the console window open
}
// Method to check different conditions involving two integers
public static bool test(int x, int y)
{
// Check if any of the following conditions are true:
// 1. 'x' is equal to 5
// 2. 'y' is equal to 5
// 3. The sum of 'x' and 'y' is equal to 5
// 4. The absolute difference between 'x' and 'y' is equal to 5
return x == 5 || y == 5 || x + y == 5 || Math.Abs(x - y) == 5;
}
}
}
Sample Output:
True False True
Flowchart:
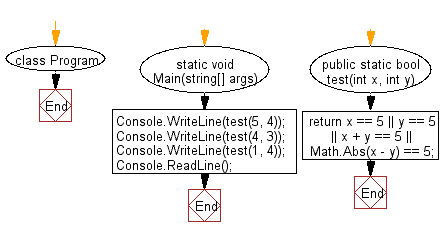
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Next: Write a C# Sharp program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-41.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics