C#: Check if a given string is a palindrome or not
Check Palindrome String
Write a C# program to check if a given string is a palindrome or not.
Sample Example:
For 'aba' the output should be true
For 'abcd' the output should be false
Sample Solution:
C# Sharp Code:
using System;
public class Example
{
// Function to check if a string is a palindrome
public static bool checkPalindrome(string inputString)
{
// Convert the input string into a character array
char[] c = inputString.ToCharArray();
// Reverse the character array
Array.Reverse(c);
// Check if the reversed string is equal to the original input string
return new string(c).Equals(inputString);
}
// Main method to test the checkPalindrome function
public static void Main()
{
// Testing the checkPalindrome function with different input strings
Console.WriteLine(checkPalindrome("aaa")); // Output: True
Console.WriteLine(checkPalindrome("abc")); // Output: False
Console.WriteLine(checkPalindrome("madam")); // Output: True
Console.WriteLine(checkPalindrome("1234")); // Output: False
}
}
Sample Output:
True False True False
Visual Presentation:
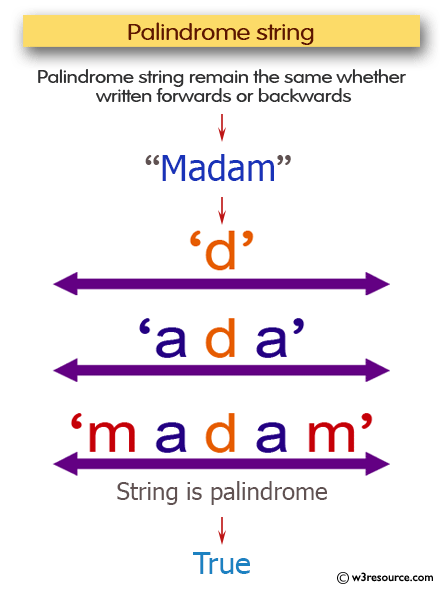
Flowchart:
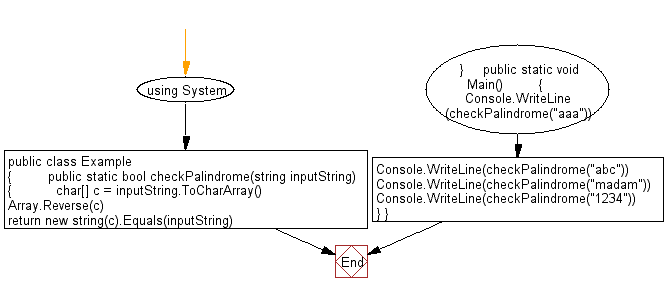
C# Sharp Code Editor:
Previous: Write a C# program to find the pair of adjacent elements that has the largest product of an given array.
Next: Write a C# program to find the pair of adjacent elements that has the highest product of an given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.