C#: Add seconds to a DateTime value
Write C# Sharp Program to add 30 seconds and the number of seconds in one day to a DateTime value.
Note: It displays each new value and displays the difference between it and the original value. The difference is displayed both as a time span and as a number of ticks.
Sample Solution:-
C# Sharp Code:
using System;
public class Example13
{
// Main method, entry point of the program
public static void Main()
{
// Date format string for displaying dates without milliseconds
string dateFormat = "MM/dd/yyyy hh:mm:ss";
// Create a DateTime object 'date1' set to August 15, 2016, 4:00:00 PM
DateTime date1 = new DateTime(2016, 8, 15, 16, 0, 0);
Console.WriteLine("Original date: {0} ({1:N0} ticks)\n",
date1.ToString(dateFormat), date1.Ticks);
// Create 'date2' by adding 30 seconds to 'date1'
DateTime date2 = date1.AddSeconds(30);
Console.WriteLine("Second date: {0} ({1:N0} ticks)",
date2.ToString(dateFormat), date2.Ticks);
Console.WriteLine("Difference between dates: {0} ({1:N0} ticks)\n",
date2 - date1, date2.Ticks - date1.Ticks);
// Create 'date3' by adding 1 day (24 hours) to 'date1'
DateTime date3 = date1.AddSeconds(60 * 60 * 24); // 60 secs * 60 mins * 24 hrs
Console.WriteLine("Third date: {0} ({1:N0} ticks)",
date3.ToString(dateFormat), date3.Ticks);
Console.WriteLine("Difference between dates: {0} ({1:N0} ticks)",
date3 - date1, date3.Ticks - date1.Ticks);
}
}
Sample Output:
Original date: 08/15/2016 04:00:00 (636,068,736,000,000,000 ticks) Second date: 08/15/2016 04:00:30 (636,068,736,300,000,000 ticks) Difference between dates: 00:00:30 (300,000,000 ticks) Third date: 08/16/2016 04:00:00 (636,069,600,000,000,000 ticks) Difference between dates: 1.00:00:00 (864,000,000,000 ticks)
Flowcharts:
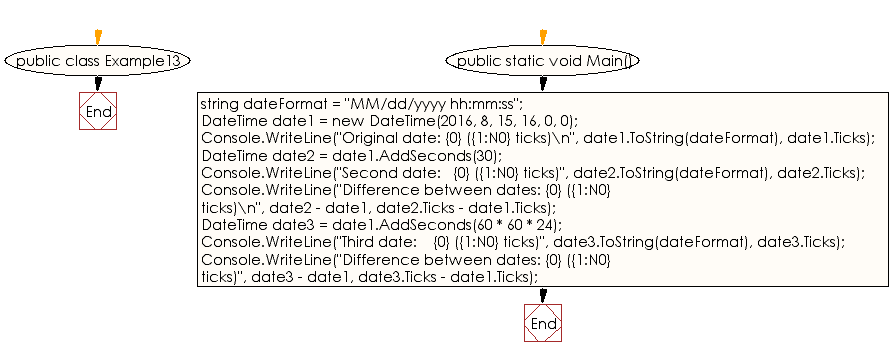
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write C# Sharp Program to add one millisecond and 2.5 milliseconds to a given date value and display each new value and the difference between it and the original value.
Next: Write a C# Sharp program to add specified number of months between zero and fifteen months to the last day of August, 2016.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.