C#: Remove special characters from a given text
Write a C# Sharp program to remove special characters from given text. Return the updated string which allows alphanumeric characters, spaces, underscores _ and dashes -.
Note: A special character is a character that is not an alphabetic or numeric character. Special characters are: ., !, @, #, $, %, ^, &, \, *, (, )
Sample Data:
("AA@%^&CC") -> "AACC"
("Python") -> "Python"
("w3resource.com") -> "w3resourcecom"
Sample Solution-1:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Define and initialize test strings
string text1 = "AA@%^&CC";
Console.WriteLine("Original string: " + text1);
// Call the test method and display the modified string
Console.WriteLine("New string: " + test(text1));
// Update the test string
text1 = "Python";
Console.WriteLine("Original string: " + text1);
// Call the test method and display the modified string
Console.WriteLine("New string: " + test(text1));
// Update the test string
text1 = "w3resource.com";
Console.WriteLine("Original string: " + text1);
// Call the test method and display the modified string
Console.WriteLine("New string: " + test(text1));
}
// Method to remove special characters from a string using Regex
public static string test(string text)
{
// Replace any character that is not a letter, digit, space, underscore, or hyphen with an empty string
return Regex.Replace(text, "[^0-9A-Za-z _-]", "");
}
}
}
Sample Output:
Original string: AA@%^&CC New string: AACC Original string: Python New string: Python Original string: w3resource.com New string: w3resourcecom
Flowchart:
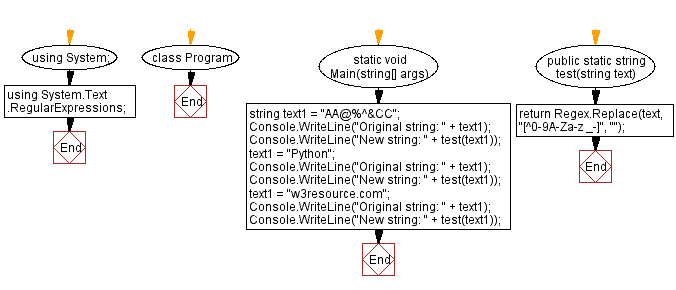
Sample Solution-2:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Define and initialize test strings
string text = "AA@%^&CC";
Console.WriteLine("Original string: " + text);
// Call the test method and display the modified string
Console.WriteLine("New string: " + test(text));
// Update the test string
text = "Python";
Console.WriteLine("Original string: " + text);
// Call the test method and display the modified string
Console.WriteLine("New string: " + test(text));
// Update the test string
text = "w3resource.com";
Console.WriteLine("Original string: " + text);
// Call the test method and display the modified string
Console.WriteLine("New string: " + test(text));
}
// Method to remove special characters from a string using Regex
public static string test(string text)
{
// Replace any character that is not a letter, digit, space, underscore, or hyphen with an empty string
return Regex.Replace(text, @"[^\w \-]", "");
}
}
}
Sample Output:
Original string: AA@%^&CC New string: AACC Original string: Python New string: Python Original string: w3resource.com New string: w3resourcecom
Flowchart:
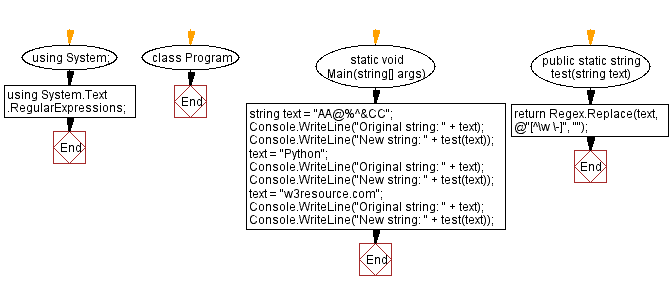
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Check whether a string represents a currency value.
Next C# Sharp Exercise: Find a specific word in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.