C#: Find common elements between two stacks
Write a C# program that implements a stack and finds common elements between two stacks.
Sample Solution:
C# Code:
using System;
using System.Collections.Generic;
public class Stack
{
private int[] items; // Array to store stack elements
private int top; // Index pointing to the top of the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Creating an array of integers with the given size
top = -1; // Initializing top index to -1 indicating an empty stack
}
// Check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Returns true if the stack is empty
}
// Check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Returns true if the stack is full
}
// Push an element onto the stack
public void Push(int item)
{
if (IsFull()) // Check if the stack is full
{
Console.WriteLine("Stack Full!"); // Display a message indicating stack overflow
return;
}
items[++top] = item; // Increment top and add the item to the stack
}
// Pop an element from the stack
public int Pop()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack underflow"); // Display a message indicating stack underflow
return -1;
}
return items[top--]; // Return and decrement top to remove the element from the stack
}
// Peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack is empty"); // Display a message indicating the stack is empty
return -1;
}
return items[top]; // Return the top element of the stack
}
// Static method to get the size of a stack
public static int Size(Stack stack)
{
return stack.top + 1; // Return the size of the stack based on the current top index
}
// Method to count all the elements in a stack
public static int Count(Stack stack)
{
int count = 0;
Stack temp = new Stack(Size(stack));
// Move elements from the original stack to a temporary stack and count them
while (!stack.IsEmpty())
{
temp.Push(stack.Pop()); // Move elements from original stack to temporary stack
count++; // Increment count for each element moved
}
// Restore the original stack by moving elements back from the temporary stack
while (!temp.IsEmpty())
{
stack.Push(temp.Pop()); // Move elements back to the original stack
}
return count; // Return the total count of elements in the stack
}
// Method to check if an element is present or not in a stack
public bool Contains(int item)
{
for (int i = 0; i <= top; i++)
{
if (items[i] == item)
{
return true; // Return true if the element is found in the stack
}
}
return false; // Return false if the element is not found in the stack
}
// Find common elements between two stacks
public static Stack FindCommonElements(Stack stack1, Stack stack2)
{
Stack commonElements = new Stack(Math.Min(Size(stack1), Size(stack2)));
// Iterate through stack1 and check for common elements with stack2
for (int i = 0; i <= stack1.top; i++)
{
int item = stack1.items[i];
// Check if the element exists in stack2 and is not already present in commonElements
if (stack2.Contains(item) && !commonElements.Contains(item))
{
commonElements.Push(item); // Add the common element to the commonElements stack
}
}
return commonElements; // Return the stack containing common elements
}
// Method to display the elements of the stack
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return;
}
Console.WriteLine("Stack elements:");
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " ");
}
}
}
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize three stacks:");
// Create and display the first stack
Stack stack1 = new Stack(10);
stack1.Push(1);
stack1.Push(2);
stack1.Push(3);
Console.Write("Stack1: ");
Stack.Display(stack1);
// Create and display the second stack
Stack stack2 = new Stack(10);
stack2.Push(2);
stack2.Push(3);
stack2.Push(4);
stack2.Push(5);
Console.Write("\nStack2: ");
Stack.Display(stack2);
// Create and display the third stack
Stack stack3 = new Stack(10);
stack3.Push(3);
stack3.Push(4);
stack3.Push(5);
Console.Write("\nStack3: ");
Stack.Display(stack3);
// Find and display common elements between Stack1 and Stack2
Stack commonStack1and2 = Stack.FindCommonElements(stack1, stack2);
Console.Write("\n\nCommon elements between Stack1 and Stack2: ");
Stack.Display(commonStack1and2);
// Find and display common elements between Stack1 and Stack3
Stack commonStack1and3 = Stack.FindCommonElements(stack1, stack3);
Console.Write("\n\nCommon elements between Stack1 and Stack3: ");
Stack.Display(commonStack1and3);
// Find and display common elements between Stack2 and Stack3
Stack commonStack2and3 = Stack.FindCommonElements(stack2, stack3);
Console.Write("\n\nCommon elements between Stack2 and Stack3: ");
Stack.Display(commonStack2and3);
}
}
Sample Output:
Initialize three stacks: Stack1: Stack elements: 3 2 1 Stack2: Stack elements: 5 4 3 2 Stack3: Stack elements: 5 4 3 Common elements between Stack1 and Stack2: Stack elements: 3 2 Common elements between Stack1 and Stack3: Stack elements: 3 Common elements between Stack2 and Stack3: Stack elements: 5 4 3
Flowchart:
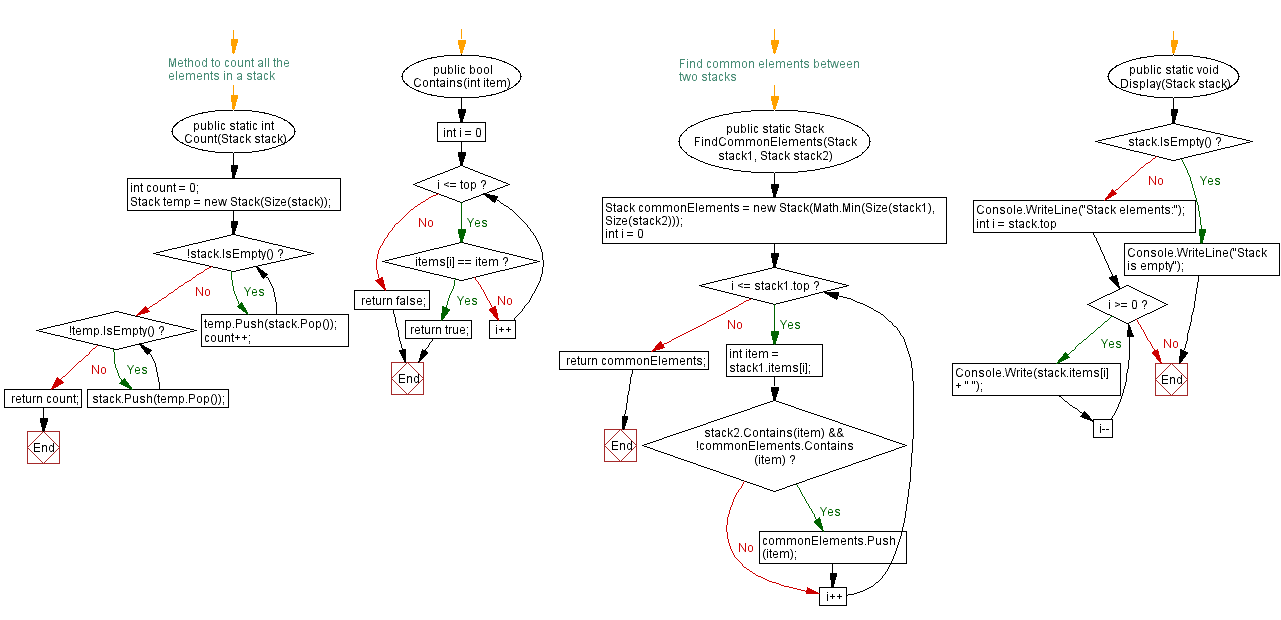
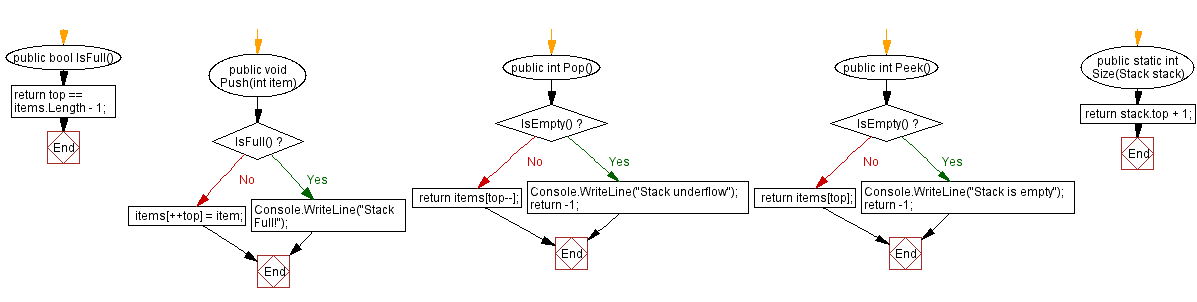
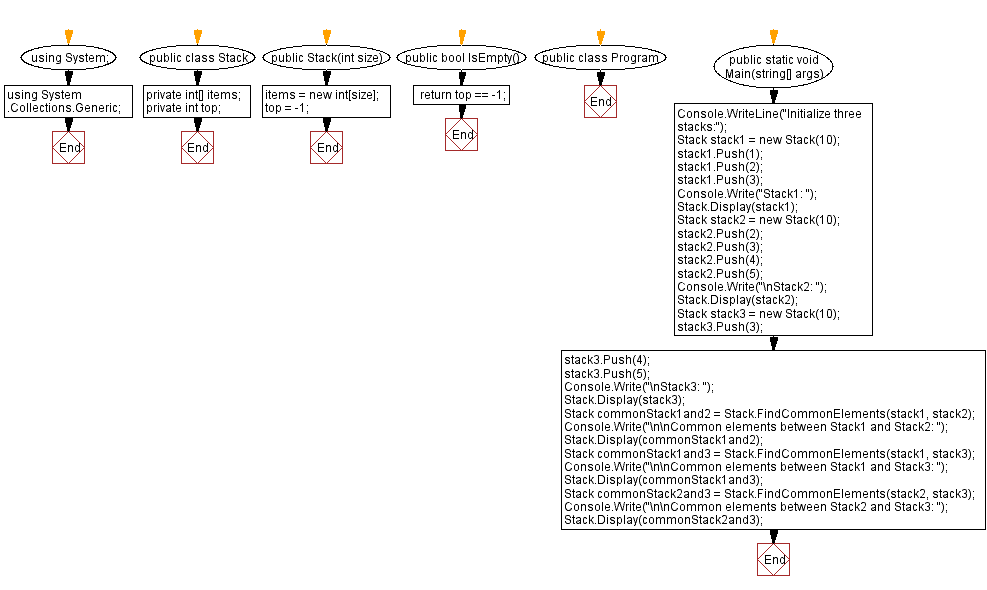
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Checks if two stacks are equal.
Next: Stack elements in the first but not in the second.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.