Maximizing Water Storage: Two-Pointer Technique Explained
Problem Statement
Container With Most Water:
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai), n vertical lines are drawn such that the two endpoints of the line i are at (i, ai) and (i, 0). Find two lines that, together with the x-axis, form a container containing the most water.
- Introduction to the Problem
- Underlying Concepts
- Examples and Illustrations
- Brute Force Approach
- Optimization Techniques
- Algorithmic Thinking
- Algorithmic Strategies
- Common Mistakes and Pitfalls
- Coding Best Practices
- Testing and Debugging
- Algorithm Analysis
- Application in Real-world Projects
- Example test cases
- Solution for different languages
- Time and Space Complexity
Introduction to the Problem:
Problem Statement:
Given a set of non-negative integers representing the heights of vertical lines at different positions, the task is to determine the two lines that, along with the x-axis, form a container that contains the most water. The goal is to find the maximum area enclosed by any two lines on the graph.
Input and Output Requirements:
- Input: An array of non-negative integers representing the heights of vertical lines. Each integer represents the height of a line at a specific position.
- Output: The maximum area of water that can be contained by any two lines and the x-axis.
Real-world Scenario:
One real-world scenario where this problem might arise is in urban planning or architectural design, specifically when designing drainage systems or rainwater harvesting solutions. In such cases, engineers or planners need to determine the optimal placement of drainage channels or reservoirs to efficiently collect and store rainwater while minimizing the use of space.
By solving the "Container With Most Water" problem, they can identify the locations where most water can be contained within a given area, helping them make informed decisions to optimize water management systems.
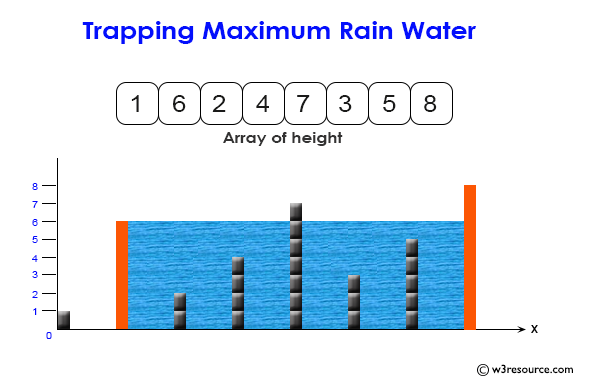
Underlying Concepts:
- Area calculation:
- Area calculation in the context of the "Container With Most Water" problem refers to determining the area formed by two vertical lines and the x-axis. This area represents the amount of water contained within the container formed by the two lines. The formula to calculate the area is:
Area = Width×Height
Where: - Width: The horizontal distance between the two vertical lines.
- Height: The minimum height of the two vertical lines.
- Constraints:
- Constraints refer to any limitations or conditions imposed on the problem. In the context of the "Container With Most Water" problem, one of the primary constraints is that the heights of the vertical lines are non-negative integers. This means that the heights cannot be negative, as negative heights would not make sense in the context of the problem. Additionally, the problem typically assumes a finite number of vertical lines represented by the input array a, denoted as a1,2,...,an a1,a2,...,an where n is the number of lines.
Examples and Illustrations:
- Walkthrough examples:
Suppose we have the following input array representing the heights of vertical lines: [1,8,6,2,5,4,8,3,7]
We can walk through this example to demonstrate how to approach the problem: - Start with two pointers, one at the beginning (left) of the array and the other at the end (right) of the array.
- Calculate the area formed by the lines at the current positions of the pointers.
- Move the pointer with the shorter line towards the center.
- Recalculate the area and update the maximum area if the new area is greater.
- Repeat this process until the pointers meet.
- Visualization: We can use a visual aid like a graph to illustrate how the container's area changes with different pairs of lines. For example, consider the same input array as before:
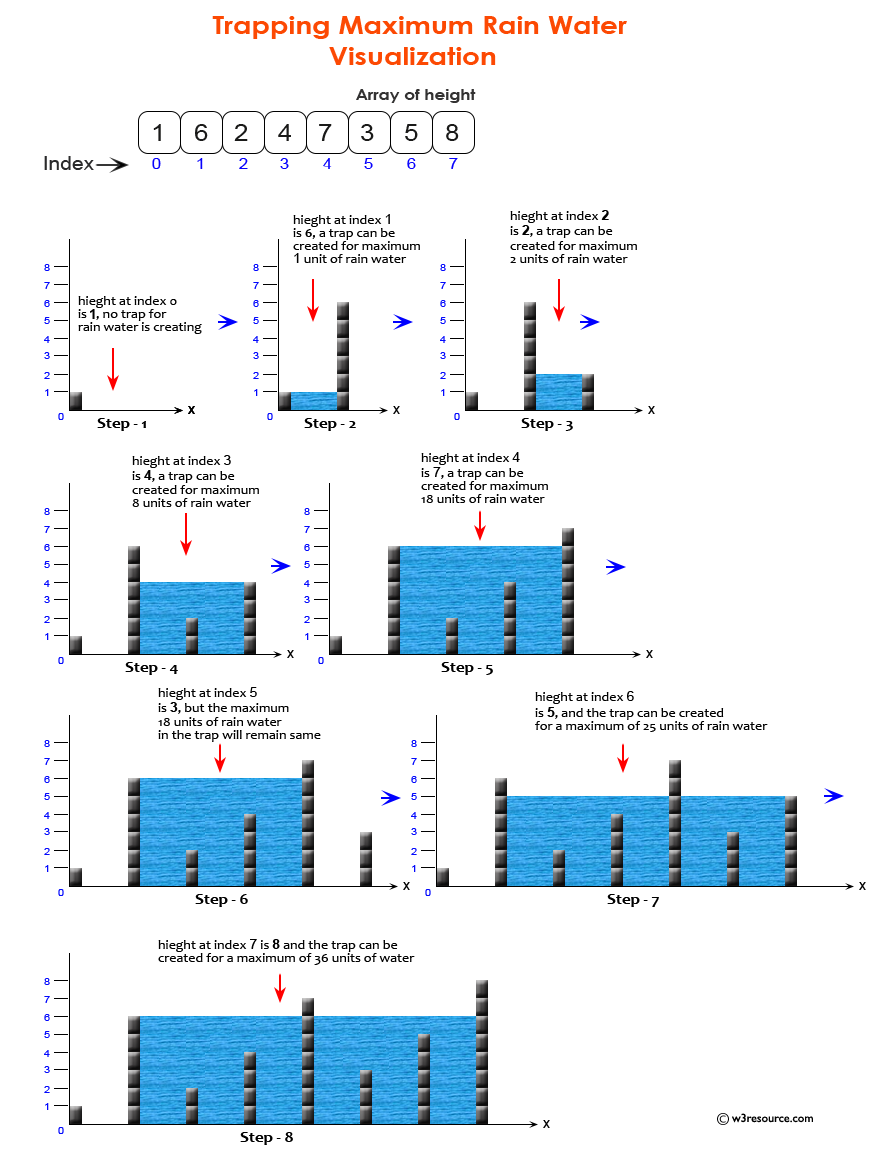
Brute Force Approach:
Naive Solution:
- The naive or brute force solution to the "Container With Most Water" problem involves iterating through all possible pairs of lines and calculating the area formed by each pair. For each pair of lines, the width is determined by the difference in their indices, and the height is determined by the minimum height of the two lines. By iterating through all possible pairs of lines, we can find the maximum area among them.
Complexity Analysis:
- The time complexity of the brute force approach is O(n2), where 𝑛n is the number of lines. This is because we need to consider all possible pairs of lines, resulting in nested loops.
- The space complexity of the brute force approach is O(1) since we only need a constant amount of extra space for variables to store the maximum area and indices of the lines forming the container. However, if the input array is considered part of the space complexity, it would be O(n).
Optimization Techniques:
- Two Pointer Technique:
- The two-pointer approach is an optimized solution for the "Container With Most Water" problem. It involves using two pointers, one starting from the beginning of the array and the other starting from the end. These pointers gradually move towards each other, and at each step, the width of the container decreases (since the pointers move closer), but the goal is to maximize the height of the container.
- The key insight is that the maximum area can only be increased if we move the pointer with the smaller height towards the center, as it may potentially find a taller line that can form a larger container. This approach avoids the need to consider all possible pairs of lines, significantly reducing the number of computations needed.
- Complexity Analysis:
- The time complexity of the two-pointer approach is O(n), where 𝑛n is the number of lines. This is because we iterate through the array once using the two pointers, and each pointer moves at most n steps.
- The space complexity of the two-pointer approach is O(1) since we only need a constant amount of extra space for variables to store the pointers and other necessary variables. Compared to the brute force approach, which has a space complexity of O(n) due to the input array, the two-pointer approach is more space-efficient.
Algorithmic Thinking:
- Greedy Strategy:
- The greedy strategy involves making decisions that seem locally optimal at each step with the hope of finding a global optimum. In the context of the "Container With Most Water" problem, the greedy approach entails maximizing the area between two lines at each step.
This means choosing the pair of lines that promises the highest increase in area compared to the previous pair. By continually selecting the pair of lines with the maximum potential area increase, the algorithm aims to reach the overall maximum area efficiently.
Algorithmic Strategies:
- Algorithmic strategies refer to the techniques and methods used to devise efficient solutions to computational problems. In the case of the "Container With Most Water" problem, algorithmic strategies involve identifying and utilizing patterns or insights in the problem to improve the efficiency of the solution.
This includes techniques such as the two-pointer approach, which exploits the characteristics of the problem to reduce time complexity, or dynamic programming, which stores and reuses intermediate results to optimize the solution. By employing algorithmic strategies, we can develop algorithms that efficiently solve complex problems by leveraging key insights and patterns.
Common Mistakes and Pitfalls:
Incorrect Pointer Movement:
- Moving Both Pointers Towards Each Other Irrespective of Heights:
- Mistake: Moving both pointers towards each other without considering the heights of the lines they point to.
- Implication: This approach may miss the optimal solution. It fails to leverage the potential increase in area by moving the pointer associated with the shorter line towards the taller line.
- Moving Only One Pointer Based on Local Comparison:
- Mistake: Moving only one pointer (either left or right) based on a local comparison of adjacent line heights.
- Implication: This strategy may overlook better solutions. It does not consider the possibility of a taller line beyond the adjacent one, leading to suboptimal area calculations.
- Incorrectly Updating Pointers Based on Heights:
- Mistake: Updating pointers inconsistently based on height comparisons, e.g., always moving the pointer associated with the taller line or the shorter line without considering the context.
- Implication: This approach may result in missing the maximum area. It fails to adaptively adjust pointer movement to maximize the area between lines effectively.
- Not Considering All Possible Pair Combinations:
- Mistake: Failing to explore all possible pair combinations by prematurely converging pointers.
- Implication: This oversight can lead to missing the global optimum. It restricts the search space, potentially excluding pairs of lines that could form a container with a larger area.
Misinterpreting Constraints:
- Assuming Negative Heights:
- Mistake: Incorrectly assuming that the heights of the vertical lines can be negative.
- Implication: Negative heights are not valid in the context of the problem. Assuming negative heights can lead to erroneous calculations and solutions that do not align with the problem's requirements.
- Invalid Inputs:
- Mistake: Failing to handle or validate invalid inputs, such as non-numeric values or an empty input array.
- Implication: Invalid inputs can cause runtime errors or unexpected behavior in the solution algorithm. Without proper validation, the algorithm may produce incorrect results or encounter exceptions during execution.
Coding Best Practices:
Container With Most Water Problem:
- Modularity:
- Break down the solution into modular functions or methods, each addressing a specific subtask.
- Encapsulate logic related to specific functionalities within dedicated functions.
- Improved readability: Each function focuses on a specific task, making the code easier to understand.
- Reusability: Modular functions can be reused in other parts of the code or in different projects.
- Easy debugging: Isolate and troubleshoot issues in specific modules without affecting the entire codebase. Example (Python code):
- Example (Python code):
- Advantages of Modularity:
def find_container_with_most_water(height):
# Function for finding the container with the most water
# ...
def main():
# Main function to orchestrate the program
# ...
if __name__ == "__main__":
main()
- Variable Naming:
- Choose descriptive and meaningful variable names that convey the purpose or content of the variable.
- Avoid single-letter variable names unless they represent common conventions (e.g., loop indices).
- Readability: Clear and descriptive names enhance code readability for yourself and others.
- Maintenance: Easier maintenance as the purpose of variables is evident.
- Reduced comments: Well-named variables can reduce the need for excessive comments.
- Example (Python code):
Benefits of Good Variable Naming:
def find_container_with_most_water(height):
# height: List of non-negative integers representing the heights of vertical lines
# ...
for index, h in enumerate(height):
# ...
- Maintain a consistent coding style throughout the codebase.
- Follow established conventions, such as PEP 8 for Python, for a uniform appearance.
- Consistency facilitates version control and collaboration, especially in team environments.
- Adopting a consistent style ensures that team members can easily understand and contribute to the code.
- Example (Python code):
Version Control and Collaboration:
# Inconsistent Style
def findContainerWithMostWater(heights):
for i, h in enumerate(heights):
# ...
# Consistent Style (Following PEP 8)
def find_container_with_most_water(heights):
for i, height in enumerate(heights):
# ...
Testing and Debugging:
- Test cases:
- Provide a set of test cases to verify the correctness of the solution.
- Test cases should cover various scenarios, such as:
- A simple case with a small number of vertical lines.
- Cases with different heights of vertical lines.
- Edge cases where the heights of vertical lines are all the same or all different.
- Debugging techniques:
- Discuss common debugging techniques for identifying and fixing errors, particularly related to pointer manipulation in this problem.
- Techniques may include:
- Step-by-step execution using a debugger to trace the flow of execution and identify logical errors.
- Adding print statements to output intermediate values and verify the correctness of calculations.
- Visualizing the problem using diagrams or graphs to better understand the behavior of the algorithm.
- Checking boundary conditions and edge cases to ensure the solution handles all possible scenarios correctly.
Algorithm Analysis:
- Time and Space Complexity:
- Explain how to analyze the time and space complexity of the solution, focusing on the optimized "two-pointer" approach.
- Time Complexity:
- The optimized solution using the "two-pointer" approach has a time complexity of O(n), where n is the number of vertical lines.
- This is because the algorithm only requires a single pass through the array of vertical lines, comparing and updating the maximum area.
- Space Complexity:
- The space complexity of the optimized solution is O(1), indicating constant space usage.
- The algorithm does not require any additional data structures or memory allocation beyond a few variables to store pointers and the maximum area.
- Trade-offs:
- Discuss trade-offs between time and space complexity and how they influence algorithm selection.
- The "two-pointer" approach offers optimal time complexity of O(n) but sacrifices some readability compared to other approaches.
- However, the trade-off is justified as improved time complexity is crucial for handling large input sizes efficiently.
- By prioritizing time efficiency over space, the algorithm ensures fast execution even with large datasets.
- Additionally, the constant space complexity makes the algorithm more memory-efficient, suitable for applications with limited memory resources.
Application in Real-world Projects:
Practical Use Cases:
- In urban planning, algorithms similar to finding the container with the most water can be applied to optimize the placement of water reservoirs or rainwater harvesting systems.
- Similarly, in resource allocation, such algorithms can be used to optimize the distribution of resources in areas with varying demands, ensuring maximum utilization and efficiency.
Example test cases:
- Positive Case:
- Input: heights = [1, 8, 6, 2, 5, 4, 8, 3, 7]
- Expected Output: 49
- Explanation: The maximum area is achieved by selecting the lines at indices 1 and 8, forming a container with a height of 7 and width of 8, resulting in an area of 7 * 7 = 49.
- Another Positive Case:
- Input: heights = [4, 3, 2, 1, 5]
- Expected Output: 16
- Explanation: Water can be trapped between the first and fifth lines. The maximum amount of water trapped in this scenario would be 16 units, with a container formed by the first and fifth lines having a height of 4 and a width of 4.
- Edge Case:
- Input: heights = []
- Expected Output: 0
- Explanation: An empty array cannot form a container, so the maximum water trapped is 0.
Solution for different languages:
Python - Container With Most Water
Code:
def max_area(height):
max_area = 0
left = 0
right = len(height) - 1
while left < right:
# Calculate the area between the lines at left and right pointers
width = right - left
h = min(height[left], height[right])
area = width * h
# Update max_area if the current area is greater
max_area = max(max_area, area)
# Move the pointer with the smaller height inward
if height[left] < height[right]:
left += 1
else:
right -= 1
return max_area
# Test cases
print(max_area([1, 8, 6, 2, 5, 4, 8, 3, 7])) # Expected output: 49
print(max_area([4, 3, 2, 1, 5])) # Expected output: 16
print(max_area([5])) # Expected output: 0
print(max_area([])) # Expected output: 0
Output:
49 16 0 0
Explanation of the said Python code:
- Initialize 'max_area' to 0, 'left' to 0 (index of the leftmost line), and 'right' to 'len(height) - 1' (index of the rightmost line).
- Use a while loop to iterate until the 'left' pointer is less than the 'right' pointer.
- Inside the loop:
- Calculate the width of the container between the lines at the 'left' and 'right' pointers.
- Calculate the height of the container, which is the minimum of the heights of the lines at the 'left' and 'right' pointers.
- Calculate the area of the container using the width and height.
- Update 'max_area' if the current area is greater than the previous maximum.
- Move the pointer with the smaller height inward. If the height at the 'left' pointer is less than the height at the 'right' pointer, increment the 'left' pointer; otherwise, decrement the 'right' pointer.
- Return the 'max_area'.
Java - Container With Most Water
Code:
public class ContainerWithMostWater {
public static int maxArea(int[] height) {
int maxArea = 0;
int left = 0;
int right = height.length - 1;
while (left < right) {
// Calculate the area between the lines at left and right pointers
int width = right - left;
int h = Math.min(height[left], height[right]);
int area = width * h;
// Update maxArea if the current area is greater
maxArea = Math.max(maxArea, area);
// Move the pointer with the smaller height inward
if (height[left] < height[right]) {
left++;
} else {
right--;
}
}
return maxArea;
}
public static void main(String[] args) {
int[] heights1 = {1, 8, 6, 2, 5, 4, 8, 3, 7};
System.out.println(maxArea(heights1));
int[] heights2 = {4, 3, 2, 1, 5};
System.out.println(maxArea(heights2));
int[] heights3 = {};
System.out.println(maxArea(heights3));
int[] heights4 = {};
System.out.println(maxArea(heights4));
}
}
Output:
49 16 0 0
Explanation of the said Java code:
- The "maxArea()" method takes an integer array 'height' as input and returns the maximum area of water that can be trapped.
- Inside the method:
- Initialize 'maxArea' to 0, 'left' to 0 (index of the leftmost line), and 'right' to height.length - 1 (index of the rightmost line).
- Use a while loop to iterate until the 'left' pointer is less than the 'right' pointer.
- Calculate the width of the container between the lines at the 'left' and 'right' pointers.
- Calculate the height of the container, which is the minimum of the heights of the lines at the 'left' and 'right' pointers.
- Calculate the area of the container using the width and height.
- Update 'maxArea' if the current area is greater than the previous maximum.
- Move the pointer with the smaller height inward. If the height at the 'left' pointer is less than the height at the 'right' pointer, increment the 'left' pointer; otherwise, decrement the 'right' pointer.
- The "main()" method is used to demonstrate the usage of the "maxArea()" method with different test cases.
C++ - Container With Most Water
Code:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int maxArea(vector<int>& height) {
int maxArea = 0;
int left = 0;
int right = height.size() - 1;
while (left < right) {
// Calculate the area between the lines at left and right pointers
int width = right - left;
int h = min(height[left], height[right]);
int area = width * h;
// Update maxArea if the current area is greater
maxArea = max(maxArea, area);
// Move the pointer with the smaller height inward
if (height[left] < height[right]) {
left++;
} else {
right--;
}
}
return maxArea;
}
int main() {
vector<int> heights1 = {1, 8, 6, 2, 5, 4, 8, 3, 7};
cout <<; maxArea(heights1) << endl;
vector<int> heights2 = {4, 3, 2, 1, 5};
cout << maxArea(heights2) << endl;
vector<int> heights3 = {};
cout << maxArea(heights3) << endl;
vector<int> heights4 = {};
cout << maxArea(heights4) << endl;
return 0;
}
Output:
49 16 0 0
Explanation of the said C++ code:
- The "maxArea()" method takes an integer array 'height' as input and returns the maximum area of water that can be trapped.
- Inside the method:
- Initialize 'maxArea' to 0, 'left' to 0 (index of the leftmost line), and 'right' to height.length - 1 (index of the rightmost line).
- Use a while loop to iterate until the 'left' pointer is less than the 'right' pointer.
- Calculate the width of the container between the lines at the 'left' and 'right' pointers.
- Calculate the height of the container, which is the minimum of the heights of the lines at the 'left' and 'right' pointers.
- Calculate the area of the container using the width and height.
- Update 'maxArea' if the current area is greater than the previous maximum.
- Move the pointer with the smaller height inward. If the height at the 'left' pointer is less than the height at the 'right' pointer, increment the 'left' pointer; otherwise, decrement the 'right' pointer.
- The "main()" method is used to demonstrate the usage of the "maxArea()" method with different test cases.
C# - Container With Most Water
Code:
using System;
public class Solution
{
public int MaxArea(int[] height)
{
int maxArea = 0;
int left = 0;
int right = height.Length - 1;
while (left < right)
{
// Calculate the area between the lines at left and right pointers
int width = right - left;
int h = Math.Min(height[left], height[right]);
int area = width * h;
// Update maxArea if the current area is greater
maxArea = Math.Max(maxArea, area);
// Move the pointer with the smaller height inward
if (height[left] < height[right])
{
left++;
}
else
{
right--;
}
}
return maxArea;
}
public static void Main(string[] args)
{
Solution solution = new Solution();
int[] heights1 = { 1, 8, 6, 2, 5, 4, 8, 3, 7 };
Console.WriteLine(solution.MaxArea(heights1));
int[] heights2 = { 4, 3, 2, 1, 5 };
Console.WriteLine(solution.MaxArea(heights2));
int[] heights3 = {5};
Console.WriteLine(solution.MaxArea(heights3));
int[] heights4 = { };
Console.WriteLine(solution.MaxArea(heights4));
}
}
Output:
49 16 0 0
Explanation of the said C# code:
- The "MaxArea()" method takes an array of integers 'height' as input and returns the maximum area of water that can be trapped.
- Inside the method:
- Initialize 'maxArea' to 0, 'left' to 0 (index of the leftmost line), and 'right' to height.Length - 1 (index of the rightmost line).
- Use a while loop to iterate until the 'left' pointer is less than the 'right' pointer.
- Calculate the width of the container between the lines at the 'left' and 'right' pointers.
- Calculate the height of the container, which is the minimum of the heights of the lines at the 'left' and 'right' pointers.
- Calculate the area of the container using the width and height.
- Update 'maxArea' if the current area is greater than the previous maximum.
- Move the pointer with the smaller height inward. If the height at the 'left' pointer is less than the height at the 'right' pointer, increment the 'left' pointer; otherwise, decrement the 'right' pointer.
- The "Main()" method is used to demonstrate the usage of the "MaxArea()" method with different test cases.
JavaScript - Container With Most Water
Code:
function maxArea(height) {
let maxArea = 0;
let left = 0;
let right = height.length - 1;
while (left < right) {
// Calculate the area between the lines at left and right pointers
const width = right - left;
const h = Math.min(height[left], height[right]);
const area = width * h;
// Update maxArea if the current area is greater
maxArea = Math.max(maxArea, area);
// Move the pointer with the smaller height inward
if (height[left] < height[right]) {
left++;
} else {
right--;
}
}
return maxArea;
}
// Test cases
console.log(maxArea([1, 8, 6, 2, 5, 4, 8, 3, 7]));
console.log(maxArea([4, 3, 2, 1, 5]));
console.log(maxArea([]));
console.log(maxArea([5]));
Output:
49 16 0 0
Explanation of the said JavaScript code:
- The "maxArea()" function takes an array 'height' as input and returns the maximum area of water that can be trapped.
- Inside the function:
- Initialize 'maxArea' to 0, 'left' to 0 (index of the leftmost line), and 'right' to height.length - 1 (index of the rightmost line).
- Use a while loop to iterate until the 'left' pointer is less than the 'right' pointer.
- Calculate the width of the container between the lines at the 'left' and 'right' pointers.
- Calculate the height of the container, which is the minimum of the heights of the lines at the 'left' and 'right' pointers.
- Calculate the area of the container using the width and height.
- Update 'maxArea' if the current area is greater than the previous maximum.
- Move the pointer with the smaller height inward. If the height at the 'left' pointer is less than the height at the 'right' pointer, increment the 'left' pointer; otherwise, decrement the 'right' pointer.
Time and Space Complexity:
Time Complexity:
- The code consists of a single while loop that iterates until the 'left' pointer is less than the 'right' pointer.
- Within each iteration of the loop, constant time operations are performed, including comparisons, arithmetic operations, and function calls ('min' and 'max').
- Since the loop runs until 'left' and 'right' pointers meet (or cross each other), and at each step, either the 'left' or 'right' pointer moves closer to each other, the time complexity of the while loop is O(n), where n is the number of elements in the input array 'height'.
- Therefore, the overall time complexity of the "max_area()" function is O(n).
Space Complexity:
- The code uses only a constant amount of extra space, regardless of the size of the input array 'height'.
- It initializes a few variables ('max_area', 'left', and 'right'), which occupy constant space.
- Additionally, it computes a few intermediate variables ('width', 'h', and 'area'), which also occupy constant space.
- There are no additional data structures or recursive calls that would increase the space complexity with respect to the input size.
- Thus, the space complexity of the code is O(1), indicating constant space complexity.
Overall, the provided code has a time complexity of O(n) and a space complexity of O(1).