Product of Array Except Self: Algorithm Explanation
Problem:
Product of Array except Self: Given an array of nums, return an array output such that output[i] is equal to the product of all elements except nums[i].
Introduction to the Problem:
The "Product of Array Except Self" problem involves calculating an output array where each element 'output[i]' is equal to the product of all elements in the input array 'nums' except for the element at index 'i'.
- Input and Output Requirements
- Real-world Scenario
- Underlying Concepts
- Examples and Illustrations
- Brute Force Approach
- Optimization Techniques
- Algorithmic Thinking
- Algorithmic Strategies
- Common Mistakes and Pitfalls
- Coding Best Practices
- Testing and Debugging
- Algorithm Analysis
- Application in Real-world Projects
- Example test cases
- Solution for different languages
- Time and Space Complexity
Input and Output Requirements:
- Input format:
- The input consists of an array 'nums' of integers.
- The array 'nums' can have both positive and negative integers.
- The array length can vary.
- Output format:
- The output should be an array 'output' of the same length as 'nums'.
- Each element 'output[i]' should represent the product of all elements in 'nums' except for the element at index 'i'.
Real-world Scenario:
Suppose we have a manufacturing plant that produces various components for electronic devices. Each component has its own production time, represented by an array of integers. The task is to calculate the production time for each component if it were the only one being produced at a time, excluding itself from the production time of other components.
Components Production Time: [10, 5, 8, 15]
In this scenario,
- We want to calculate the production time for each component individually, assuming it is the only one being produced at a time.
- For example, if we consider the first component (with a production time of 10), the production time for it would be the product of all other components' production times, which is 5*8*15 = 600.
- Similarly, for the second component (with a production time of 5), the production time would be 10*8*15 = 1200, and so on.
This problem helps optimize production scheduling by understanding the impact of producing each component independently on overall production time.
Underlying Concepts:
Multiplicative Thinking:
- Concept: Multiplicative thinking involves understanding and leveraging the properties of multiplication to solve problems efficiently.
- Application: In the context of the "Product of an Array Except Self" problem, multiplicative thinking allows us to approach the problem by considering the cumulative effect of multiplying all elements except the current one. By leveraging this approach, we can avoid repeated multiplications and optimize the overall computation process.
Prefix and suffix products:
- Concept: Prefix and suffix products are intermediate arrays that store the cumulative product of elements from the beginning (prefix) and end (suffix) of the array, respectively.
- Application: Utilizing prefix and suffix products enables us to efficiently calculate the product of array elements except the current element. By precomputing these products, we eliminate redundant calculations and optimize the overall computation process.
Examples and Illustrations:
Walkthrough Examples:
Example 1:
Consider the array [1, 2, 3, 4].
- Start with the first element 1.
- Calculate the product of all other elements except 1: 2*3* 4 = 24.
- The output for the first element is 24.
- Move to the second element 2.
- Calculate the product of all other elements except 2: 1*3*4 = 12.
- The output for the second element is 12.
- Repeat this process for the remaining elements.
Example 2:
Take the array [2, 3, 5, 7].
- Begin with the first element 2.
- Calculate the product of all other elements except 2: 3*5*7 = 105.
- The output for the first element is 105.
- Move to the second element 3.
- Calculate the product of all other elements except 3: 2*5*7 = 70.
- The output for the second element is 70.
- Repeat this process for the remaining elements.
Visualization:
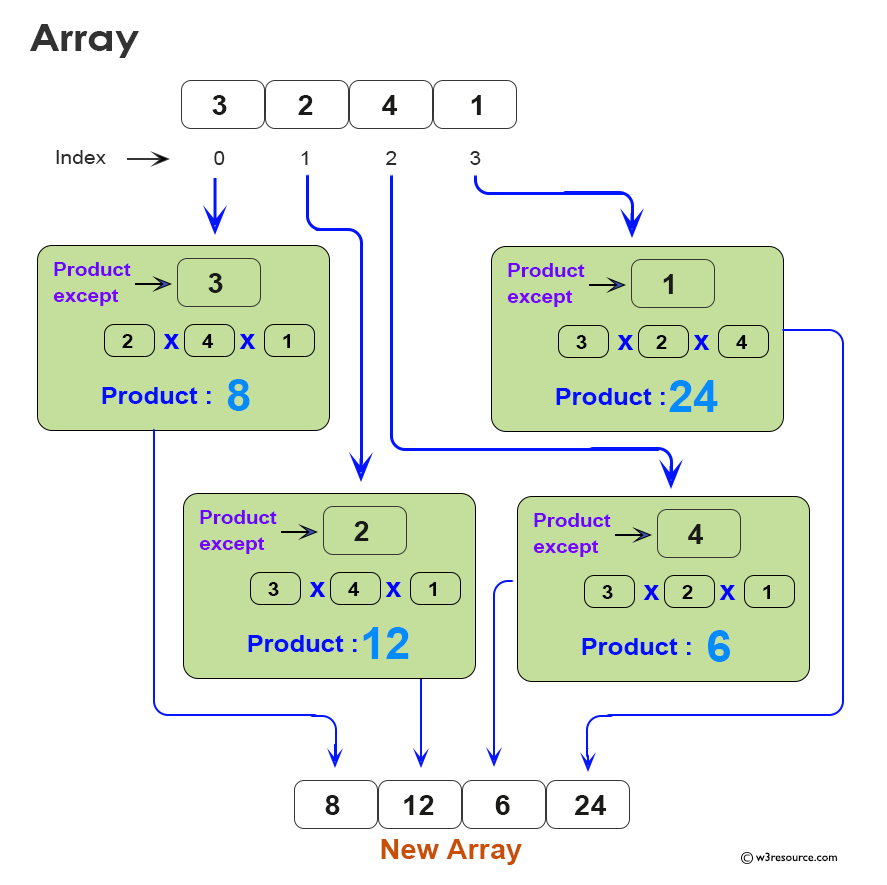
Brute Force Approach:
Naive Solution: The brute force approach for the "Product of Array Except Self" problem involves calculating the product of all elements in the array except the current element. The steps include:
- Iteration:
- Iterate through each element in the array.
- For each element at index 'i', calculate the product of all other elements in the array.
- Product calculation:
- Initialize a variable to store the product of all elements.
- For each element at index 'i', traverse the array again and multiply all elements except the one at index 'i'.
- Output generation:
- Store the calculated product for each element at index 'i' in the output array.
Complexity Analysis:
Let's discuss the time and space complexity of the brute force approach:
- Time complexity:
- In the brute force approach, for each element in the array, we iterate through all other elements to calculate their product.
- This results in a time complexity of O(n^2), where 'n' is the size of the array.
- As we have nested loops traversing the array, the time taken increases quadratically with the size of the input array.
- Space complexity:
- The space complexity of the brute force approach is O(n), where 'n' is the size of the array.
- We use additional space to store the output array, which has the same size as the input array.
- Efficiency:
- While the brute force approach is straightforward and easy to implement, it may not be efficient for large datasets.
- Its quadratic time complexity makes it impractical for arrays with a large number of elements.
- This approach involves redundant calculations, as the product of elements is recomputed for each element in the array.
Optimization Techniques:
Prefix and Suffix Products Approach:
The Prefix and Suffix Products approach is an optimized solution for the "Product of an Array Except Self" problem. It involves utilizing prefix and suffix products to compute the final result efficiently.
Steps:
- Prefix Products:
- Calculate the prefix product of all elements in the array. This represents the product of all elements to the left of each element.
- Suffix Products:
- Calculate the suffix product of all elements in the array. This represents the product of all elements to the right of each element.
- Final output:
- Multiply the corresponding prefix and suffix products to get the final output array. Each element is the product of all elements except the current element.
Complexity analysis:
Let's compare the time and space complexity of the Prefix and Suffix Products approach with the brute force approach.
- Time complexity:
- The time complexity of the Prefix and Suffix Products approach is O(n), where 'n' is the size of the array.
- We perform three passes through the array: one for calculating prefix products, one for calculating suffix products, and one for computing the final output.
- Each pass involves traversing the array linearly, resulting in a linear time complexity.
- Space complexity:
- The space complexity of the Prefix and Suffix Products approach is O(n), where 'n' is the size of the array.
- We use additional space to store the prefix and suffix product arrays, each of size 'n'.
- However, unlike the brute force approach, which requires storing the entire output array, the Prefix and Suffix Products approach only requires storing the prefix and suffix products, reducing space overhead.
Algorithmic Thinking:
Divide and Conquer: Divide and Conquer is a problem-solving technique that involves breaking down a complex problem into simpler subproblems, solving each subproblem independently, and then combining the solutions to obtain the overall solution. In the context of the "Product of an Array Except Self" problem, the technique of dividing and conquering can be applied effectively by using prefix and suffix products.
- Breaking Down the Problem:
- Divide and Conquer involves identifying key subproblems within the problem statement. In the case of "Product of Array Except Self," the main subproblems include calculating the prefix products and suffix products of the array elements.
- By breaking down the problem into these subproblems, the initial complexity becomes more manageable, allowing for a systematic approach to solving the problem.
- Steps for Divide and Conquer:
- Identify key subproblems within the problem statement, such as calculating prefix and suffix products.
- Solve each subproblem independently. In this case, compute the prefix products and suffix products of the array elements separately.
- Combine the subproblem solutions to obtain the overall solution. Multiply the corresponding prefix and suffix products to get the final output array.
- Application to "Product of Array Except Self":
- The Divide and Conquer approach breaks down the problem into subproblems related to calculating prefix and suffix products.
- By solving these subproblems independently and combining the results, an effective solution to the original problem is achieved.
Algorithmic Strategies:
- Brute Force approach:
- Calculate the product of all elements in the array.
- For each element nums[i], divide the total product by nums[i] to get the product of all elements except nums[i].
- Time Complexity: O(n^2) - As it involves nested loops.
- Space Complexity: O(1) - No extra space is required.
- Prefix and suffix products:
- Create two arrays 'prefix' and 'suffix'.
- prefix[i] represents the product of all elements to the left of nums[i].
- suffix[i] represents the product of all elements to the right of nums[i].
- Multiply prefix[i] and suffix[i] to get the product of all elements except nums[i].
- Time Complexity: O(n) - Requires two passes through the array.
- Space Complexity: O(n) - Requires additional space for 'prefix' and 'suffix' arrays.
- Optimized Prefix and Suffix Products:
- Instead of using separate arrays for prefix and suffix products, calculate them on the fly.
- Use a single array to store prefix products and update it incrementally.
- Use a variable to keep track of the product of elements encountered from the right side while traversing the array.
- Time Complexity: O(n) - Single pass through the array.
- Space Complexity: O(1) - No extra space is required apart from the output array.
- Using division:
- Calculate the total product of all elements in the array.
- For each element nums[i], divide the total product by nums[i] to get the product of all elements except nums[i].
- Handle cases where nums[i] is zero separately to avoid division by zero.
- Time Complexity: O(n) - Single pass through the array.
- Space Complexity: O(1) - No extra space is required apart from the output array.
Common Mistakes and Pitfalls:
- Edge Cases:
- Overlooking the empty array:
- Mistake: Assuming the array always has elements.
- Solution: Check for an empty array at the beginning and handle it appropriately to avoid errors.
- Handling Single Element Array:
- Mistake: Not considering the case where the array has only one element.
- Solution: Ensure the algorithm works correctly for arrays with one or two elements.
- Extreme values:
- Mistake: Ignoring edge cases with extreme values (e.g., very large or very small integers).
- Solution: Test the algorithm with extreme values to verify its robustness.
- Zero as an element:
- Missing Zero Element:
- Mistake: Not testing the algorithm with arrays containing zero as an element.
- Solution: Explicitly test the algorithm with arrays containing zero to ensure correct handling.
- Handling duplicate elements:
- Ignore duplicate elements:
- Mistake: Overlooking duplicate elements in the array.
- Solution: Account for duplicate elements and decide whether to include them in the result or not.
- Overflow and Underflow:
- Ignoring integer overflow:
- Mistake: Not considering integer overflow when calculating products.
- Solution: Use data types that handle large products or implement checks to prevent overflow.
- Handling negative numbers:
- Misinterpreting negative numbers:
- Mistake: Misinterpreting how negative numbers affect product calculations.
- Solution: Understand the impact of negative numbers on product calculation and adjust algorithm accordingly.
Coding Best Practices:
- Modularity:
- Emphasize modular code by breaking down the solution into smaller functions or methods, each addressing a specific subtask.
- Encapsulate logic related to specific functionalities within dedicated functions.
- Advantages:
- Improved readability: Each function focuses on a specific task, making the code easier to understand.
- Reusability: Modular functions can be reused in other parts of the code or in different projects.
- Easy debugging: Isolate and troubleshoot issues in specific modules without affecting the entire codebase.
- Example (Python code):
def product_except_self(nums):
# Function to calculate product of array except self
# ...
def main():
# Main function to orchestrate the program
# ...
if __name__ == "__main__":
main()
- Variable Naming:
- Use meaningful and descriptive variable names that convey the purpose or content of the variable.
- Avoid single-letter variable names unless they represent common conventions (e.g., loop indices).
- Benefits:
- Readability: Clear and descriptive names enhance code readability for yourself and others.
- Maintenance: Easy maintenance as the purpose of variables is evident.
- Reduced comments: Well-named variables can reduce the need for excessive comments.
- Consistency:
- Maintain a consistent coding style throughout the codebase.
- Follow established conventions for a uniform appearance.
- Consistency facilitates version control and collaboration, especially in team environments.
- Adopting a consistent style ensures that team members can easily understand and contribute to the code.
Testing and Debugging:
Test cases:
- Test Case Selection:
- Test the solution with a variety of input scenarios to ensure its correctness.
- Include both typical cases and edge cases to cover a wide range of possibilities.
- Example Test Cases for "Product of Array Except Self":
- Positive cases with valid outputs where the product of all elements except the current one equals the expected output.
- Negative cases with no valid output, such as an empty array or an array with only one element.
- Cases with extreme values, including large or small integers, to verify the robustness of the solution.
- Test Case Execution:
- Automate the execution of test cases to streamline the testing process.
- Utilize testing frameworks or write custom test scripts to cover various scenarios effectively.
Debugging techniques:
Debugging is the process of identifying and fixing errors or bugs in your code. Here are some common debugging techniques:
- Print Statements: Inserting print statements strategically in your code can help output variable values and intermediate results. This allows you to track the flow of execution and identify where the code deviates from the expected behavior.
- Using a Debugger: Integrated development environments (IDEs) often come with debuggers that allow you to set breakpoints, step through the code, and inspect variable values. This interactive approach helps pinpoint issues more effectively.
- Code Review: Engaging in code reviews with peers or mentors can provide valuable insights. External perspectives may reveal overlooked errors or suggest alternative approaches to problem-solving.
- Rubber Duck Debugging: Sometimes, explaining your code step by step to an inanimate object (or a colleague) can help uncover logical errors. The act of verbalizing the code can lead to insights into the problem.
- Isolating Sections: Temporarily commenting out or isolating specific sections of code can help identify the source of errors. By narrowing down the scope of investigation to smaller segments, you can focus on debugging more efficiently.
- Check Variable Values: Ensure that variables hold expected values at different stages of execution. Pay attention to changes in variable states, as unexpected modifications can indicate potential issues.
- Use Exception Handling: Implementing exception handling allows you to catch and log errors, preventing the program from crashing abruptly. Logging error messages provides valuable information for troubleshooting.
- Reproduce the Issue: Attempt to reproduce the issue in a controlled environment. By identifying the steps leading to the error and examining the relevant code, you can gain insights into the root cause of the problem.
- Logging: Incorporate logging statements in your code to record the program's state during execution. Log messages can provide valuable insights into the program flow and variable values, aiding in debugging.
- Test Incrementally: Implement and test your solution incrementally, focusing on small sections at a time. This approach helps detect issues early in the development process and facilitates troubleshooting.
- Pair Programming: Collaborating with a partner in a pair programming session can be an effective debugging technique. Two sets of eyes can catch errors more efficiently, leading to faster problem resolution.
Algorithm Analysis:
- Time and Space Complexity:
- Time Complexity Analysis: Analyzing the time complexity of an algorithm involves evaluating how its runtime grows with respect to the input size. It often involves identifying the dominant operations and understanding their relationship with the input size.
- Explanation: To analyze time complexity, we typically count the number of basic operations performed by the algorithm as a function of the input size. This helps us understand how the algorithm's performance scales with larger inputs.
- Space Complexity Analysis: Space complexity analysis focuses on understanding how much additional memory an algorithm requires relative to the input size. It involves considering the space used by variables, data structures, and recursive function calls.
- Explanation: Space complexity analysis helps us understand the memory requirements of an algorithm. We evaluate how the space usage grows with increasing input size and identify any additional data structures or resources used.
- Trade-offs:
- Trade-offs Between Time and Space Complexity: When designing algorithms, there is often a trade-off between optimizing for time or space complexity. Improving one aspect may come at the expense of the other.
- Explanation: For example, algorithms that use additional data structures, such as hash tables or trees, to optimize for time complexity may have higher space complexity. Conversely, algorithms optimized for space efficiency may sacrifice speed.
Application in Real-world Projects:
- Financial Transactions:
- In financial systems, algorithms for array manipulation, such as those used in "Product of Array Except Self," can be applied to detect anomalies or patterns in transaction data. For example, if we have an array representing transaction amounts, we can use the product of array elements except self to identify unusual transaction patterns. An abnormally high or low product may indicate potentially fraudulent transactions.
- Stock market analysis:
- When analyzing historical stock prices, the problem of "Product of Array Except Self" can be utilized to identify trends or patterns. For instance, if we have an array representing daily stock prices, calculating the product of array elements except self can help identify days where the product is significantly different from others. This could indicate days with unusual stock price movements or specific trading patterns.
- Network Security:
- In network security, algorithms for array manipulation like "Product of Array Except Self" can aid in detecting anomalies or suspicious patterns in network traffic. For example, if we have an array representing network traffic data, calculating the product of array elements except self could help identify periods of unusually high or low network activity. This information could be used to detect potential security threats or abnormalities in network behavior.
- Supply chain optimization:
- In supply chain management, the problem of "Product of Array Except Self" can be applied to optimize inventory planning and management. For instance, if we have an array representing product demand, calculating the product of array elements except self can help identify products with complementary demand patterns. This information can be used to optimize inventory levels and ensure efficient supply chain operations.
- Social Network Recommendations:
- Social network platforms can utilize algorithms similar to "Product of Array Except Self" to make personalized recommendations to users. For example, if we have an array representing user preferences or interactions, calculating the product of array elements except self can help identify users with similar interests or behaviors. This information can be used to make targeted recommendations for connections, groups, or content tailored to each user's preferences.
Example test cases:
- Positive cases with valid arrays:
- Input: nums = [1, 2, 3, 4] Expected output: [24, 12, 8, 6] Explanation: For index 0, the product of all elements except nums[0] is 24 (234), for index 1, it's 12 (134), for index 2, it's 8 (124), and for index 3, it's 6 (123).
- Input: nums = [5, 2, 1, 3] Expected output: [6, 15, 30, 10] Explanation: For index 0, the product of all elements except nums[0] is 6 (2135), for index 1, it's 15 (5135), for index 2, it's 30 (5235), and for index 3, it's 10 (5215).
- Negative cases with empty or invalid arrays:
- Input: nums = [] Expected output: [] Explanation: Since the input array is empty, there are no elements to calculate the product, resulting in an empty array.
- Input: nums = [0, 0, 0, 0] Expected output: [0, 0, 0, 0] Explanation: In this case, every element in the output array should be 0, as the product of any element except itself is 0 when there are multiple zeros in the input.
- Edge cases with single-element arrays:
- Input: nums = [10] Expected output: [1] Explanation: There's only one element in the array, so the output should contain only 1 as the product of all elements except itself.
- Input: nums = [0] Expected output: [0] Explanation: Similar to the previous case, the output should contain only 0 as the product of all elements except itself.
- Cases with negative numbers and large values:
- Input: nums = [-1, 2, -3, 4] Expected output: [-24, 12, -8, 6] Explanation: This test case includes negative numbers. For example, for index 1, the product is 12 (-1 -3 4), and for index 2, it's -8 (-1 2 4).
- Input: nums = [1000, 2000, 3000, 4000] Expected output: [24000000000, 12000000000, 8000000000, 6000000000] Explanation: This test case includes large values. Each element in the output array is the product of all other elements in the input array except itself.
Solution for different languages:
Python - Product of Array except Self
Code:
def productExceptSelf(nums):
# Initialize two arrays to store products of elements to the left and right of each element
left_products = [1] * len(nums)
right_products = [1] * len(nums)
result = [0] * len(nums)
# Calculate products of elements to the left of each element
for i in range(1, len(nums)):
left_products[i] = left_products[i - 1] * nums[i - 1]
# Calculate products of elements to the right of each element
for i in range(len(nums) - 2, -1, -1):
right_products[i] = right_products[i + 1] * nums[i + 1]
# Multiply corresponding elements from left and right products arrays to get the result
for i in range(len(nums)):
result[i] = left_products[i] * right_products[i]
return result
# Test cases
test_cases = [
[1, 2, 3, 4], # Expected output: [24, 12, 8, 6]
[4, 3, 2, 1], # Expected output: [6, 8, 12, 24]
[1, 1, 1, 1], # Expected output: [1, 1, 1, 1]
]
# Run test cases
for nums in test_cases:
print(productExceptSelf(nums))
Output:
[24, 12, 8, 6] [6, 8, 12, 24] [1, 1, 1, 1]
Explanation of the said Python code:
- Initialization:
- 'left_products' and 'right_products' are initialized as arrays of length 'len(nums)' filled with 1, indicating that each element initially represents the product of all elements to its left and right, respectively.
- 'result' is initialized as an array of length 'len(nums)' filled with 0, to be filled with the final product values.
- Calculating the products to the left:
- A loop iterates through the range from 1 to len(nums) - 1.
- At each index i, left_products[i] is calculated by multiplying left_products[i - 1] with nums[i - 1]. This represents the product of all elements to the left of nums[i].
- Calculating products to the right:
- Another loop iterates through the range from len(nums) - 2 down to 0.
- At each index i, right_products[i] is calculated by multiplying right_products[i + 1] with nums[i + 1]. This represents the product of all elements to the right of nums[i].
- Calculating the final result:
- Finally, a loop iterates through each index of nums.
- At each index i, result[i] is calculated by multiplying left_products[i] with right_products[i]. This represents the product of all elements except nums[i].
Java - Product of Array except Self
Code:
import java.util.Arrays;
class ProductExceptSelf {
public static int[] productExceptSelf(int[] nums) {
// Initialize arrays to store products of elements to the left and right of each element
int[] leftProducts = new int[nums.length];
int[] rightProducts = new int[nums.length];
int[] result = new int[nums.length];
// Calculate products of elements to the left of each element
leftProducts[0] = 1;
for (int i = 1; i < nums.length; i++) {
leftProducts[i] = leftProducts[i - 1] * nums[i - 1];
}
// Calculate products of elements to the right of each element
rightProducts[nums.length - 1] = 1;
for (int i = nums.length - 2; i >= 0; i--) {
rightProducts[i] = rightProducts[i + 1] * nums[i + 1];
}
// Multiply corresponding elements from left and right products arrays to get the result
for (int i = 0; i < nums.length; i++) {
result[i] = leftProducts[i] * rightProducts[i];
}
return result;
}
public static void main(String[] args) {
// Test cases
int[][] testCases = {
{1, 2, 3, 4}, // Expected output: [24, 12, 8, 6]
{4, 3, 2, 1}, // Expected output: [6, 8, 12, 24]
{1, 1, 1, 1} // Expected output: [1, 1, 1, 1]
};
// Run test cases
for (int[] nums : testCases) {
System.out.println(Arrays.toString(productExceptSelf(nums)));
}
}
}
Output:
[24, 12, 8, 6] [6, 8, 12, 24] [1, 1, 1, 1]
Explanation of the said Java code:
- Initialization:
- Three arrays are initialized: 'leftProducts', 'rightProducts', and 'result', each with the length of the input array 'nums'. These arrays will store the products of elements to the left, right, and the final result, respectively.
- Calculating Products to the Left:
- The first loop initializes the 'leftProducts' array.
- It starts from index 1 and calculates each element by multiplying the previous element in 'leftProducts' with the corresponding element in nums. The first element is set to 1, as there are no elements to the left of it.
- Calculating Products to the Right:
- The second loop initializes the 'rightProducts' array.
- It starts from the second-to-last index (nums.length - 2) and goes backward to index 0.
- Similar to the first loop, it calculates each element by multiplying the next element in 'rightProducts' with the corresponding element in 'nums'. The last element is set to 1, as there are no elements to its right.
- Calculating Final Result:
- The third loop calculates the final result.
- It multiplies the corresponding elements from the 'leftProducts' and 'rightProducts' arrays and assigns the result to the 'result' array.
C++ - Product of Array except Self
Code:
#include <iostream>
#include <vector>
using namespace std;
vector<int> productExceptSelf(vector<int>& nums) {
// Initialize vectors to store products of elements to the left and right of each element
vector<int> leftProducts(nums.size(), 1);
vector<int> rightProducts(nums.size(), 1);
vector<int> result(nums.size());
// Calculate products of elements to the left of each element
for (int i = 1; i < nums.size(); i++) {
leftProducts[i] = leftProducts[i - 1] * nums[i - 1];
}
// Calculate products of elements to the right of each element
for (int i = nums.size() - 2; i >= 0; i--) {
rightProducts[i] = rightProducts[i + 1] * nums[i + 1];
}
// Multiply corresponding elements from left and right products vectors to get the result
for (int i = 0; i < nums.size(); i++) {
result[i] = leftProducts[i] * rightProducts[i];
}
return result;
}
int main() {
// Test cases
vector<vector<int>> testCases = {
{1, 2, 3, 4}, // Expected output: [24, 12, 8, 6]
{4, 3, 2, 1}, // Expected output: [6, 8, 12, 24]
{1, 1, 1, 1} // Expected output: [1, 1, 1, 1]
};
// Run test cases
for (auto& nums : testCases) {
vector<int> result = productExceptSelf(nums);
for (int num : result) {
cout << num << " ";
}
cout << endl;
}
return 0;
}
Output:
24 12 8 6 6 8 12 24 1 1 1 1
Explanation of the said C++ code:
- Header Files:
- #include <iostream> and #include <vector>: These are standard C++ library headers for input-output operations and vector data structure respectively.
- Namespace:
- using namespace std;: This line allows you to use names from the "std" namespace without prefixing them with std::.
- Function productExceptSelf:
- It takes a reference to a vector of integers 'nums' as input and returns a vector of integers.
- Three vectors are initialized: 'leftProducts', 'rightProducts', and 'result', each with the size of the input vector 'nums'. These vectors will store the products of elements to the left, to the right, and the final result, respectively.
- Calculating Products to the Left:
- The first loop initializes the 'leftProducts' vector.
- It starts from index 1 and calculates each element by multiplying the previous element in leftProducts with the corresponding element in nums. The first element is set to 1, as there are no elements to the left of it.
- Calculating Products to the Right:
- The second loop initializes the 'rightProducts' vector.
- It starts from the second-to-last index (nums.size() - 2) and goes backward to index 0.
- Similar to the first loop, it calculates each element by multiplying the next element in 'rightProducts' with the corresponding element in 'nums'. The last element is set to 1, as there are no elements to the right of it.
- Calculating Final Result:
- The third loop calculates the final result.
- It multiplies the corresponding elements from 'leftProducts' and 'rightProducts' vectors and assigns the result to the 'result' vector.
- Main Function:
- The "main()" function initializes test cases as a vector of vectors, where each inner vector represents a test case input.
- It then runs each test case through the "productExceptSelf()" function and prints the result.
C# - Product of Array except Self
Code:
using System;
class ProductExceptSelf
{
public static int[] CalculateProductExceptSelf(int[] nums)
{
// Initialize arrays to store products of elements to the left and right of each element
int[] leftProducts = new int[nums.Length];
int[] rightProducts = new int[nums.Length];
int[] result = new int[nums.Length];
// Calculate products of elements to the left of each element
leftProducts[0] = 1;
for (int i = 1; i < nums.Length; i++)
{
leftProducts[i] = leftProducts[i - 1] * nums[i - 1];
}
// Calculate products of elements to the right of each element
rightProducts[nums.Length - 1] = 1;
for (int i = nums.Length - 2; i >= 0; i--)
{
rightProducts[i] = rightProducts[i + 1] * nums[i + 1];
}
// Multiply corresponding elements from left and right products arrays to get the result
for (int i = 0; i < nums.Length; i++)
{
result[i] = leftProducts[i] * rightProducts[i];
}
return result;
}
static void Main(string[] args)
{
// Test cases
int[][] testCases = {
new int[] {1, 2, 3, 4}, // Expected output: [24, 12, 8, 6]
new int[] {4, 3, 2, 1}, // Expected output: [6, 8, 12, 24]
new int[] {1, 1, 1, 1} // Expected output: [1, 1, 1, 1]
};
// Run test cases
foreach (int[] nums in testCases)
{
Console.WriteLine(string.Join(" ", CalculateProductExceptSelf(nums)));
}
}
}
Output:
24 12 8 6 6 8 12 24 1 1 1 1
Explanation of the said C# code:
- CalculateProductExceptSelf Method:
- This method takes an integer array nums as input and returns another integer array representing the product of all elements except itself.
- It initializes three arrays: 'leftProducts', 'rightProducts', and 'result', each with the same length as 'nums'.
- It calculates the product of elements to the left of each element and stores them in the 'leftProducts' array.
- It calculates the product of elements to the right of each element and stores them in the 'rightProducts' array.
- Finally, it multiplies the corresponding elements from the 'leftProducts' and 'rightProducts' arrays to get the result.
- Main Method:
- This method contains test cases represented as an array of integer arrays (testCases).
- It iterates over each test case, calculates the product except self using the "CalculateProductExceptSelf()" method, and prints the result to the console.
JavaScript - Product of Array except Self
Code:
function productExceptSelf(nums) {
// Initialize arrays to store products of elements to the left and right of each element
const leftProducts = new Array(nums.length).fill(1);
const rightProducts = new Array(nums.length).fill(1);
const result = new Array(nums.length);
// Calculate products of elements to the left of each element
for (let i = 1; i < nums.length; i++) {
leftProducts[i] = leftProducts[i - 1] * nums[i - 1];
}
// Calculate products of elements to the right of each element
for (let i = nums.length - 2; i >= 0; i--) {
rightProducts[i] = rightProducts[i + 1] * nums[i + 1];
}
// Multiply corresponding elements from left and right products arrays to get the result
for (let i = 0; i < nums.length; i++) {
result[i] = leftProducts[i] * rightProducts[i];
}
return result;
}
// Test cases
const testCases = [
[1, 2, 3, 4], // Expected output: [24, 12, 8, 6]
[4, 3, 2, 1], // Expected output: [6, 8, 12, 24]
[1, 1, 1, 1] // Expected output: [1, 1, 1, 1]
];
// Run test cases
testCases.forEach(nums => {
console.log(productExceptSelf(nums));
});
Output:
[24, 12, 8, 6] [6, 8, 12, 24] [1, 1, 1, 1]
Explanation of the said JavaScript code
- productExceptSelf Function:
- This function takes an array 'nums' as input and returns another array representing the product of all elements except itself.
- It initializes three arrays: 'leftProducts', 'rightProducts', and 'result', each with the same length as nums, and fills them with initial values of 1.
- It calculates the product of elements to the left of each element and stores them in the 'leftProducts' array.
- It calculates the product of elements to the right of each element and stores them in the 'rightProducts' array.
- Finally, it multiplies the corresponding elements from the 'leftProducts' and 'rightProducts' arrays to get the final result.
Time and Space Complexity:
Time Complexity:
The time complexity of the given code is (π)O(n), where πn is the number of elements in the input array 'nums'.
- The first loop iterates through the array once to calculate the products of elements to the left of each element. This loop runs in (π)O(n) time.
- Similarly, the second loop iterates through the array once to calculate the products of elements to the right of each element. This loop also runs in (π)O(n) time.
- The third loop iterates through the array once to multiply corresponding elements from the 'leftProducts' and 'rightProducts' arrays to get the final result. This loop runs in (π)O(n) time.
Since all three loops iterate through the array independently, the overall time complexity is π(π)+π(π)+π(n)=π(π)O(n)+O(n)+O(n)=O(n).
Space Complexity:
The space complexity of the given code is (π)O(n), where πn is the number of elements in the input array 'nums'.
- Three arrays are created: 'leftProducts', 'rightProducts', and 'result', each with a size equal to the length of the input array 'nums'. Therefore, the space complexity for these arrays is π(π)+π(π)+π(π)=π(π)O(n)+O(n)+O(n)=O(n).
- Apart from these arrays, no additional space is used that scales with the input size.
Therefore, the overall space complexity is O(n).
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics