Java: Replace every element with the next greatest element in a given array of integers
Java Array: Exercise-53 with Solution
Write a Java program to replace every element with the next greatest element (from the right side) in a given array of integers.
There is no element next to the last element, therefore replace it with -1.
Sample Solution:
Java Code:
// Import the necessary Java input/output and utility classes.
import java.io.*;
import java.util.Arrays;
// Define the Main class.
public class Main {
public static void main(String[] args)
{
// Create an array of integers.
int nums[] = {45, 20, 100, 23, -5, 2, -6};
int result[];
// Print the original array.
System.out.println("Original Array ");
System.out.println(Arrays.toString(nums));
// Call the next_greatest_num method to modify the array.
result = next_greatest_num(nums);
// Print the modified array.
System.out.println("The modified array:");
System.out.println(Arrays.toString(result));
}
// A method to modify the array by replacing each element with the next greatest number.
static int[] next_greatest_num(int arr_nums[])
{
// Get the size of the array.
int size = arr_nums.length;
// Initialize the maximum element from the right side.
int max_from_right_num = arr_nums[size - 1];
// Set the last element of the array to -1.
arr_nums[size - 1] = -1;
// Iterate through the array from right to left.
for (int i = size - 2; i >= 0; i--)
{
int temp = arr_nums[i];
// Replace the current element with the maximum from the right side.
arr_nums[i] = max_from_right_num;
// Update the maximum from the right side if needed.
if (max_from_right_num < temp)
max_from_right_num = temp;
}
// Return the modified array.
return arr_nums;
}
}
Sample Output:
Original Array [45, 20, 100, 23, -5, 2, -6] The modified array: [100, 100, 23, 2, 2, -6, -1]
Flowchart:
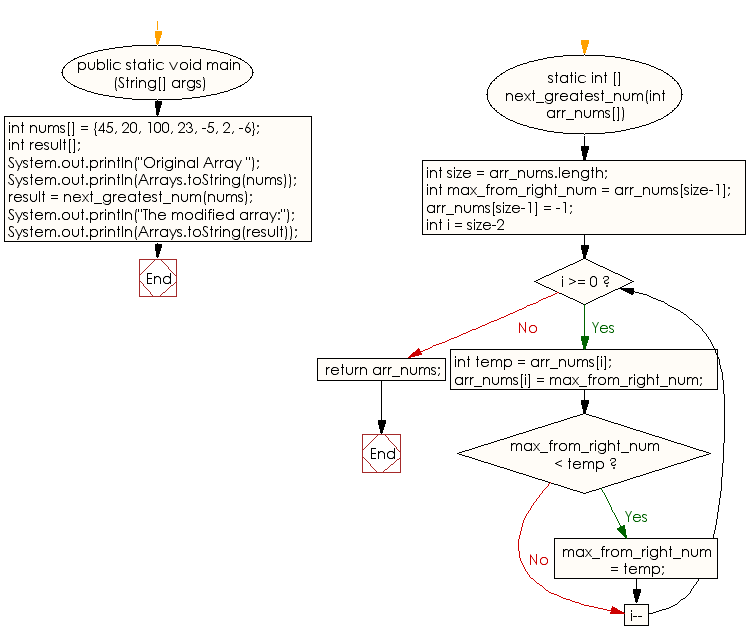
Java Code Editor:
Previous: Write a Java program to separate even and odd numbers of a given array of integers. Put all even numbers first, and then odd numbers.
Next: Write a Java program to check if a given array contains a subarray with 0 sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics