Java: Find all of the longest word in a given dictionary
Java Basic: Exercise-138 with Solution
Write a Java program to find the longest words in a dictionary.
Example-1:
{
"cat",
"flag",
"green",
"country",
"w3resource"
}
Result: "w3resource"
Example-1:
{
"cat",
"dog",
"red",
"is",
"am"
}
Result: "cat", "dog", "red"
Pictorial Presentation:
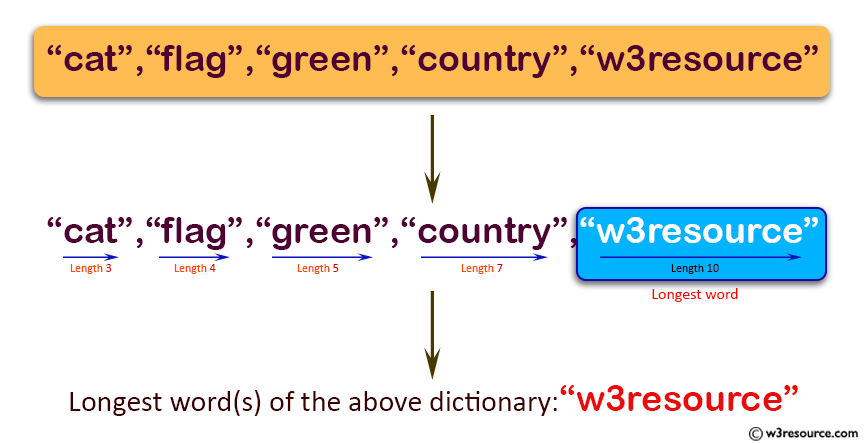
Sample Solution:
Java Code:
import java.util.*;
public class Solution {
// Function to find and return the longest words in the given dictionary
static ArrayList longestWords(String[] dictionary) {
ArrayList list = new ArrayList();
int longest_length = 0;
// Iterate through each word in the dictionary
for (String str : dictionary) {
int length = str.length();
// Check if the current word is longer than the previously found longest word(s)
if (length > longest_length) {
longest_length = length;
list.clear(); // Clear the list as a new longest word is found
}
// If the current word has the same length as the longest word(s), add it to the list
if (length == longest_length) {
list.add(str);
}
}
return list; // Return the list of longest words
}
public static void main(String[] args) {
// Sample dictionary containing words
// String[] dict = {"cat", "flag", "green", "country", "w3resource"};
String[] dict = {"cat", "dog", "red", "is", "am"};
// Print the original dictionary and the longest word(s)
System.out.println("Original dictionary: " + Arrays.toString(dict));
System.out.println("Longest word(s) of the above dictionary: " + longestWords(dict));
}
}
Sample Output:
Original dictionary : [cat, dog, red, is, am] Longest word(s) of the above dictionary: [cat, dog, red]
Flowchart:
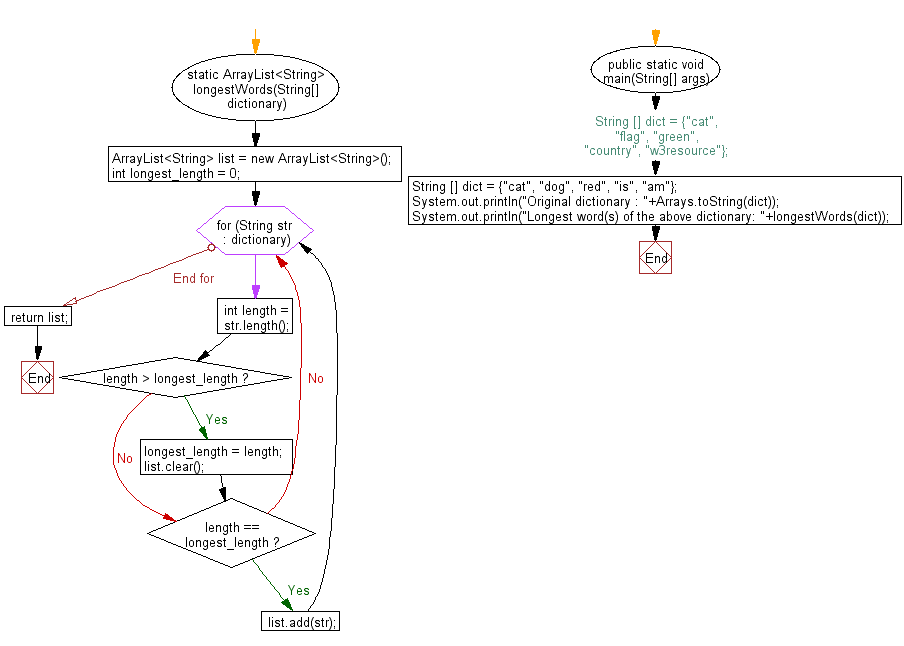
Java Code Editor:
Previous: Write a Java program to find possible unique paths from top-left corner to bottom-right corner of a given grid (m x n).
Next: Write a Java program to get the index of the first number and the last number of a subarray where the sum of numbers is zero from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics