Java: Accepts four integer from the user and prints equal
Java Basic: Exercise-152 with Solution
Write a Java program that accepts four integers from the user and prints equal if all four are equal, and not equal otherwise.
Visual Presentation:
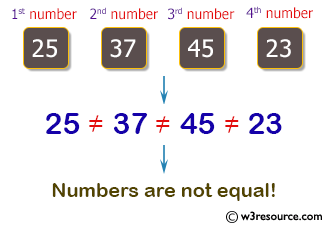
Sample Solution:
Java Code:
import java.util.Scanner;
public class Solution {
public static void main(String[] args) {
// Creating a Scanner object to take input from the user
Scanner in = new Scanner(System.in);
// Prompting the user to input the first number
System.out.print("Input first number: ");
int num1 = in.nextInt();
// Prompting the user to input the second number
System.out.print("Input second number: ");
int num2 = in.nextInt();
// Prompting the user to input the third number
System.out.print("Input third number: ");
int num3 = in.nextInt();
// Prompting the user to input the fourth number
System.out.print("Input fourth number: ");
int num4 = in.nextInt();
// Checking if all four numbers are equal
if (num1 == num2 && num2 == num3 && num3 == num4) {
// Printing a message if all numbers are equal
System.out.println("Numbers are equal.");
} else {
// Printing a message if numbers are not all equal
System.out.println("Numbers are not equal!");
}
}
}
Sample Output:
Input first number: 25 Input second number: 37 Input third number: 45 Input fourth number: 23 Numbers are not equal!
Flowchart:
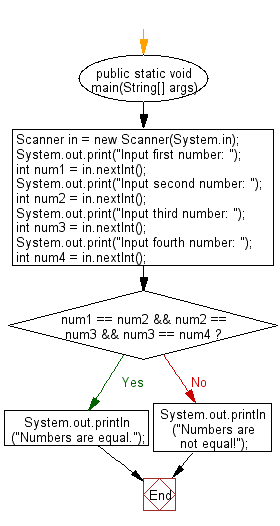
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to find the value of specified expression.
Next: Write a Java program that accepts two double variables and test if both strictly between 0 and 1 and false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics