Java: Count the number of set bits in a 32-bit integer
Java Basic: Exercise-249 with Solution
From Wikipedia,
The Hamming weight of a string is the number of symbols that are different from the zero-symbol of the alphabet used. It is thus equivalent to the Hamming distance from the all-zero string of the same length. For the most typical case, a string of bits, this is the number of 1's in the string, or the digit sum of the binary representation of a given number and the ℓ₁ norm of a bit vector. In this binary case, it is also called the population count, popcount, sideways sum, or bit summation.
Example:
String | Hamming weight |
---|---|
11101 | 4 |
11101000 | 4 |
00000000 | 0 |
789012340567 | 10 |
Sample Solution:
Java Code:
// Importing the Scanner class from the java.util package to read user input
import java.util.Scanner;
// Defining a class named "solution"
public class solution {
// Method to count the number of set bits (1s) in the binary representation of a number
static int count_Set_Bits(int num) {
int ctr = 0;
// Looping until the number becomes zero
while (num != 0) {
// Using bitwise AND operation to clear the rightmost set bit and incrementing the counter
num = num & (num - 1);
ctr++;
}
// Returning the count of set bits
return ctr;
}
// Main method, the entry point of the program
public static void main(String args[]) {
// Creating a Scanner object for user input
Scanner sc = new Scanner(System.in);
// Prompting the user to input a number
System.out.print("Input a number: ");
// Reading the input number from the user
int num = sc.nextInt();
// Calling the count_Set_Bits method and printing the result
System.out.println(count_Set_Bits(num));
// Closing the Scanner to avoid resource leaks
sc.close();
}
}
Sample Output:
Input a number: 1427 6
Flowchart:
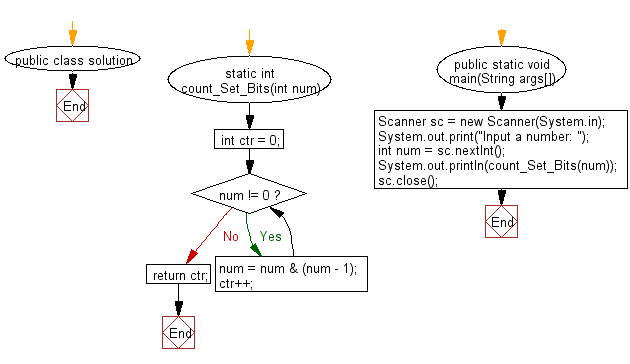
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to check if each letter of a given word is less than the one before it.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics