Java: Searchable interface for document and Web page searching
Java Interface: Exercise-9 with Solution
Write a Java program to create an interface Searchable with a method search(String keyword) that searches for a given keyword in a text document. Create two classes Document and WebPage that implement the Searchable interface and provide their own implementations of the search() method.
Sample Solution:
Java Code:
// Searchable.java
// Declare the Searchable interface
interface Searchable {
// Declare the abstract method "search" that classes implementing this interface must provide
boolean search(String keyword);
}
// Document.java
// Declare the Document class, which implements the Searchable interface
class Document implements Searchable {
// Declare a private instance variable to store the content of the document
private String content;
// Constructor for initializing the document's content
public Document(String content) {
this.content = content;
}
// Implement the "search" method required by the Searchable interface
public boolean search(String keyword) {
// Check if the content contains the specified keyword
return content.contains(keyword);
}
}
// WebPage.java
// Declare the WebPage class, which implements the Searchable interface
class WebPage implements Searchable {
// Declare private instance variables to store the URL and HTML content
private String url;
private String htmlContent;
// Constructor for initializing the URL and HTML content
public WebPage(String url, String htmlContent) {
this.url = url;
this.htmlContent = htmlContent;
}
// Implement the "search" method required by the Searchable interface
public boolean search(String keyword) {
// Check if the HTML content contains the specified keyword
return htmlContent.contains(keyword);
}
}
// Main.java
// Declare the Main class
public class Main {
public static void main(String[] args) {
// Create an instance of the Document class with a sample content
Document document = new Document("This is a sample document.");
// Search for a keyword in the document and store the result
boolean documentContainsKeyword = document.search("sample");
// Print whether the document contains the keyword
System.out.println("Document contains keyword 'sample': " + documentContainsKeyword);
// Create an instance of the WebPage class with a sample URL and HTML content
WebPage webPage = new WebPage("https://www.w3resource.com", "This is a sample webpage.");
// Search for a keyword in the webpage and store the result
boolean webPageContainsKeyword = webPage.search("webpage");
// Print whether the webpage contains the keyword
System.out.println("Webpage contains keyword 'webpage': " + webPageContainsKeyword);
}
}
Sample Output:
Document contains keyword 'sample': true Webpage contains keyword 'webpage': true
Explanation:
In the above exercise –
- The "Document" class and "WebPage" class both implement the Searchable interface and provide their own implementations of the search() method. The "Document" class searches for the keyword in its content, and the WebPage class searches for the keyword in its HTML content.
- In the main() method, we create an instance of the "Document" class and an instance of the WebPage class. We call the search() method on each instance, passing in a keyword, and storing the result in a boolean variable. Finally, we print the result to indicate whether the keyword was found in the document or web page.
Flowchart of Searchable Java:
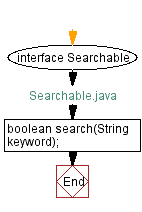
Flowchart of Document Java:
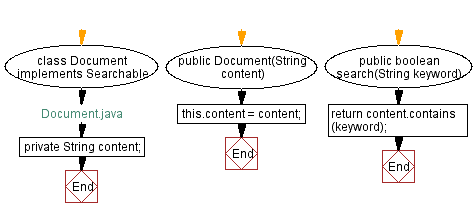
Flowchart of WebPage Java:
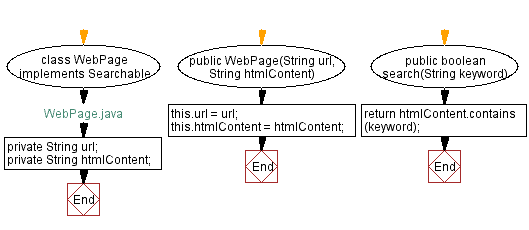
Flowchart of Main Java:
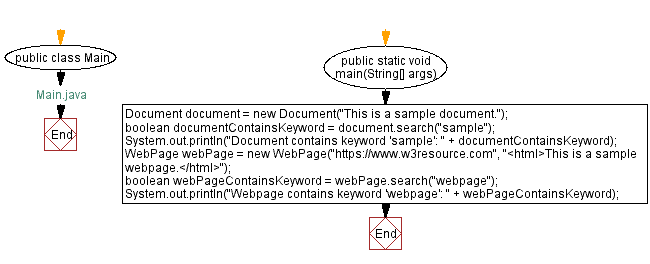
Java Code Editor:
Previous: A Playable Interface with Football, Volleyball, and Basketball implementations implementations.
Next: Encryptable interface for AES and RSA encryption.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics