BorderPane Layout: JavaFX application example
JavaFx Layout Management: Exercise-3 with Solution
Write a JavaFX application that utilizes a BorderPane to arrange a 'label' at the top, a 'button' in the center, and a 'list view' at the bottom.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.ListView;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("BorderPane Example");
// Create a BorderPane layout
BorderPane borderPane = new BorderPane();
// Create a label at the top
Label topLabel = new Label("Top Label");
borderPane.setTop(topLabel);
// Create a button in the center
Button centerButton = new Button("Center Button");
borderPane.setCenter(centerButton);
// Create a ListView at the bottom
ListView listView = new ListView<>();
listView.getItems().addAll("Item 1", "Item 2", "Item 3", "Item 4");
borderPane.setBottom(listView);
// Create the scene and set it in the stage
Scene scene = new Scene(borderPane, 400, 300); // Width and height of the window
primaryStage.setScene(scene);
// Show the window
primaryStage.show();
}
}
In the above exercise, we create a 'BorderPane' layout and add a label to the top, a 'button' to the center, and a 'ListView' to the bottom. The 'setTop', 'setCenter', and 'setBottom' methods of the 'BorderPane' are used to position the components. You can run this application to see the layout in action.
Sample Output:
Flowchart:
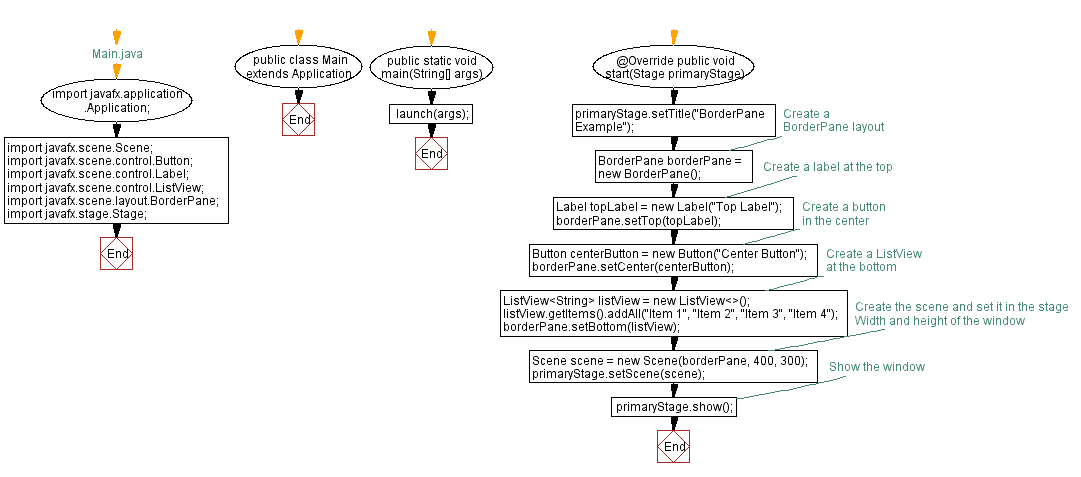
Java Code Editor:
Previous: Horizontal Images: JavaFX application example.
Next: JavaFX GridPane: Simple form creation example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/javafx/javafx-layout-management-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics