Java Recursive Method: Sum of odd numbers in an array
Java Recursive: Exercise-9 with Solution
Write a Java recursive method to find the sum of all odd numbers in an array.
Sample Solution:
Java Code:
public class OddNumberSumCalculator {
public static int calculateOddNumberSum(int[] arr) {
return calculateOddNumberSum(arr, 0);
}
private static int calculateOddNumberSum(int[] arr, int index) {
// Base case: if the index reaches the end of the array, return 0
if (index == arr.length) {
return 0;
}
// Recursive case: check if the element at the current index is odd,
// and recursively call the method with the next index and add the current element if it is odd
int sum = calculateOddNumberSum(arr, index + 1);
if (arr[index] % 2 != 0) {
sum += arr[index];
}
return sum;
}
public static void main(String[] args) {
int[] numbers = {
1,
2,
3,
4,
5,
6,
7,
8,
9
};
int sum = calculateOddNumberSum(numbers);
System.out.println("The sum of all odd numbers in the array is: " + sum);
}
}
Sample Output:
The sum of all odd numbers in the array is: 25
Explanation:
In the above exercises -
First, we define a class OddNumberSumCalculator that includes a recursive method calculateOddNumberSum() to find the sum of all odd numbers in an array.
The calculateOddNumberSum() method has two cases:
- Base case: If the index reaches the end of the array (index == arr.length), we return 0 as there are no more elements to check.
- Recursive case: For any index that is within the bounds of the array, we check if the element at that index is odd. We then recursively call the method with the next index and add the current element to the sum if it is odd. This process continues until we reach the end of the array.
In the main() method, we demonstrate the calculateOddNumberSum() method by finding the sum of all odd numbers in the array [1, 2, 3, 4, 5, 6, 7, 8, 9] and printing the result.
Flowchart:
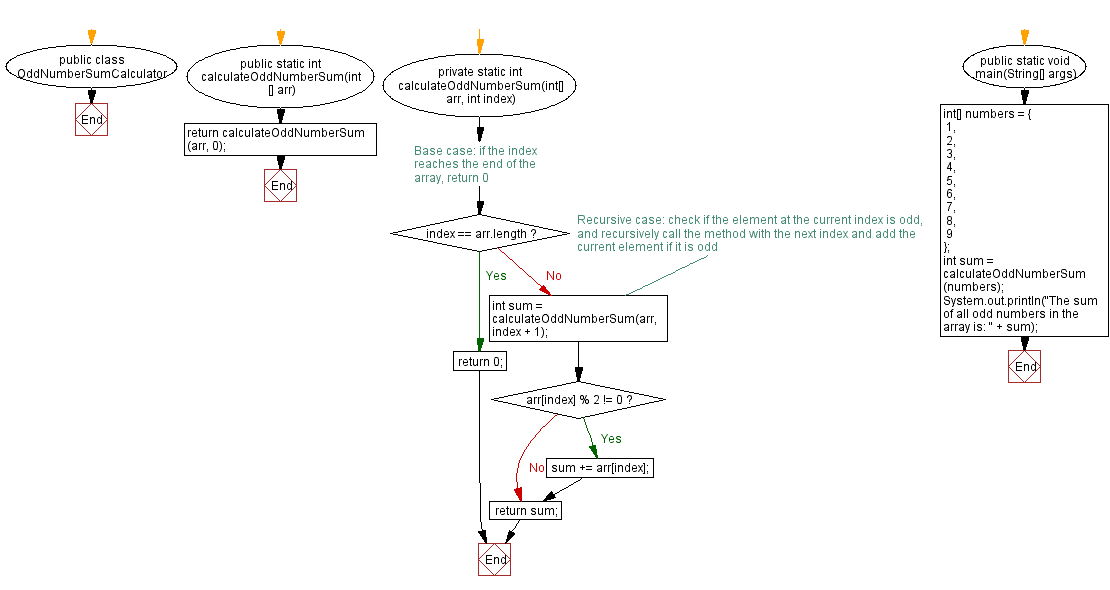
Java Code Editor:
Java Recursive Previous: Count occurrences of a specific element.
Java Recursive Next: Find the length of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics