Java: Check if a given string contains another string
Java String: Exercise-100 with Solution
Write a Java program to check if a given string contains another string. Returns true or false.
Visual Presentation:
Sample Solution:
Java Code:
// Define a class named Main
public class Main {
// The main method, entry point of the program
public static void main(String[] args) {
// Define the main string
String main_string = "Java is the foundation for virtually every type of " +
"networked application and is the global standard for developing and " +
" delivering embedded and mobile applications, games, Web-based content, " +
" and enterprise software. With more than 9 million developers worldwide," +
" Java enables you to efficiently develop, deploy and use exciting applications and services.";
// Display the original string
System.out.println("Original string:");
System.out.println(main_string);
// Define two sub-strings to search within the main string
String sub_string1 = "million";
String sub_string2 = "millions";
// Check if the first sub-string is present in the main string
boolean result1 = is_present(main_string, sub_string1);
System.out.println("\nIs '" + sub_string1 + "'" + " present in the said text?");
System.out.println(result1);
// Check if the second sub-string is present in the main string
boolean result2 = is_present(main_string, sub_string2);
System.out.println("\nIs '" + sub_string2 + "'" + " present in the said text?");
System.out.println(result2);
}
// Method to check if a sub-string is present in the main string
public static boolean is_present(String main_string, String sub_string) {
// Check for null or empty strings
if (main_string == null || sub_string == null || main_string.isEmpty() || sub_string.isEmpty()) {
return false;
}
// Check if the sub-string exists in the main string
return main_string.indexOf(sub_string) != -1;
}
}
Sample Output:
Original string: Java is the foundation for virtually every type of networked application and is the global standard for developing and delivering embedded and mobile applications, games, Web-based content, and enterprise software. With more than 9 million developers worldwide, Java enables you to efficiently develop, deploy and use exciting applications and services. Is 'million' present in the said text? true Is 'millions' present in the said text? false
Flowchart:
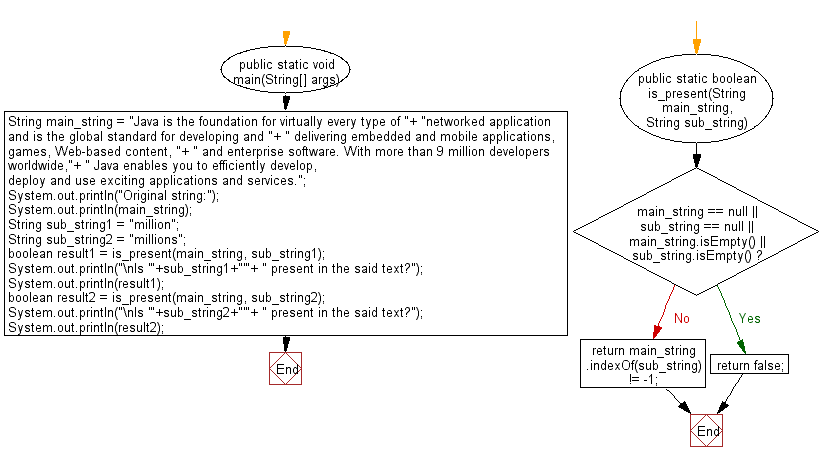
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to return a new string using every character of even positions from a given string.
Next: Write a Java program to test if a given string contains only digits. Return true or false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/string/java-string-exercise-100.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics