Java: Read a string and if one or both of the first two characters is equal to specified character return without those characters otherwise return the string unchanged
Java String: Exercise-67 with Solution
Write a Java program to read a string and remove the first two characters if one or both are equal to a specified character, otherwise leave them unchanged.
Visual Presentation:
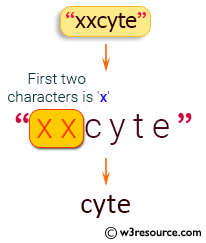
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to exclude 'x' characters at the first two positions from the input string
public String exceptTwoO(String stng) {
String temp = ""; // Create an empty string
// Iterate through each character in the input string
for (int i = 0; i < stng.length(); i++) {
// If the current index is 0 and the character is not 'x', append the character to the temporary string
if (i == 0 && stng.charAt(i) != 'x')
temp += stng.charAt(i);
// If the current index is 1 and the character is not 'x', append the character to the temporary string
else if (i == 1 && stng.charAt(i) != 'x')
temp += stng.charAt(i);
// If the current index is greater than 1, append the character to the temporary string
else if (i > 1)
temp += stng.charAt(i);
}
return temp; // Return the modified string
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "xxcyte"; // Input string
// Display the given string and the new string using exceptTwoO method
System.out.println("The given strings is: " + str1);
System.out.println("The new string is: " + m.exceptTwoO(str1));
}
}
Sample Output:
The given strings is: xxcyte The new string is: cyte
Flowchart:
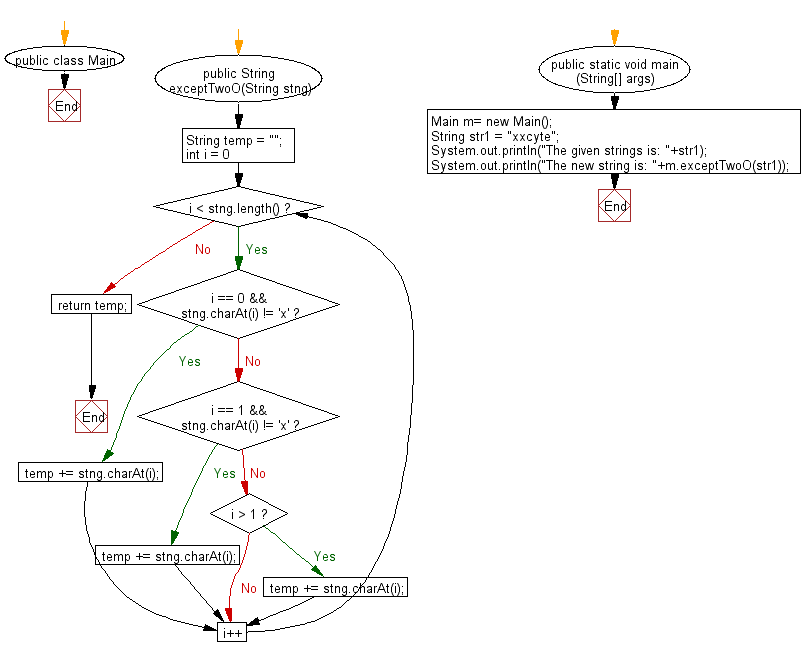
Visual Presentation:
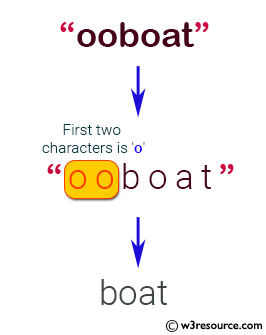
Java Code:
import java.util.*;
public class Main
{
public String exceptTwoO(String stng)
{
String temp = "";
for (int i = 0; i < stng.length(); i++)
{
if (i == 0 && stng.charAt(i) != 'o')
temp += stng.charAt(i);
else if (i == 1 && stng.charAt(i) != 'o')
temp += stng.charAt(i);
else if (i > 1)
temp += stng.charAt(i);
}
return temp;
}
public static void main (String[] args)
{
Main m= new Main();
String str1 = "ooboat";
System.out.println("The given strings is: "+str1);
System.out.println("The new string is: "+m.exceptTwoO(str1));
}
}
Sample Output:
The given strings is: ooboat The new string is: boat
Flowchart:
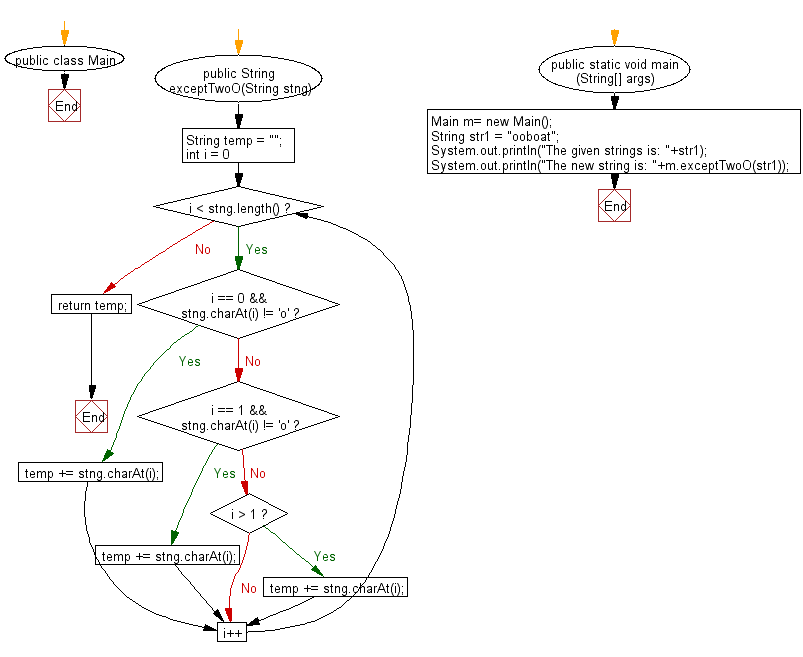
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to read a string and return the string without the first two characters. Keep the first character if it is 'g' and keep the second character if it is 'h'.
Next: Write a Java program to read a string and returns after removing a specified character and its immediate left and right characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/string/java-string-exercise-67.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics