Testing Java Singleton class for Multi-Threading with JUnit
Java Unit Test: Exercise-9 with Solution
Write a Java program that tests a singleton class, ensuring it behaves as expected in a multi-threaded environment.
Sample Solution:
Java Code:
// Singleton.java
public class Singleton {
private static volatile Singleton instance;
private Singleton() {
// Private constructor to prevent instantiation
}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
// SingletonTest.java
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class SingletonTest {
@Test
public void testSingletonInstance() throws InterruptedException {
// Create multiple threads to access the singleton instance concurrently
Thread thread1 = new Thread(() -> {
Singleton instance1 = Singleton.getInstance();
try {
Thread.sleep(100); // Simulate some work in the thread
} catch (InterruptedException e) {
e.printStackTrace();
}
Singleton instance2 = Singleton.getInstance();
// Assert that both instances obtained in the same thread are the same
assertEquals(instance1, instance2);
});
Thread thread2 = new Thread(() -> {
Singleton instance3 = Singleton.getInstance();
try {
Thread.sleep(100); // Simulate some work in the thread
} catch (InterruptedException e) {
e.printStackTrace();
}
Singleton instance4 = Singleton.getInstance();
// Assert that both instances obtained in the same thread are the same
assertEquals(instance3, instance4);
});
// Start the threads
thread1.start();
thread2.start();
// Wait for threads to finish
thread1.join();
thread2.join();
}
public static void main(String[] args) {
// Run the JUnit test
org.junit.runner.JUnitCore.main("SingletonTest");
}
}
Sample Output:
JUnit version 4.10 . Time: 0.114 OK (1 test)
Explanation:
The above exercise code consists of two Java classes:
- Singleton.java
- This class represents a singleton pattern implementation using double-check locking to ensure thread safety during lazy initialization.
- The class has a private static volatile instance variable, which is the single instance of the class.
- The constructor is private to prevent external instantiation.
- The "getInstance()" method is a static method that returns the singleton instance. It uses double-check locking to ensure that only one instance is created, even in a multi-threaded environment.
- SingletonTest.java:
- This class contains a JUnit test to verify the behavior of the "Singleton" class in a multi-threaded environment.
- The "testSingletonInstance()" method creates two threads, each trying to obtain the singleton instance concurrently.
- In each thread, it asserts that the instances obtained before and after a short sleep are the same, ensuring thread safety.
- The "main" method is provided for running the JUnit test using "JUnitCore".
Flowchart:
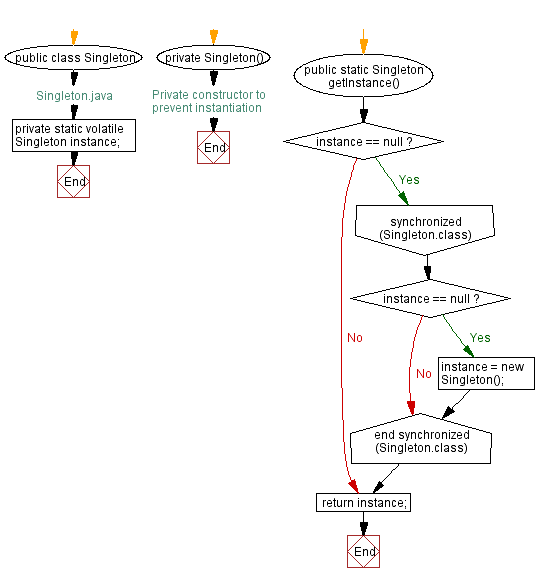
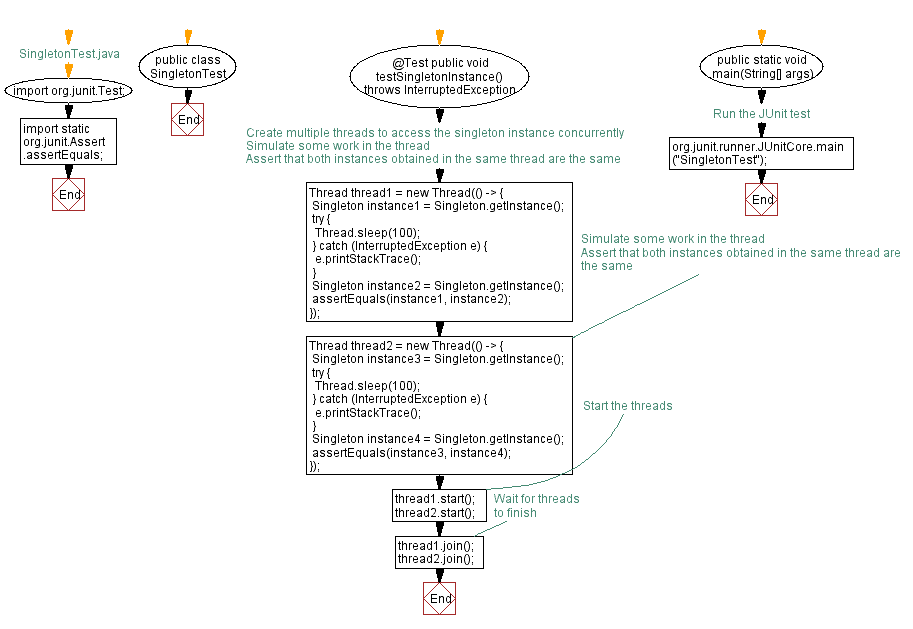
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java Testing Private methods with reflection: ExampleClass demonstration.
Next: Java Application Integration testing with JUnit: UserService and OrderService Interaction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/unittest/java-unittest-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics