JavaScript: Convert a string to kebab case
JavaScript fundamental (ES6 Syntax): Exercise-123 with Solution
String to Kebab Case
Write a JavaScript program to convert a string to kebab case.
Note: Break the string into words and combine them adding - as a separator, using a regexp.
- Use String.prototype.match() to break the string into words using an appropriate regexp.
- Use Array.prototype.map(), Array.prototype.join() and String.prototype.toLowerCase() to combine them, adding - as a separator.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'toKebabCase' function.
const toKebabCase = str =>
str &&
str
.match(/[A-Z]{2,}(?=[A-Z][a-z]+[0-9]*|\b)|[A-Z]?[a-z]+[0-9]*|[A-Z]|[0-9]+/g)
.map(x => x.toLowerCase())
.join('-');
// Test the 'toKebabCase' function with sample inputs.
console.log(toKebabCase('camelCase')); // Output: camel-case
console.log(toKebabCase('some text')); // Output: some-text
console.log(toKebabCase('some-mixed_string With spaces_underscores-and-hyphens')); // Output: some-mixed-string-with-spaces-underscores-and-hyphens
console.log(toKebabCase('AllThe-small Things')); // Output: all-the-small-things
console.log(toKebabCase('IAmListeningToFMWhileLoadingDifferentURLOnMyBrowserAndAlsoEditingSomeXMLAndHTML')); // Output: i-am-listening-to-fm-while-loading-different-url-on-my-browser-and-also-editing-some-xml-and-html
Output:
camel-case some-text some-mixed-string-with-spaces-underscores-and-hyphens all-the-small-things i-am-listening-to-fm-while-loading-different-url-on-my-browser-and-also-editing-some-xml-and-html
Visual Presentation:
Flowchart:
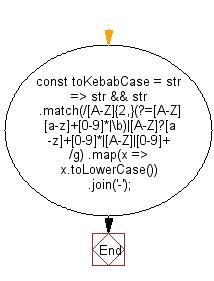
Live Demo:
See the Pen javascript-basic-exercise-123-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a given string into kebab-case by replacing spaces and punctuation with hyphens.
- Write a JavaScript function that transforms camelCase or PascalCase strings into lowercase kebab-case.
- Write a JavaScript program that normalizes and lowercases a string, then replaces non-word characters with hyphens.
Go to:
PREV : Add Ordinal Suffix to Number.
NEXT : Reduce Array-Like to Hash Map.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.