JavaScript: Remove elements from an array for which the given function returns false
JavaScript fundamental (ES6 Syntax): Exercise-227 with Solution
Remove Elements by Function
Write a JavaScript program to remove elements from an array for which the given function returns false.
- Use Array.prototype.filter() to find array elements that return truthy values.
- Use Array.prototype.reduce() to remove elements using Array.prototype.splice().
- The callback function is invoked with three arguments (value, index, array).
Sample Solution:
JavaScript Code:
// Define a function 'remove' to remove elements from an array based on a given condition
const remove = (arr, func) =>
Array.isArray(arr) // Check if the input is an array
? arr.filter(func).reduce((acc, val) => { // Filter elements that satisfy the given condition
arr.splice(arr.indexOf(val), 1); // Remove each filtered element from the original array
return acc.concat(val); // Accumulate removed elements in an array
}, [])
: []; // Return an empty array if the input is not an array
// Log the result of removing even numbers from the array [1, 2, 3, 4]
console.log(remove([1, 2, 3, 4], n => n % 2 === 0)); // Output: [2, 4] (even numbers removed from the array)
Output:
[2,4]
Flowchart:
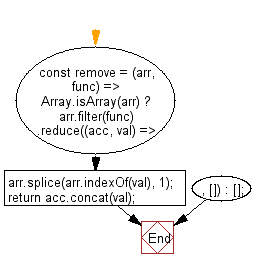
Live Demo:
See the Pen javascript-basic-exercise-227-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes elements from an array for which a provided function returns false and returns the modified array.
- Write a JavaScript function that filters an array in place by deleting elements that do not satisfy a given predicate.
- Write a JavaScript program that iterates over an array and splices out elements where the callback returns false.
- Write a JavaScript function that returns a new array with elements removed if they fail a custom test, while mutating the original array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get n random elements at unique keys from array up to the size of array.
Next: Write a JavaScript program to log the name of a function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.