JavaScript: Get a short textual representation of a month
JavaScript Datetime: Exercise-27 with Solution
Write a JavaScript function to get a short textual representation of a month, three letters (Jan through Dec).
Test Data :
dt = new Date(2015, 10, 1);
console.log(short_months(dt));
"Nov"
Sample Solution:
JavaScript Code:
// Define a property 'shortMonths' on the Date object to store an array of short month names
Date.shortMonths = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'];
// Define a JavaScript function called short_months with parameter dt (date)
function short_months(dt)
{
// Return the short month name corresponding to the month of the provided date
return Date.shortMonths[dt.getMonth()];
}
// Create a new Date object representing the current date
dt = new Date();
// Output the short month name for the current date
console.log(short_months(dt));
// Create a new Date object representing November 1, 2015
dt = new Date(2015, 10, 1);
// Output the short month name for November 1, 2015
console.log(short_months(dt));
Output:
Jun Nov
Explanation:
In the exercise above,
- The code defines a property 'shortMonths' on the "Date" object, which is an array containing the short names of months ('Jan', 'Feb', ..., 'Dec').
- It then defines a JavaScript function named "short_months()" with one parameter 'dt', representing a Date object.
- Inside the short_months function:
- It retrieves the month index (0 for January, 1 for February, ..., 11 for December) from the provided Date object "dt" using the "getMonth()" method.
- It uses this month index as an index to access the corresponding short month name from the 'Date.shortMonths' array.
- It returns the short month name.
- The code then demonstrates the usage of the short_months function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It outputs the short month name for the current date by calling the "short_months()" function with 'dt' and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 2015, using 'new Date(2015, 10, 1)'.
- It outputs the short month name for November 1, 2015, by calling the "short_months()" function with 'dt' and logging the result to the console.
Flowchart:
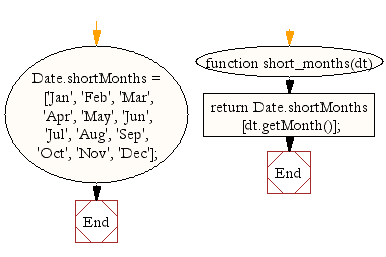
Live Demo:
See the Pen JavaScript - Get a short textual representation of a month-date-ex-27 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get a numeric representation of a month, with leading zeros (01 through 12).
Next: Write a JavaScript function to get a full numeric representation of a year (4 digits).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-date-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics