JavaScript: Check if a date is between two other dates
JavaScript Datetime: Exercise-54 with Solution
Write a JavaScript function to check if a given date is between two other dates.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function isBetweenDates that takes three Date objects as parameters: dateStart, dateEnd, and date
const isBetweenDates = (dateStart, dateEnd, date) =>
// Check if the provided date falls between the start and end dates
date > dateStart && date < dateEnd;
// Log the result of isBetweenDates with specific dates provided as arguments
console.log(isBetweenDates(
new Date(2010, 11, 20), // December 20, 2010 (start date)
new Date(2010, 11, 30), // December 30, 2010 (end date)
new Date(2010, 11, 19) // December 19, 2010 (date to check)
));
console.log(isBetweenDates(
new Date(2010, 11, 20), // December 20, 2010 (start date)
new Date(2010, 11, 30), // December 30, 2010 (end date)
new Date(2010, 11, 25) // December 25, 2010 (date to check)
));
Output:
false true
Explanation:
In the exercise above,
The code defines a function "isBetweenDates()" that takes three Date objects: "dateStart", "dateEnd", and "date". It checks if the provided 'date' falls between 'dateStart' and 'dateEnd' by comparing them using logical operators. The function returns 'true' if the 'date' is greater than 'dateStart' and less than 'dateEnd', indicating that it lies within the specified range. The code then tests this function with specific dates and logs the results to the console.
Flowchart:
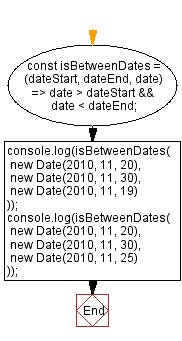
Live Demo:
See the Pen javascript-date-exercise-54 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the month start date.
Next: Check if a given date is weekday, weekend.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-date-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics