JavaScript Exercises: Nth element from the top of the stack
JavaScript Stack: Exercise-16 with Solution
Nth Element from Top
Write a JavaScript program to get the nth element from the top of the stack.
Sample Solution:
JavaScript Code:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty()) {
return "Underflow";
}
return this.items.pop();
}
peek() {
return this.items[this.items.length - 1];
}
isEmpty() {
return this.items.length === 0;
}
size() {
return this.items.length;
}
getNthFromTop(n) {
if (this.items.length < n) {
return "Index out of range";
}
return this.items[this.items.length - n];
}
displayStack(stack) {
console.log("Stack elements are:");
let str = "";
for (let i = 0; i < stack.items.length; i++)
str += stack.items[i] + " ";
return str.trim();
}
}
let stack = new Stack();
stack.push(100);
stack.push(200);
stack.push(300);
stack.push(400);
stack.push(500);
stack.push(600);
stack.push(700);
console.log(stack.displayStack(stack));
n = 2;
console.log("nth element from the top of the stack where n = "+n);
console.log(stack.getNthFromTop(n));
n = 5;
console.log("nth element from the top of the stack where n = "+n);
console.log(stack.getNthFromTop(n));
n = 7;
console.log("nth element from the top of the stack where n = "+n);
console.log(stack.getNthFromTop(n));
Sample Output:
Stack elements are: 100 200 300 400 500 600 700 nth element from the top of the stack where n = 2 600 nth element from the top of the stack where n = 5 300 nth element from the top of the stack where n = 7 100
Flowchart:
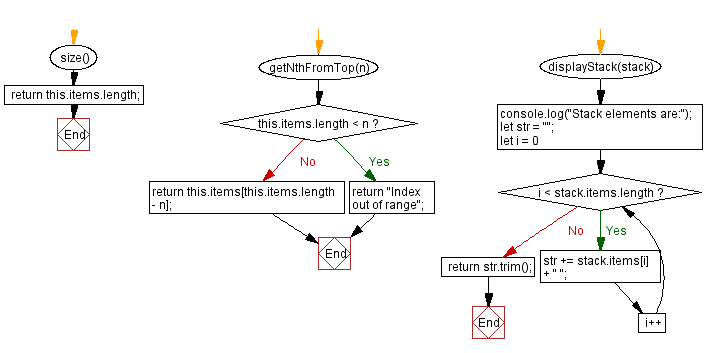
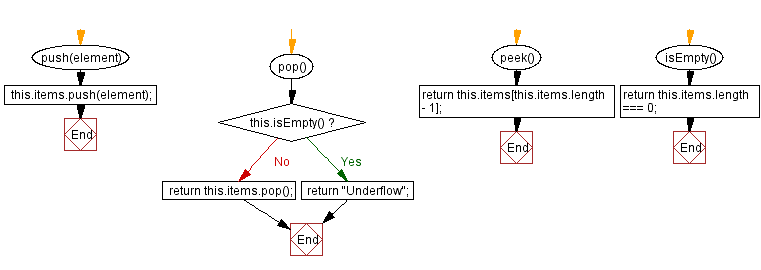
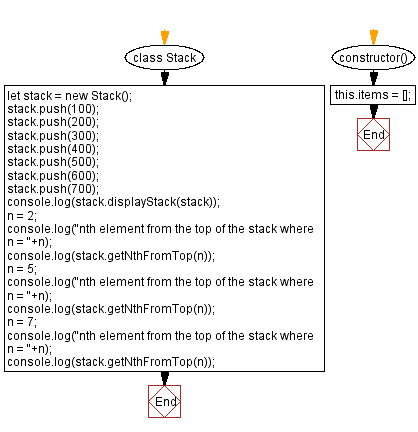
Live Demo:
See the Pen javascript-stack-exercise-16 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the nth element from the top of a stack without altering the stack.
- Write a JavaScript function that uses array indexing to retrieve the nth element from the top, handling invalid indices gracefully.
- Write a JavaScript function that iterates through a stack to locate and return the nth element from the top.
- Write a JavaScript function that validates input n and returns a descriptive error if n exceeds the stack size.
Go to:
PREV : Swap Top Two Elements.
NEXT : Nth Element from Bottom.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.