PHP Exercises: Compute the sum of values in a given array of integers except the number 17
PHP Basic Algorithm: Exercise-108 with Solution
Write a PHP program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize a variable $sum with a value of 0
$sum = 0;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is not equal to 17, increment $sum by the value of the current element
if ($nums[$i] != 17) $sum += $nums[$i];
}
// Return the sum of values in the array except for the number 17
return $sum;
}
// Call the 'test' function with an array [1, 5, 7, 9, 10, 17] and print the result
echo "Sum of values in the array of integers except the number 17: ". test([1, 5, 7, 9, 10, 17] );
?>
Sample Output:
Sum of values in the array of integers except the number 17: 32
Flowchart:
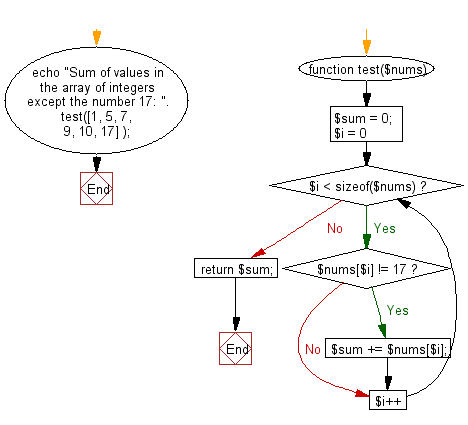
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the difference between the largest and smallest values in a given array of integers and length one or more.
Next: Write a PHP program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-108.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics