PHP Exercises: Create a new string of length 2, using first two characters of a given string
PHP Basic Algorithm: Exercise-75 with Solution
Write a PHP program to create a new string of length 2, using first two characters of a given string. If the given string length is less than 2 use '#' as missing characters.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that modifies the input string based on its length
function test($s1)
{
// Check if the length of the string is greater than or equal to 2
if (strlen($s1) >= 2)
{
// If true, extract the first two characters from the string
$s1 = substr($s1, 0, 2);
}
// If the length is greater than 0 but less than 2
else if (strlen($s1) > 0)
{
// Extract the first character and concatenate "#" to it
$s1 = substr($s1, 0, 1) . "#";
}
// If the length is 0
else
{
// Set the string to "##"
$s1 = "##";
}
// Return the modified string
return $s1;
}
// Test the 'test' function with different strings, then display the results using echo
echo test("Hello")."\n";
echo test("Python")."\n";
echo test("a")."\n";
echo test(" ")."\n";
?>
Explanation:
- Function Definition:
- The test function is defined with a single parameter:
- $s1: the input string.
- String Modification Based on Length:
- The function has three cases based on the length of $s1:
- If the length is 2 or more:
- Extracts the first two characters of $s1 using substr($s1, 0, 2).
- If the length is 1:
- Extracts the first character and appends a "#" to it, making $s1 = substr($s1, 0, 1) . "#".
- If the length is 0:
- Sets $s1 to "##".
- Return Value:
- Returns the modified $s1 based on the above conditions.
Output:
He Py a# #
Flowchart:
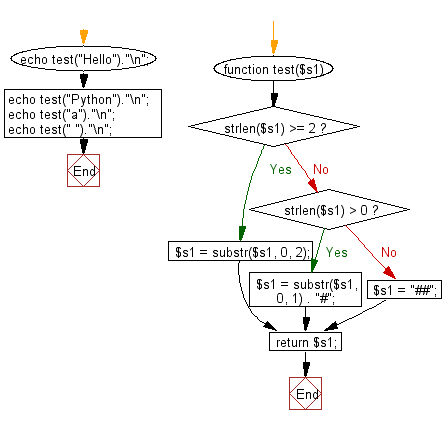
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string taking 3 characters from the middle of a given string at least 3.
Next: Write a PHP program to create a new string taking the first character from a given string and the last character from another given string. If the length of any given string is 0, use '#' as its missing character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-75.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics