PHP Database Connection: Exception handling
8. Simulated Database Connection Exception
Write a PHP program that simulates a database connection and throws an exception if it fails.
Sample Solution:
PHP Code :
<?php
class DatabaseConnectionException extends Exception {
public function __construct($message, $code = 0, Throwable $previous = null) {
parent::__construct($message, $code, $previous);
}
public function __toString() {
return __CLASS__ . ": [{$this->code}]: {$this->message}\n";
}
}
function connectToDatabase($host, $username, $password, $database) {
// Simulate a database connection attempt
$success = false;
// Check if the connection was successful
if (!$success) {
throw new DatabaseConnectionException("Failed to connect to the database.");
}
// Return the database connection object if successful
return $databaseConnection;
}
try {
$host = "localhost";
$username = "root";
$password = "password";
$database = "database";
$databaseConnection = connectToDatabase($host, $username, $password, $database);
echo "Database connection successful!";
} catch (DatabaseConnectionException $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
An error occurred: Failed to connect to the database.
Explanation:
In the above exercise, we define a custom exception class DatabaseConnectionException that extends the base Exception class. The custom exception class has a constructor that calls the parent constructor and overrides the __toString() method. This is to provide a custom string representation of the exception.
We have a function connectToDatabase() that attempts to connect to a database. In this example, we simulate a database connection attempt that always fails by setting $success to false. If the connection fails, we throw an instance of the DatabaseConnectionException class with the message "Failed to connect to the database."
In the main code, we call the connectToDatabase() function with the appropriate parameters for the database connection. If the connection fails, it throws DatabaseConnectionException, which is caught in the catch block. We display the error message using $e->getMessage().
Flowchart:
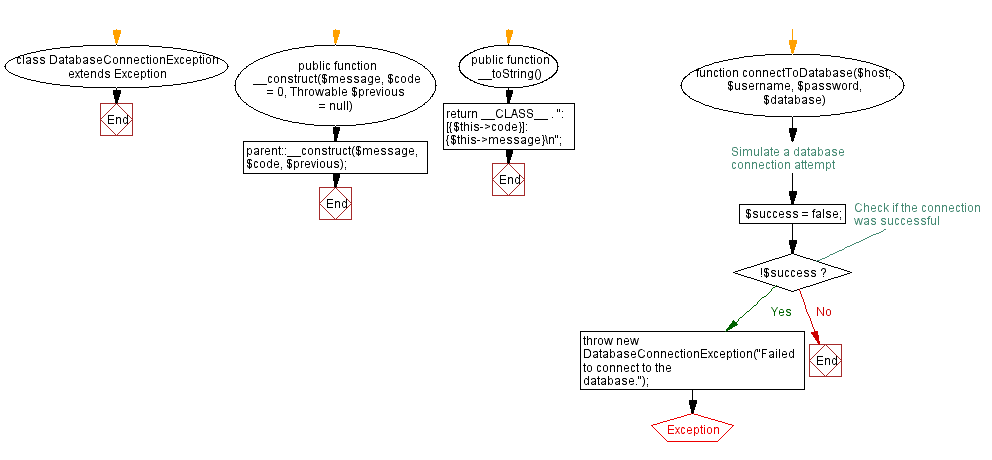
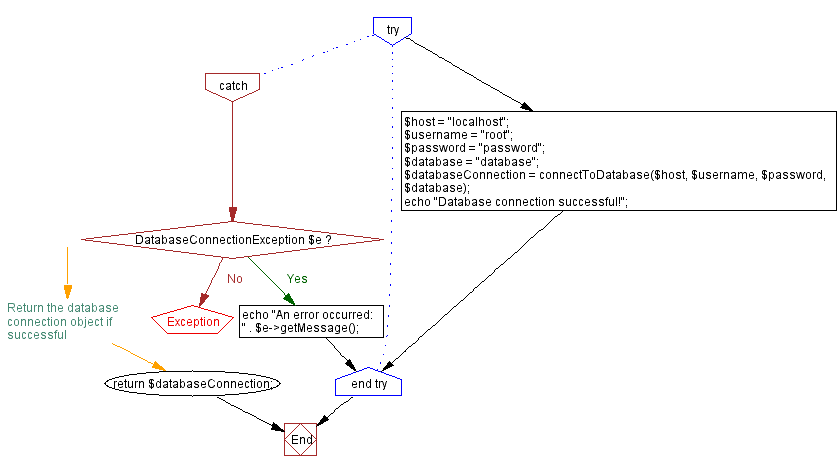
For more Practice: Solve these Related Problems:
- Write a PHP script to simulate a database connection attempt and throw an exception if connection parameters are invalid.
- Write a PHP function that mimics connecting to a database, throwing a custom exception on failure, and catching the exception to display an error message.
- Write a PHP program that uses try-catch blocks to simulate multiple database connection attempts and logs failures.
- Write a PHP script to simulate a timeout error during a database connection and handle this exception by providing a fallback mechanism.
Go to:
PREV : Multiple Catch Blocks Implementation.
NEXT : Finally Block in Exception Handling.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.