PHP interface resizable: Resizing functionality in the square class
PHP OOP: Exercise-4 with Solution
Write a PHP interface called 'Resizable' with a method 'resize()'. Implement the 'Resizable' interface in a class called 'Square' and add functionality to resize the square.
Sample Solution:
PHP Code :
<?php
interface Resizable {
public function resize($percentage);
}
class Square implements Resizable {
private $side;
public function __construct($side) {
$this->side = $side;
}
public function resize($percentage) {
$this->side = $this->side * ($percentage / 100);
}
public function getArea() {
return pow($this->side, 2);
}
public function getSide() {
return $this->side;
}
}
$square = new Square(10);
echo "Initial Side Length: " . $square->getSide() . "</br>";
$square->resize(60); // Resize the square to 60% of its original size
echo "Resized Side Length: " . $square->getSide() . "</br>";
echo "Area: " . $square->getArea() . "</br>";
?>
Sample Output:
Initial Side Length: 10 Resized Side Length: 6 Area: 36
Explanation:
In the above exercise -
- The Resizable interface defines a contract with a single method resize(). Any class that implements this interface must implement the resize() method.
- The "Square" class implements the Resizable interface and provides its own implementation for the resize() method. It also has a private property $side to represent the sides of the square.
- The resize() method in the Square class resizes the square by adjusting its side length based on the provided percentage.
- The getArea() method calculates and returns the square area using the formula: side * side.
- The getSide() method returns the current square side length.
Flowchart:
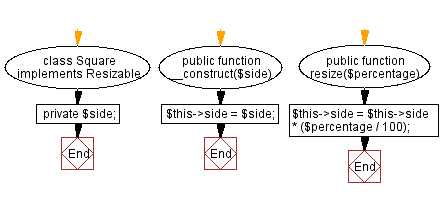
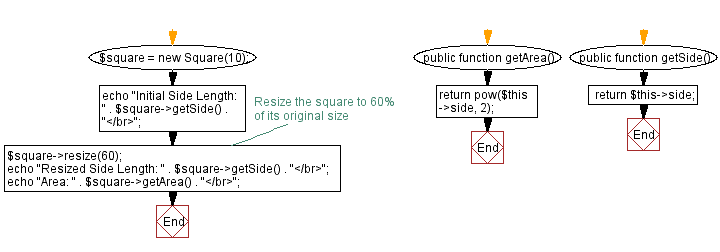
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Abstract method and subclass implementation.
Next: Display vehicle details.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/oop/php-oop-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics