Python Projects: Get a property from a nested object using a dot path
Python Project-7 with Solution
Create a Python project to get a property from a nested object using a dot path.
Sample Solution:
Python Code:
main.py
#Source: https://bit.ly/2OEBGUj
from property import get
data = {
'code': 'S0001',
'nested': {'x': 'y', 'int': 0, 'null': None},
'nums': [[12, 14, 16, 18, 20]],
'names': ['Hank Navas', 'Melinda Swatzell', 'Lucio Tardy', 'Hershel Luebke']
}
print(get(data, 'code'))
print(get(data, 'nested.x'))
print(get(data, 'nested.null'))
print(get(data, 'nums.0.2'))
print(get(data, 'nums.0.-1'))
print(get(data, 'names.0'))
print(get(data, 'names.-1'))
Flowchart:
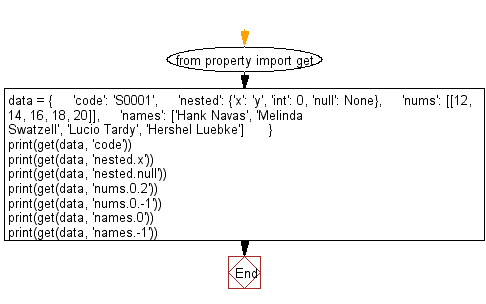
Property.py
def get(obj, path: str, default=None):
"""
Forgiving get dot prop.
If some level doesn't exist, it returns the default.
"""
value = obj
for key in path.split('.'):
if isinstance(value, list):
index = int(key)
if index < len(value):
value = value[index]
else:
return default
elif isinstance(value, dict):
if key in value:
value = value[key]
else:
return default
else:
if hasattr(value, key):
value = getattr(value, key)
else:
return default
return value
def strict_get(obj, path: str):
"""
Strict get dot prop.
If some level doesn't exist, it raises the appropriate exception.
"""
value = obj
for key in path.split('.'):
if isinstance(value, list):
value = value[int(key)]
elif isinstance(value, dict):
value = value[key]
else:
value = getattr(value, key)
return value
Output:
S0001 y None 16 20 Hank Navas Hershel Luebke
Flowchart:
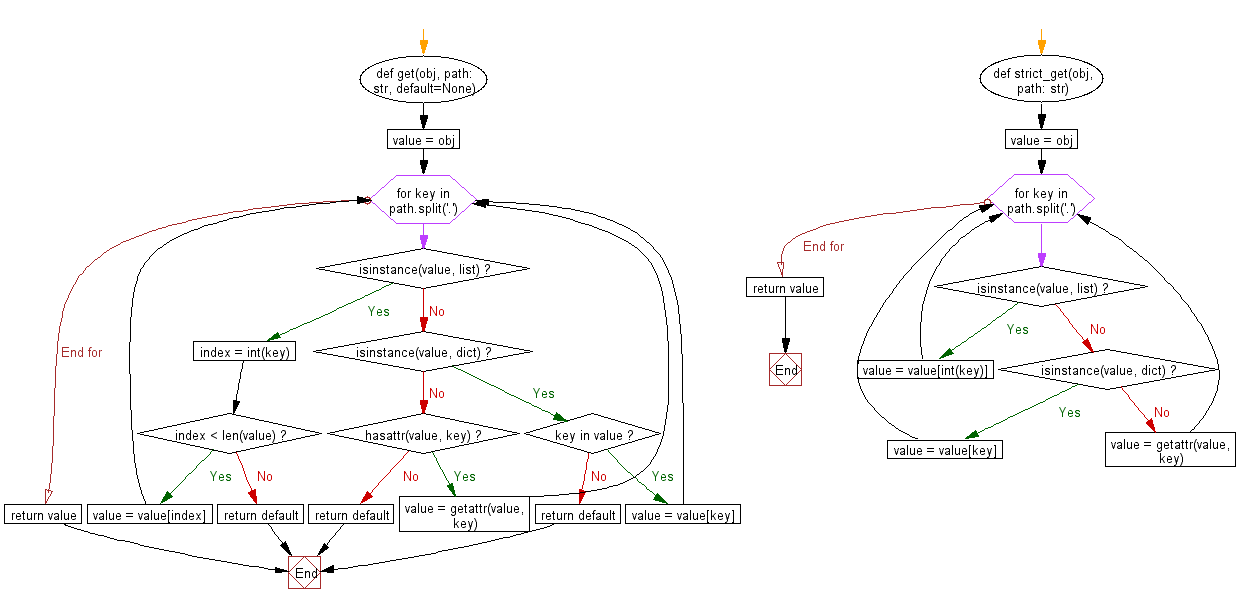
Contribute your code and comments through Disqus.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/projects/python/python-projects-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics