Python: Find a number in a given matrix which is maximum in its column and minimum in its row
Python Basic - 1: Exercise-104 with Solution
Write a Python program to find a number in a given matrix that is maximum in its column and minimum in its row.
Sample Solution:
Python Code:
# Define a function named matrix_number that takes a matrix as an argument.
def matrix_number(matrix):
# Use set operations to find the numbers that are the minimum in their row and maximum in their column.
# The set(map(min, matrix)) finds the minimum in each row, and set(map(max, zip(*matrix))) finds the maximum in each column.
result = set(map(min, matrix)) & set(map(max, zip(*matrix)))
return list(result)
# Test the function with different matrices and print the results.
# Test case 1
m1 = [[1,2], [2,3]]
print("Original matrix:", m1)
print("Number in the said matrix which is maximum in its column and minimum in its row:")
print(matrix_number(m1))
# Test case 2
m1 = [[1,2,3], [3,4,5]]
print("\nOriginal matrix:", m1)
print("Number in the said matrix which is maximum in its column and minimum in its row:")
print(matrix_number(m1))
# Test case 3
m1 = [[7,5,6], [3,4,4], [6,5,7]]
print("\nOriginal matrix:", m1)
print("Number in the said matrix which is maximum in its column and minimum in its row:")
print(matrix_number(m1))
Sample Output:
Original matrix: [[1, 2], [2, 3]] Number in the said matrix which is maximum in its column and minimum in its row: [2] Original matrix: [[1, 2, 3], [3, 4, 5]] Number in the said matrix which is maximum in its column and minimum in its row: [3] Original matrix: [[7, 5, 6], [3, 4, 4], [6, 5, 7]] Number in the said matrix which is maximum in its column and minimum in its row: [5]
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named matrix_number that takes a matrix as an argument.
- Set operations:
- The function uses set operations to find the numbers that are the minimum in their row and maximum in their column. The expression set(map(min, matrix)) finds the minimum in each row, and set(map(max, zip(*matrix))) finds the maximum in each column.
- Return statement:
- The function returns the list of numbers that satisfy both conditions.
Flowchart:
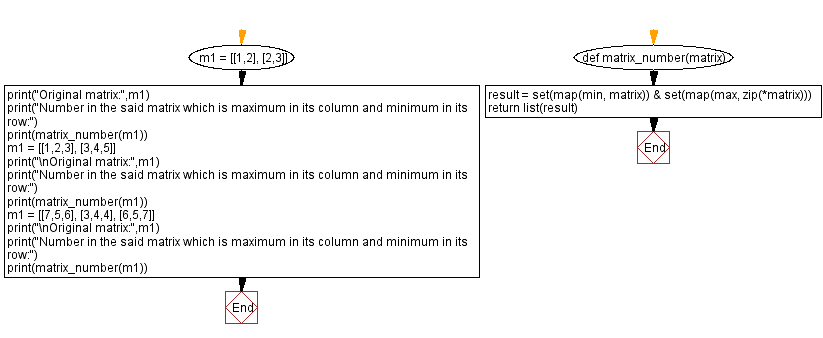
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check whether two given lines are parallel or not.
Next: Write a Python program to check whether a given sequence is linear, quadratic or cubic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics