Python: Create a key-value list pairings in a given dictionary
Python dictionary: Exercise-64 with Solution
Write a Python program that creates key-value list pairings within a dictionary.
Visual Presentation:
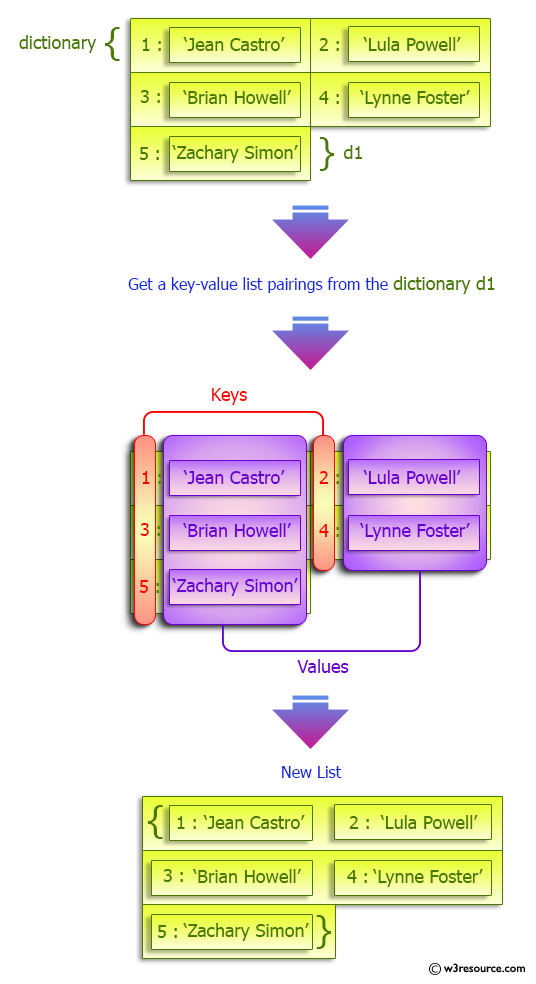
Sample Solution:
Python Code:
# Import the 'product' function from the 'itertools' module.
from itertools import product
# Define a function 'test' that takes a dictionary 'dictt' as an argument.
def test(dictt):
# Use a list comprehension to create a list of dictionaries by pairing keys from 'dictt'
# with all possible combinations of values from the input dictionary.
result = [dict(zip(dictt, sub)) for sub in product(*dictt.values())]
return result
# Create a dictionary 'students' where keys are student IDs and values are lists with one name each.
students = {1: ['Jean Castro'], 2: ['Lula Powell'], 3: ['Brian Howell'], 4: ['Lynne Foster'], 5: ['Zachary Simon']}
# Print a message indicating the start of the code section and display the original dictionary.
print("\nOriginal dictionary:")
print(students)
# Print a message indicating the purpose and demonstrate the 'test' function's usage
# to generate key-value list pairings from the dictionary.
print("\nA key-value list pairings of the said dictionary:")
print(test(students))
Sample Output:
Original dictionary: {1: ['Jean Castro'], 2: ['Lula Powell'], 3: ['Brian Howell'], 4: ['Lynne Foster'], 5: ['Zachary Simon']} A key-value list pairings of the said dictionary: [{1: 'Jean Castro', 2: 'Lula Powell', 3: 'Brian Howell', 4: 'Lynne Foster', 5: 'Zachary Simon'}]
Flowchart:
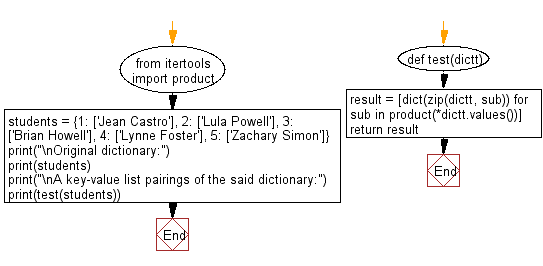
Python Code Editor:
Previous: Write a Python program to convert a given list of lists to a dictionary.
Next: Write a Python program to get the total length of all values of a given dictionary with string values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics