Python Enum: Iterate over an enum class and display individual member and their value
Python Enum: Exercise-2 with Solution
Write a Python program that iterates over an enum class and displays each member and their value.
Sample Solution:
Python Code:
from enum import Enum
class Country(Enum):
Afghanistan = 93
Albania = 355
Algeria = 213
Andorra = 376
Angola = 244
Antarctica = 672
for data in Country:
print('{:15} = {}'.format(data.name, data.value))
Sample Output:
Afghanistan = 93 Albania = 355 Algeria = 213 Andorra = 376 Angola = 244 Antarctica = 672
Flowchart:
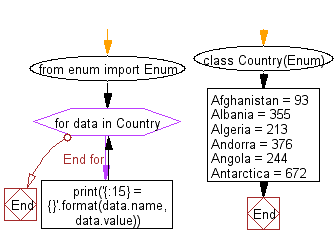
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to create an Enum object and display a member name and value.
Next: Write a Python program to display all the member name of an enum class ordered by their values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/enum/python-enum-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics