Python list empty check using Boolean logic
4. List Emptiness Checker
Write a Python function that checks if a given list is empty or not using boolean logic.
Sample Solution:
Code:
def is_empty_list(lst):
return not bool(lst)
def main():
try:
input_list = eval(input("Input a list (e.g., [1, 2, 3, 4]): "))
if isinstance(input_list, list):
if is_empty_list(input_list):
print("The list is empty.")
else:
print("The list is not empty.")
else:
print("Invalid input. Please enter a valid list.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a list (e.g., [1, 2, 3, 4]): [1,2,3] The list is not empty.
Input a list (e.g., [1, 2, 3, 4]): [] The list is empty.
In the above exercise the program prompts the user to input a list (using eval to convert the input string to a list), and then uses the "is_empty_list()" function to determine if the list is empty or not. Based on the results, it prints the appropriate message.
Flowchart:
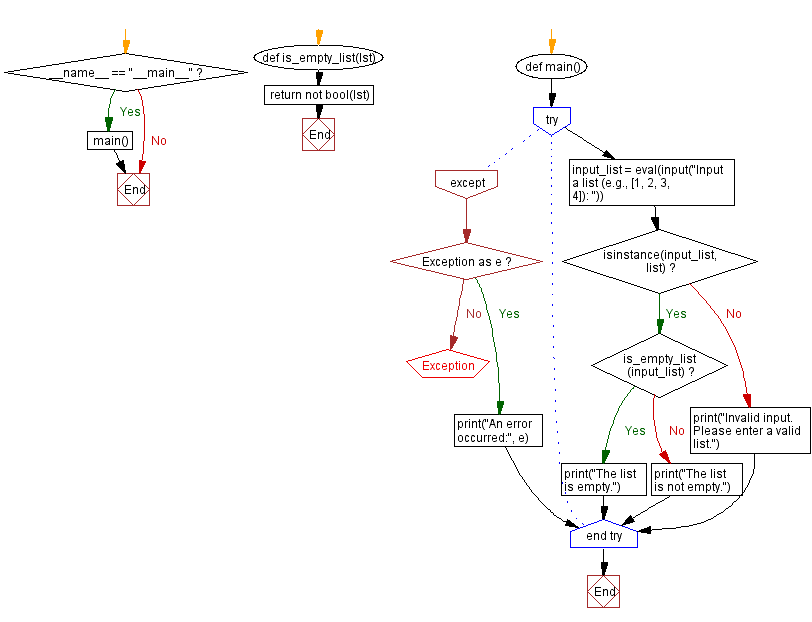
For more Practice: Solve these Related Problems:
- Write a Python program to determine if a list is empty by checking its truth value and print a corresponding message.
- Write a Python function that returns True if a given list is empty, and False otherwise, without using len().
- Write a Python script to check multiple lists for emptiness using boolean logic and then count how many of them are non-empty.
- Write a Python program to evaluate a list's emptiness using a conditional expression and print either "List is empty" or "List has elements."
Go to:
Previous: Voting eligibility checker.
Next: Python leap year checker using Boolean logic.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.