Calculating average with NumPy memory view in Python
4. Memory View Average from NumPy Array
Write a Python program that creates a memory view from a NumPy array and calculates the average of its elements.
Sample Solution:
Code:
import numpy as np
# Create a NumPy array
nums = np.array([2, 4, 6, 8, 10], dtype=np.int32)
# Create a memory view from the NumPy array
memory_view = memoryview(nums)
# Calculate the average using the memory view
total = 0
for element in memory_view:
total += element
average = total / len(memory_view)
print("NumPy Array:", nums)
print("Memory View:", memory_view)
print("Average:", average)
Output:
NumPy Array: [ 2 4 6 8 10] Memory View: <memory at 0x00000266DBB4E348> Average: 6.0
In this exercise above, we first import the "numpy" library and create a NumPy array called 'nums' with some integer values. Then, we create a memory view called 'memory_view' from the 'nums' array. We calculate the average by iterating through the memory view elements and summing them up. Finally, divide the total by the memory view length to get the average.
Flowchart:
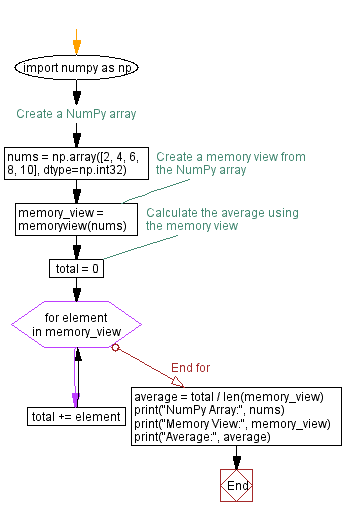
For more Practice: Solve these Related Problems:
- Write a Python program to create a memory view from a NumPy array and calculate the average of its elements without converting the view back to an array.
- Write a Python script to generate a random NumPy array, create a memory view, and then compute the mean value using a loop over the memory view.
- Write a Python function that accepts a NumPy array, creates a memory view, and returns the average of its elements along with the array’s shape.
- Write a Python program to compare the average computed from a NumPy array directly and via a memory view to verify consistency.
Go to:
Previous: Creating NumPy memory views: 1D and 3D array examples in Python.
Next: Modifying binary files using Python memory views.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.