Python NamedTuple function example: Get item information
5. Item NamedTuple Sum
Write a Python function that takes a namedtuple as input of the item's name and price. Returns the item's name and price.
Sample Solution:
Code:
from collections import namedtuple
# Define a NamedTuple named 'Item' with fields 'name' and 'price'
Item = namedtuple("Item", ["name", "price"])
def get_item_info(item):
return item.name, item.price
# Create instances of the Item NamedTuple
items = [
Item(name="Mobile", price=300.00),
Item(name="Desktop", price=1500.00),
Item(name="Laptop", price=1200.00)
]
# Print the information of each item
for item in items:
item_name, item_price = get_item_info(item)
print("Item Name:", item_name)
print("Item Price:", item_price)
print()
Output:
Item Name: Mobile Item Price: 300.0 Item Name: Desktop Item Price: 1500.0 Item Name: Laptop Item Price: 1200.0
In the exercise above, we define a NamedTuple named "Item" with fields 'name' and 'price'. The "get_item_info()" function takes a NamedTuple representing an item's name and price as input and returns a tuple containing the item's name and price.
Next we create a list 'items' containing instances of the "Item" NamedTuple representing different items. Using the "get_item_info()" function, we iterate through the list and print the information of each item.
Flowchart:
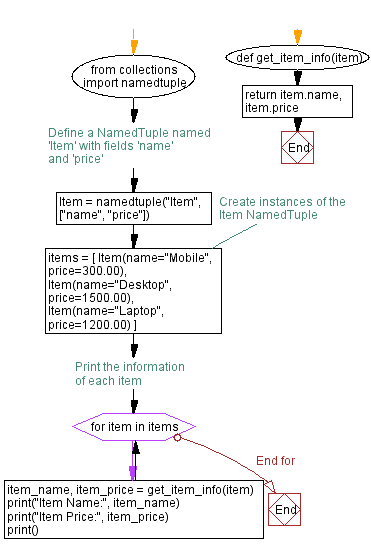
For more Practice: Solve these Related Problems:
- Write a Python function that takes a NamedTuple with fields: item_name and price, and returns a tuple containing the item_name and price increased by a discount factor.
- Write a Python program that accepts two `Item` NamedTuples, compares their prices, and returns the one with the lower price.
- Write a Python script to create a list of `Item` NamedTuples and then compute the total price of all items using your function.
- Write a Python function that takes an `Item` NamedTuple and returns its name and price only if the price exceeds a certain threshold; otherwise, returns None.
Go to:
Previous: Python NamedTuple example: Defining a student NamedTuple.
Next: Python NamedTuple function: Calculate average grade.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.