Python NamedTuple example: Triangle area calculation
8. Triangle NamedTuple Area
Write a Python program that defines a NamedTuple named "Triangle" with fields 'side1', 'side2', and 'side3'. Now write a function that takes a "Triangle" NamedTuple as input and calculates its area.
Sample Solution:
Code:
from collections import namedtuple
import math
# Define a Triangle NamedTuple
Triangle = namedtuple('Triangle', ['side1', 'side2', 'side3'])
# Function to calculate the area of a triangle
def triangle_area(triangle):
print("Sides of the triangle:",triangle.side1,',',triangle.side2,',',triangle.side3)
# Calculate the semi-perimeter (half the sum of all its
# sides) of the triangle
s = (triangle.side1 + triangle.side2 + triangle.side3) / 2
# Calculate the area using Heron's formula
area = math.sqrt(s * (s - triangle.side1) * (s - triangle.side2) * (s - triangle.side3))
return area
# Create an instance of the Triangle NamedTuple
triangle_instance = Triangle(side1=4, side2=5, side3=7)
# Calculate and print the area of the triangle
area = triangle_area(triangle_instance)
print("Triangle Area:", area)
Output:
Sides of the triangle: 4 , 5 , 7 Triangle Area: 9.797958971132712
In the exercise above, we define a "Triangle" NamedTuple with 'side1', 'side2', and 'side3' fields. We also define a function "triangle_area()" that takes a "Triangle" NamedTuple as input and calculates its area using Heron's formula. Next, we create an instance of the "Triangle" NamedTuple and use the "triangle_area()" function to calculate its area.
Flowchart:
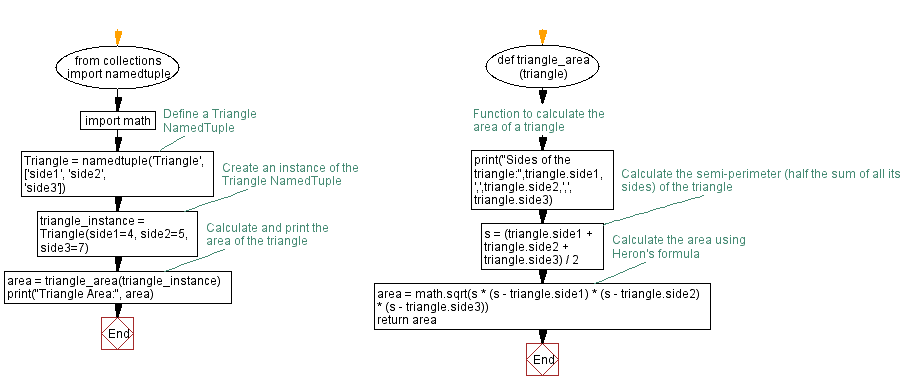
For more Practice: Solve these Related Problems:
- Write a Python program to define a NamedTuple 'Triangle' with fields: side1, side2, and side3, then compute and print its area using Heron’s formula.
- Write a Python function to validate whether a given 'Triangle' NamedTuple can form a valid triangle before calculating its area.
- Write a Python script to compare the areas of two 'Triangle' NamedTuples and output the triangle with the larger area.
- Write a Python program to create a list of 'Triangle' NamedTuples and then sort them by their calculated area.
Go to:
Previous: Python NamedTuple example: Circle attributes.
Next: Python NamedTuple example: Car attributes.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.