Python File I/O: Extract characters from various text files and puts them into a list
19. Extract Characters to List
Write a Python program to extract characters from various text files and puts them into a list.
words1.txt Welcome to the Python Wiki, a user-editable compendium of knowledge based around the Python programming language. Some pages are protected against casual editing - see WikiEditingGuidelines for more information about editing content.
words2.txt Python is a great object-oriented, interpreted, and interactive programming language. It is often compared (favorably of course :-) ) to Lisp, Tcl, Perl, Ruby, C#, Visual Basic, Visual Fox Pro, Scheme or Java... and it's much more fun.
Sample Solution:
Python Code:
import glob
char_list = []
files_list = glob.glob("*.txt")
for file_elem in files_list:
with open(file_elem, "r") as f:
char_list.append(f.read())
print(char_list)
Sample Output:
["Python is a great object-oriented, interpreted, and interactive\nprogramming language. It is often compared (favorably of course :-) )\nto Lisp, Tcl, Perl, Ruby, C#, Visual Basic, Visual Fox Pro, Scheme or Java...\nand it's much more fun.", 'Welcome to the Python Wiki, a user-editable compendium of knowledge\nbased around the Python programming language. Some pages are protected\nagainst casual editing - see WikiEditingGuidelines for more information\nabout editing content.\n']
Flowchart:
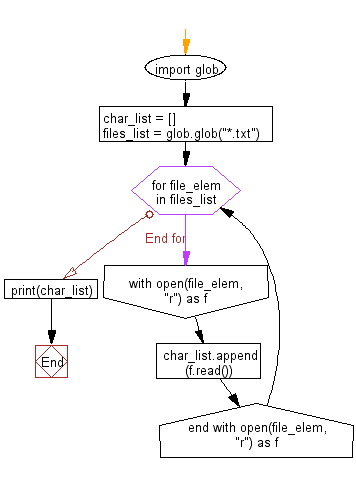
For more Practice: Solve these Related Problems:
- Write a Python program to read multiple text files and extract all unique alphabetic characters into a sorted list.
- Write a Python script to extract every character from a text file and count the frequency of each, storing the results in a list of tuples.
- Write a Python program to read a file and create a list of characters that appear more than once.
- Write a Python program to extract only the alphabetic characters from a file and store them in a list, ignoring digits and punctuation.
Go to:
Previous: Write a Python program that takes a text file as input and returns the number of words of a given text file.
Next: Write a Python program to generate 26 text files named A.txt, B.txt, and so on up to Z.txt.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.