Python: Remove None value from a given list
Python List: Exercise - 166 with Solution
Remove None from List
Write a Python program to remove the None value from a given list.
Pictorial Presentation:
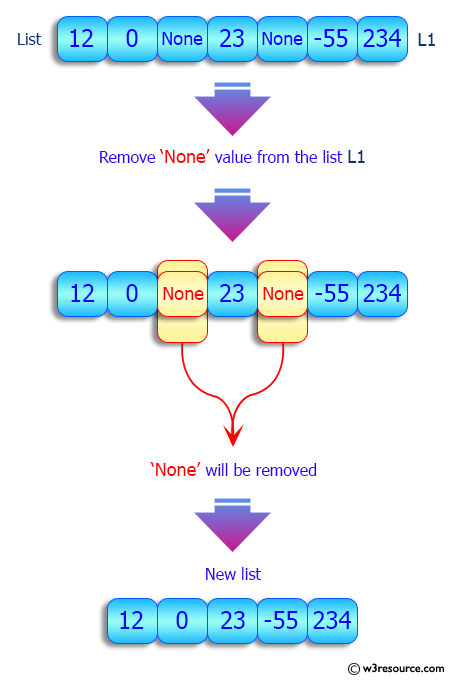
Sample Solution-1:
Python Code:
# Define a function called 'remove_none' that removes 'None' values from a list 'nums'.
def remove_none(nums):
# Use a list comprehension to create a new list 'result' containing elements that are not 'None'.
result = [x for x in nums if x is not None]
return result
# Create a list 'nums' containing various values, including 'None' elements.
nums = [12, 0, None, 23, None, -55, 234, 89, None, 0, 6, -12]
# Print a message indicating the original list.
print("Original list:")
print(nums)
# Print a message indicating the intention to remove 'None' values from the list.
print("\nRemove None value from the said list:")
# Call the 'remove_none' function to remove 'None' values from the list 'nums' and print the result.
print(remove_none(nums))
Sample Output:
Original list: [12, 0, None, 23, None, -55, 234, 89, None, 0, 6, -12] Remove None value from the said list: [12, 0, 23, -55, 234, 89, 0, 6, -12]
Flowchart:
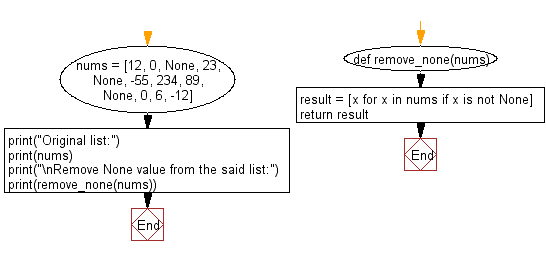
Sample Solution-2:
Python Code:
# Define a function called 'remove_none' that removes 'None' values from a list 'nums'.
def remove_none(nums):
# Use the 'filter' function with 'None' as the filter function to remove 'None' values from the list.
result = list(filter(None, nums))
return result
# Create a list 'nums' containing various values, including 'None' elements.
nums = [12, 0, None, 23, None, -55, 234, 89, None, 0, 6, -12]
# Print a message indicating the original list.
print("Original list:")
print(nums)
# Print a message indicating the intention to remove 'None' values from the list.
print("\nRemove None value from the said list:")
# Call the 'remove_none' function to remove 'None' values from the list 'nums' and print the result.
print(remove_none(nums))
Sample Output:
Original list: [12, 0, None, 23, None, -55, 234, 89, None, 0, 6, -12] Remove None value from the said list: [12, 23, -55, 234, 89, 6, -12]
Flowchart:
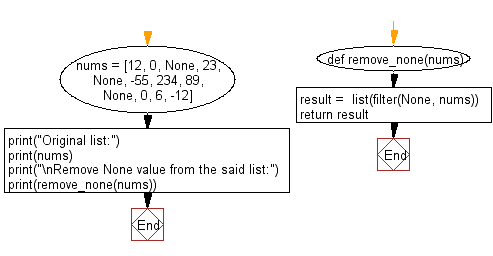
Python Code Editor:
<Previous: Write a Python program to split a given list into specified sized chunks.
Next: Write a Python program to convert a given list of strings into list of lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-166.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics