Python: Sum of two lowest negative numbers of a given array of integers
Sum Two Lowest Negatives
Write a Python program to calculate the sum of two lowest negative numbers in a given array of integers.
An integer (from the Latin integer meaning "whole") is colloquially defined as a number that can be written without a fractional component. For example, 21, 4, 0, and −2048 are integers.
Sample Solution-1:
Python Code:
# Define a function called 'test' that calculates the sum of the two lowest negative numbers in a list of integers.
def test(nums):
# Filter the list to include only negative numbers and sort them in ascending order.
result = sorted([item for item in nums if item < 0])
# Calculate the sum of the two lowest negative numbers, which are now the first two elements in the sorted list.
return result[0] + result[1]
# Create a list 'nums' containing a mix of positive and negative integers.
nums = [-14, 15, -10, -11, -12, -13, 16, 17, 18, 19, 20]
# Print a message indicating the original list elements.
print("Original list elements:")
print(nums)
# Calculate and print the sum of the two lowest negative numbers in the list using the 'test' function.
print("Sum of two lowest negative numbers of the said array of integers: ", test(nums))
# Create another list 'nums' with different values.
nums = [-4, 5, -2, 0, 3, -1, 4, 9]
# Print a message indicating the original list elements.
print("\nOriginal list elements:")
print(nums)
# Calculate and print the sum of the two lowest negative numbers in the second list using the 'test' function.
print("Sum of two lowest negative numbers of the said array of integers: ", test(nums))
Sample Output:
Original list elements: [-14, 15, -10, -11, -12, -13, 16, 17, 18, 19, 20] Sum of two lowest negative numbers of the said array of integers: -27 Original list elements: [-4, 5, -2, 0, 3, -1, 4, 9] Sum of two lowest negative numbers of the said array of integers: -6
Flowchart:
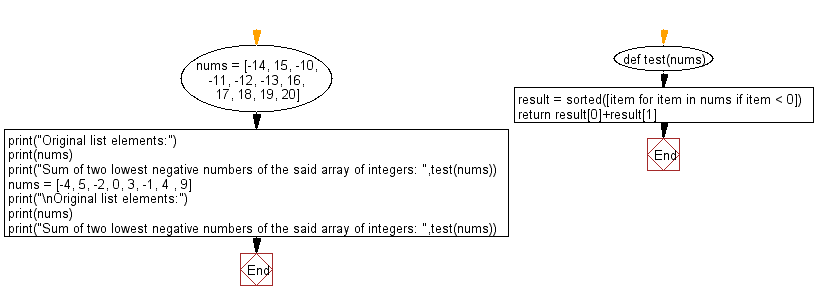
Sample Solution-2:
Python Code:
# Define a function called 'test' that calculates the sum of the two lowest negative numbers in a list of integers.
def test(nums):
# Sort the list of numbers in ascending order and select the first two negative numbers using slicing.
negative_numbers = [el for el in sorted(nums) if el < 0][:2]
# Calculate and return the sum of the two lowest negative numbers.
return sum(negative_numbers)
# Create a list 'nums' containing a mix of positive and negative integers.
nums = [-14, 15, -10, -11, -12, -13, 16, 17, 18, 19, 20]
# Print a message indicating the original list elements.
print("Original list elements:")
print(nums)
# Calculate and print the sum of the two lowest negative numbers in the list using the 'test' function.
print("Sum of two lowest negative numbers of the said array of integers: ", test(nums))
# Create another list 'nums' with different values.
nums = [-4, 5, -2, 0, 3, -1, 4, 9]
# Print a message indicating the original list elements.
print("\nOriginal list elements:")
print(nums)
# Calculate and print the sum of the two lowest negative numbers in the second list using the 'test' function.
print("Sum of two lowest negative numbers of the said array of integers: ", test(nums))
Sample Output:
Original list elements: [-14, 15, -10, -11, -12, -13, 16, 17, 18, 19, 20] Sum of two lowest negative numbers of the said array of integers: -27 Original list elements: [-4, 5, -2, 0, 3, -1, 4, 9] Sum of two lowest negative numbers of the said array of integers: -6
Flowchart:
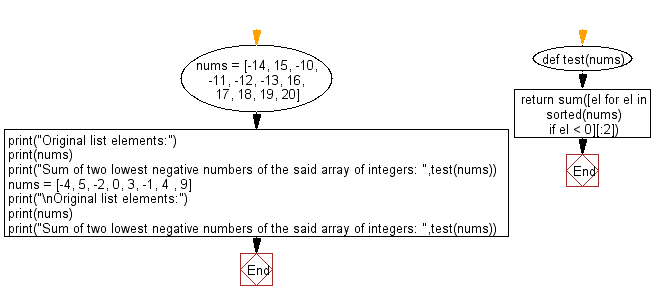
For more Practice: Solve these Related Problems:
- Write a Python program to sum the two highest negative numbers in a given list.
- Write a Python program to sum the two lowest negative numbers and then compute their absolute difference.
- Write a Python program to multiply the two smallest negative numbers together and output the product.
- Write a Python program to identify the two negative numbers with the smallest absolute values and return their sum.
Go to:
Previous: Write a Python program to remove all the values except integer values from a given array of mixed values.
Next: Write a Python program to sort a given positive number in descending/ascending order.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.