Python: Check if a given function returns True for at least one element in the list
Check Any True for Function
Write a Python program to check if a given function returns True for at least one element in the list.
Use any() in combination with map() to check if fn returns True for any element in the list.
Sample Solution:
Python Code:
# Define a function 'some' that takes a list 'lst' and an optional function 'fn'.
# It uses the 'any' function and 'map' to check if any element in the list satisfies the given condition specified by 'fn'.
def some(lst, fn=lambda x: x):
return any(map(fn, lst))
# Call the 'some' function with different lists and conditions, and print the results.
print(some([0, 1, 2, 0], lambda x: x >= 2))
print(some([5, 10, 20, 10], lambda x: x < 2))
Sample Output:
True False
Flowchart:
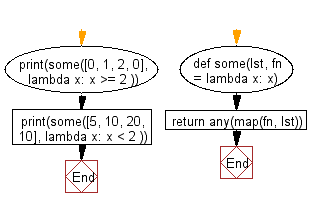
For more Practice: Solve these Related Problems:
- Write a Python program to check if at least one element in a list satisfies a given predicate and return that element.
- Write a Python program to determine if any element in a list is prime using a provided testing function.
- Write a Python program to scan a list and return True as soon as a custom condition is met for any element, otherwise False.
- Write a Python program to verify if any string in a list contains a specific substring by applying a lambda function.
Go to:
Previous: Write a Python program to that takes any number of iterable objects or objects with a length property and returns the longest one.
Next: Write a Python program to calculate the difference between two iterables, without filtering duplicate values.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.