Python: Most frequent element in a given list of numbers
Python List: Exercise - 261 with Solution
Write a Python program to get the most frequent element in a given list of numbers.
- Use set() to get the unique values in nums.
- Use max() to find the element that has the most appearances.
Sample Solution:
Python Code:
# Define a function named 'most_frequent' that finds the most frequently occurring item in a list.
def most_frequent(nums):
return max(set(nums), key=nums.count)
# Using 'set' to remove duplicates, then finding the item with the maximum count using the 'max' function.
# Test the 'most_frequent' function with different lists.
print(most_frequent([1, 2, 1, 2, 3, 2, 1, 4, 2]))
# Find the most frequently occurring item in the list. (Expected output: 2)
nums = [2, 3, 8, 4, 7, 9, 8, 2, 6, 5, 1, 6, 1, 2, 3, 2, 4, 6, 9, 1, 2]
print("Original list:")
print(nums)
print("Item with the maximum frequency of the said list:")
print(most_frequent(nums))
# Find the most frequently occurring item in the list.
nums = [1, 2, 3, 1, 2, 3, 2, 1, 4, 3, 3]
print("\nOriginal list:")
print(nums)
print("Item with the maximum frequency of the said list:")
print(most_frequent(nums))
# Find the most frequently occurring item in the list.
Sample Output:
2 Original list: [2, 3, 8, 4, 7, 9, 8, 2, 6, 5, 1, 6, 1, 2, 3, 2, 4, 6, 9, 1, 2] Item with maximum frequency of the said list: 2 Original list: [1, 2, 3, 1, 2, 3, 2, 1, 4, 3, 3] Item with maximum frequency of the said list: 3
Flowchart:
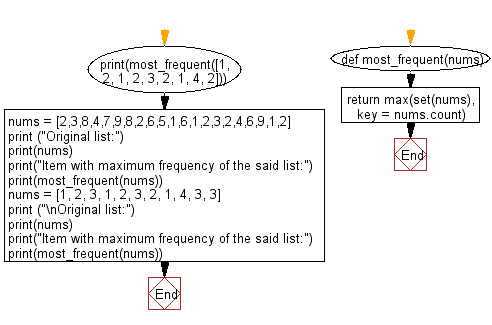
Python Code Editor:
Previous: Write a Python program to check if a given function returns True for at least one element in the list.
Next: Write a Python program to move the specified number of elements to the end of the given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-261.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics