Python Exercises: Extract the first n number of vowels from a string
Python List: Exercise - 279 with Solution
A vowel is a syllabic speech sound pronounced without any stricture in the vocal tract. Vowels are one of the two principal classes of speech sounds, the other being the consonant.
Write a Python program to extract the first specified number of vowels from a given string. If the specified number is less than the number of vowels present in the string then display "n is less than the number of vowels present in the string".
Sample Data:
("Python", 2) -> "n is less than number of vowels present in the string."
("Python Exercises", 3) -> "oEe"
("aeiou") -> "AEI"
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes two inputs: 'text' (a string) and 'n' (an integer).
def test(text, n):
# Initialize an empty string 'result_str' to store the vowels from 'text'.
result_str = ''
# Iterate through each character 'i' in the 'text'.
for i in text:
# Check if 'i' is a vowel (either lowercase or uppercase).
if i in 'aioueAEIOU':
# If 'i' is a vowel, add it to 'result_str'.
result_str += i
# Check if the length of 'result_str' is greater than or equal to 'n'.
# If it is, return the first 'n' vowels from 'result_str'.
# If not, return a message indicating that 'n' is less than the number of vowels in the string.
return result_str[:n] if len(result_str) >= n else 'n is less than the number of vowels present in the string.'
# Define the original string 'word' and the number 'n'.
word = "Python"
n = 2
print("Original string and number:", word, ",", n)
# Call the 'test' function to extract the first 'n' vowels from the string 'word'.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define another string 'word' and a different number 'n'.
word = "Python Exercises"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the new inputs to extract the first 'n' vowels from the string.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define a string 'word' consisting of only vowels and a different number 'n'.
word = "AEIOU"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the vowel-only string and 'n' to extract the first 'n' vowels.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
Sample Output:
Original string and number: Python , 2 Extract the first n number of vowels from the said string: n is less than number of vowels present in the string. Original string and number: Python Exercises , 3 Extract the first n number of vowels from the said string: oEe Original string and number: AEIOU , 3 Extract the first n number of vowels from the said string: AEI
Flowchart:
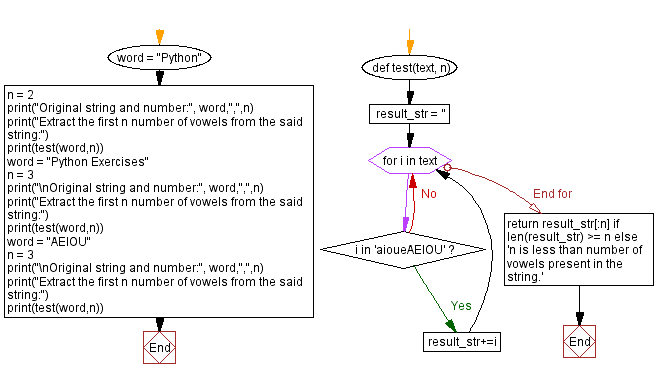
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes two inputs: 'text' (a string) and 'n' (an integer).
def test(text, n):
# Create a list 't' that contains characters from 'text' that are vowels (lowercase or uppercase).
t = [x for x in text if x in 'aeiouAEIOU']
# Check if the length of list 't' is less than 'n'.
# If 'n' is greater than the number of vowels, return a message indicating so.
# If not, join the first 'n' vowels from the list and return the result.
return 'n is less than the number of vowels present in the string.' if len(t) < n else ''.join(t[:n])
# Define the original string 'word' and the number 'n'.
word = "Python"
n = 2
print("Original string and number:", word, ",", n)
# Call the 'test' function to extract the first 'n' vowels from the string 'word'.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define another string 'word' and a different number 'n'.
word = "Python Exercises"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the new inputs to extract the first 'n' vowels from the string.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define a string 'word' consisting of only vowels and a different number 'n'.
word = "AEIOU"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the vowel-only string and 'n' to extract the first 'n' vowels.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
Sample Output:
Original string and number: Python , 2 Extract the first n number of vowels from the said string: n is less than number of vowels present in the string. Original string and number: Python Exercises , 3 Extract the first n number of vowels from the said string: oEe Original string and number: AEIOU , 3 Extract the first n number of vowels from the said string: AEI
Flowchart:
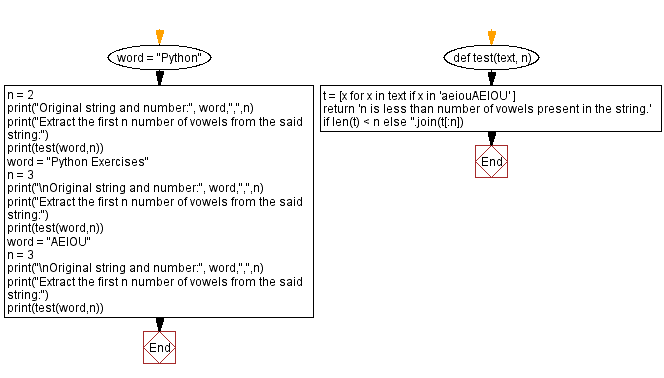
Python Code Editor:
Previous Python Exercise: Sum of missing numbers of a list of integers.
Next Python Exercise: Find all pairs of that differ by three in a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-280.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics