Python Math: Reverse a range
Python Math: Exercise-71 with Solution
Write a Python program to reverse a range.
Sample Solution:
Python Code:
#https://gist.github.com/fcicq/ddb746042150b4e959e6
def reversed_range(start, stop=None, step=1):
if stop is None:
return range(start - step, -step, -step)
else:
new_start = stop - ((stop-start-1) % step + 1)
new_end = new_start - (stop-start+step-1) // step * step
if (stop - start) % step == 0 and step < 0: new_start -= step
return range(new_start, new_end, -step)
print(reversed_range(1, 10, 2))
print(reversed_range(1, 5, 1))
Sample Output:
range(9, -1, -2) range(4, 0, -1)
Flowchart:
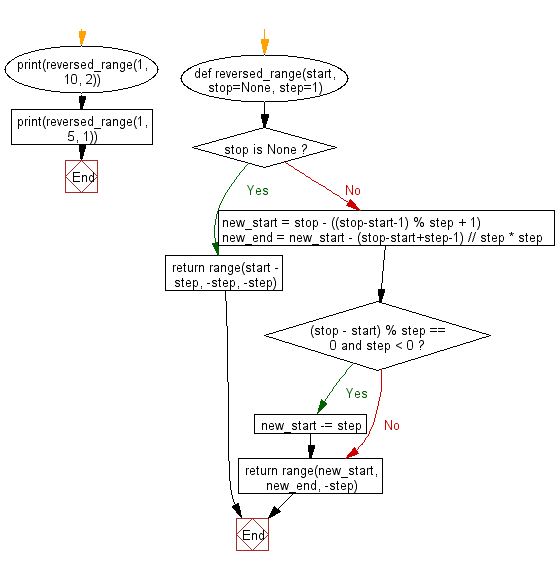
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program for casino simulation.
Next: Write a Python program to create a range for floating numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/math/python-math-exercise-71.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics