Python: Write dictionaries and a list of dictionaries to a given CSV file
7. Write CSV from Dictionaries
Write a Python program to write dictionaries and a list of dictionaries to a given CSV file. Use csv.reader
Sample Solution:
Python Code:
import csv
print("Write dictionaries to a CSV file:")
fw = open("test.csv", "w", newline='')
writer = csv.DictWriter(fw, fieldnames=["Name", "Class"])
writer.writeheader()
writer.writerow({"Name": "Jasmine Barrett", "Class": "V"})
writer.writerow({"Name": "Garry Watson", "Class": "V"})
writer.writerow({"Name": "Courtney Caldwell", "Class": "VI"})
fw.close()
fr = open("test.csv", "r")
csv = csv.reader(fr, delimiter = ",")
for row in csv:
print(row)
fr.close()
Sample Output:
Write dictionaries to a CSV file: ['Name', 'Class'] ['Jasmine Barrett', 'V'] ['Garry Watson', 'V'] ['Courtney Caldwell', 'VI']
Flowchart:
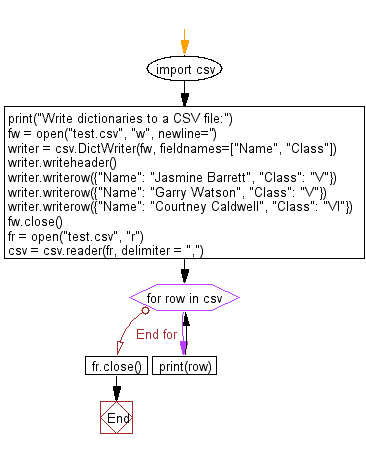
import csv
print("\nWrite a list of dictionaries to a CSV file:")
fw = open("test1.csv", "w", newline='')
writer = csv.DictWriter(fw, fieldnames=["Name", "Class"])
writer.writeheader()
writer.writerows(
[{"Name": "Jasmine Barrett", "Class": "V"},
{"Name": "Garry Watson", "Class": "V"},
{"Name": "Courtney Caldwell", "Class": "VI"}])
fw.close()
fr = open("test1.csv", "r")
csv = csv.reader(fr, delimiter = ",")
for row in csv:
print(row)
fr.close()
Sample Output:
Write a list of dictionaries to a CSV file: ['Name', 'Class'] ['Jasmine Barrett', 'V'] ['Garry Watson', 'V'] ['Courtney Caldwell', 'VI']
Flowchart:
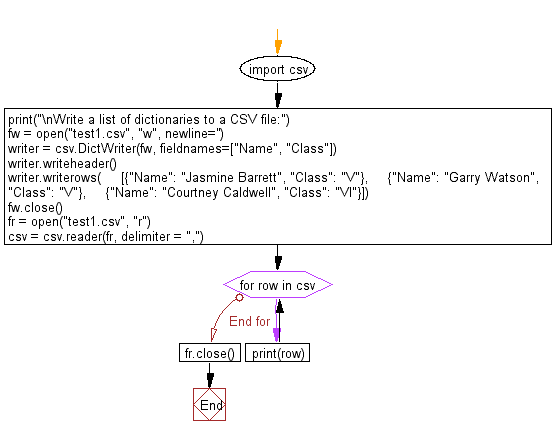
For more Practice: Solve these Related Problems:
- Write a Python program to write a list of dictionaries to a CSV file using csv.DictWriter, then read the file with csv.reader and print each row.
- Write a Python script to convert a dictionary into a CSV file, then open the file using csv.reader and verify that the header matches the dictionary keys.
- Write a Python function to export multiple dictionaries to a CSV file and then read and display the contents using csv.reader.
- Write a Python program to generate a CSV file from a list of dictionaries, ensuring that all dictionaries have the same keys, then read and print the file's rows.
Go to:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Python Code Editor:
Next: Python built-in Modules Exercise Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.