Reshaping a 1D NumPy array into a 2D array
Python Pandas Numpy: Exercise-22 with Solution
Reshape a 1D NumPy array into a 2D array.
Sample Solution:
Python Code:
import numpy as np
# Create a 1D NumPy array
array_1d = np.array([1, 2, 3, 4, 5, 6, 7, 8])
# Reshape into a 2D array with 2 rows and 4 columns
array_2d = np.reshape(array_1d, (2, 4))
# Alternatively, you can use the array's reshape method:
# array_2d = array_1d.reshape((2, 4))
# Display the 2D array
print(array_2d)
Output:
[[1 2 3 4] [5 6 7 8]]
Explanation:
Here's a breakdown of the above code:
- We create a 1D NumPy array "array_1d".
- The np.reshape(array_1d, (2, 4)) function reshapes the array into a 2D array with 2 rows and 4 columns.
- Alternatively, you can use the array's reshape method: array_2d = array_1d.reshape((2, 4)).
- The resulting 2D array (array_2d) is then printed.
Flowchart:
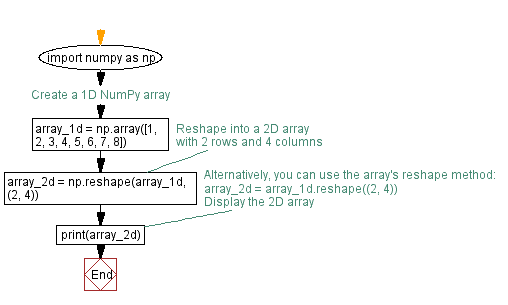
Python Code Editor:
Previous: Calculating the dot product of NumPy arrays.
Next: Slicing and extracting a portion of a NumPy array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pandas_numpy/pandas_numpy-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics