Creating a dialog box in PyQt
Python PyQt Basic: Exercise-10 with Solution
Write a Python program that creates a simple dialog box that displays a message or input form when a button is clicked using PyQt.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QDialog Class: The QDialog class is the base class of dialog windows.
QVBoxLayout Class: This class is used to construct vertical box layout objects.
QLabel Class: The QLabel widget provides a text or image display.
QWidget: The QWidget class is the base class of all user interface objects.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QDialog, QVBoxLayout, QLabel, QWidget
class MyWindow(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Dialog Box Example")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a button that triggers the dialog
dialog_button = QPushButton("Show Dialog")
dialog_button.clicked.connect(self.show_dialog)
# Create a layout for the central widget
layout = QVBoxLayout()
layout.addWidget(dialog_button)
central_widget.setLayout(layout)
def show_dialog(self):
# Create a QDialog instance
dialog = QDialog(self)
dialog.setWindowTitle("Dialog Box")
# Create a label with a message
label = QLabel("This is a message in the dialog box.")
# Create a layout for the dialog
dialog_layout = QVBoxLayout()
dialog_layout.addWidget(label)
# Set the layout for the dialog
dialog.setLayout(dialog_layout)
# Show the dialog as a modal dialog (blocks the main window)
dialog.exec_()
def main():
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a "QMainWindow" (MyWindow) with a central widget.
- Inside the central widget, we create a 'QPushButton' (dialog_button) labeled "Show Dialog" and connect its clicked signal to the show_dialog method.
- In the "show_dialog()" method, we create a QDialog instance (dialog) and set its title.
- Create a "QLabel" (label) with the message we want to display in the dialog.
- Create a QVBoxLayout for the dialog and add the label to it.
- Set the dialog layout using "dialog.setLayout".
- When the "Show Dialog" button is clicked, the show_dialog method is called, which displays the dialog as a modal dialog using “dialog.exec_()”. To interact with the main window again, the dialog must be closed.
Output:
Flowchart:
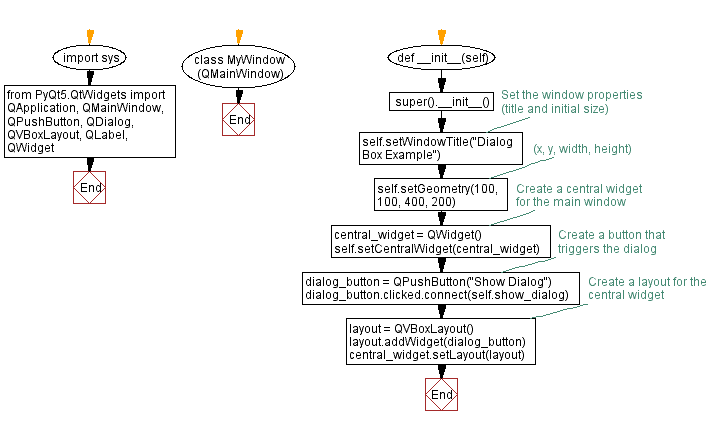
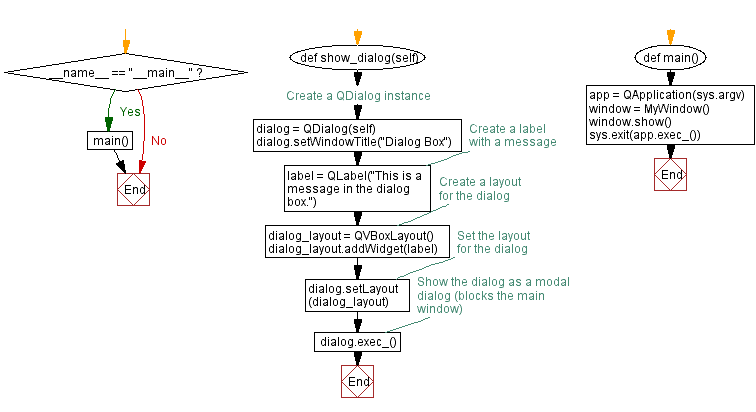
Python Code Editor:
Previous: Customizing widget behavior with PyQt event handling.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/pyqt/python-pyqt-basic-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics