Python Multi-threading and Concurrency: Creating and managing threads
Python Multi-threading: Exercise-1 with Solution
Write a Python program to create multiple threads and print their names.
Sample Solution:
Python Code:
import threading
def print_thread_names():
print("Current thread name:", threading.current_thread().name)
# Create multiple threads
threads = []
for i in range(7):
thread = threading.Thread(target=print_thread_names)
threads.append(thread)
thread.start()
# Wait for all threads to complete
for thread in threads:
thread.join()
Sample Output:
Current thread name: Thread-6 Current thread name: Thread-7 Current thread name: Thread-8 Current thread name: Thread-9 Current thread name: Thread-10 Current thread name: Thread-11 Current thread name: Thread-12
Explanation:
In the above exercise,
- The "print_thread_names()" function is defined, which simply prints the current thread name using threading.current_thread().name.
- We create a list of threads to store thread objects.
- Using a loop, we create 7 threads and add them to the threads list. Each thread is assigned by the "print_thread_names()" function as the target.
- We start each thread by calling its start method.
- After starting all the threads, we use another loop to call the join method on each thread. Prior to exiting, the program waits for all threads to complete.
Flowchart:
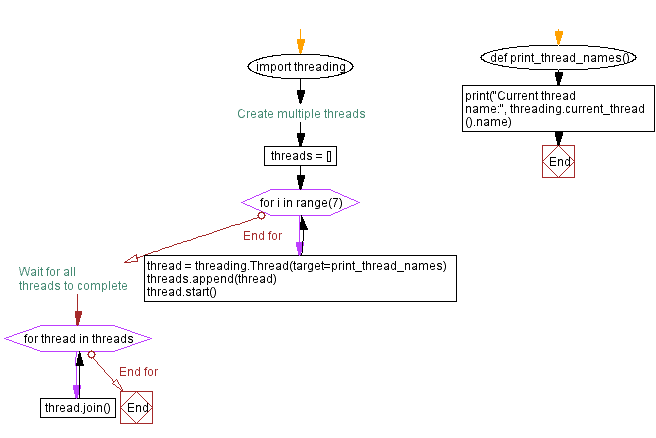
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Multi-threading Exercises Home.
Next: Concurrent file downloading.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics