Python Tkinter Treeview widget: Sorting and columns made easy
Python tkinter widgets: Exercise-18 with Solution
Write a Python GUI program to create a Treeview widget with columns and sorting functionality using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
# Function to sort the Treeview by column
def sort_treeview(tree, col, descending):
data = [(tree.set(item, col), item) for item in tree.get_children('')]
data.sort(reverse=descending)
for index, (val, item) in enumerate(data):
tree.move(item, '', index)
tree.heading(col, command=lambda: sort_treeview(tree, col, not descending))
parent = tk.Tk()
parent.title("Sortable Treeview Example")
# Create a Treeview widget with columns
columns = ("Name", "Age", "Country")
tree = ttk.Treeview(parent, columns=columns, show="headings")
# Configure column headings and sorting functionality
for col in columns:
tree.heading(col, text=col, command=lambda c=col: sort_treeview(tree, c, False))
tree.column(col, width=100)
# Insert data into the Treeview
data = [("Taliesin Megaira", 35, "USA"),
("Fionn Teofilo", 28, "UK"),
("Ata Caishen", 45, "France"),
("Marina Evie", 38, "Spain"),
("Xanthe Eligio", 32, "France"),
("Fausta Tone", 30, "Australia"),
("Yawan Idane", 42, "Mongolia"),
("Rolph Noah", 30, "South Africa")]
for item in data:
tree.insert('', 'end', values=item)
tree.pack()
parent.mainloop()
Explanation:
In the exercise above -
- Import tkinter as 'tk' and 'ttk' for creating the GUI components.
- Define a "sort_treeview()" function that sorts the Treeview by a specified column. Using this function, you can sort a Treeview widget using a column, a boolean flag, and a descending flag.
- Create the main window using tk.Tk() and set its title.
- Create a Treeview widget named tree with specified columns ("Name", "Age", "Country") and hide the default Treeview column headings by setting show="headings".
- Configure the column headings and sorting functionality by iterating through the columns. The heading method is used to set the column heading text, and the command parameter is used to specify sorting behavior when the heading is clicked. The column method sets the width of each column.
- Insert data into the Treeview by providing a list of tuples. Each tuple represents a row of data.
- The "tree.insert()" method adds data rows to the Treeview.
- Pack the Treeview widget into the main window.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
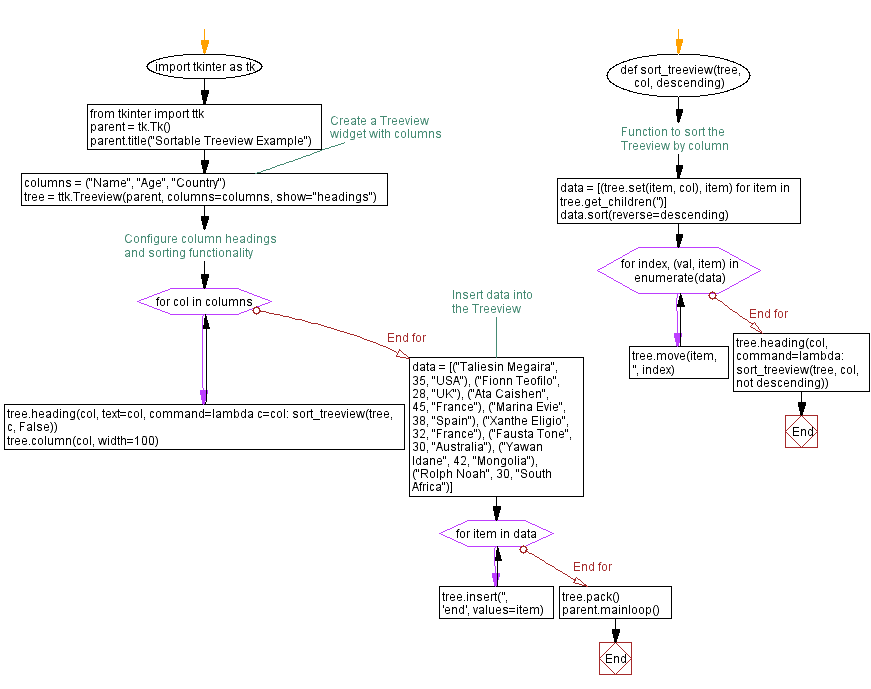
Python Code Editor:
Previous: Selecting dates made easy.
Next: Dynamic percentage updates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics