C Exercises: Find Strong Numbers within a range of numbers
C For Loop: Exercise-48 with Solution
Write a C program to find Strong Numbers within a range of numbers.
Visual Presentation:
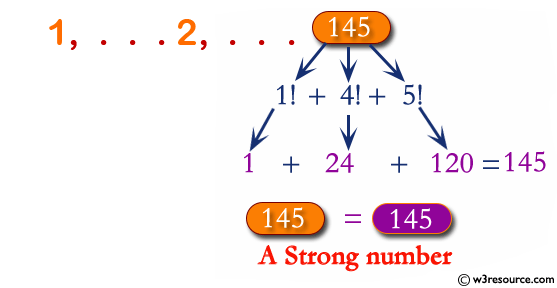
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, n, n1, s1 = 0, j, k, en, sn; // Declare variables to store input and results.
long fact; // Declare a variable to store factorial.
printf("\n\nFind Strong Numbers within a range of numbers:\n "); // Print a message.
printf("------------------------------------------------------\n"); // Print a separator.
// Prompt the user for the starting and ending range of numbers.
printf("Input starting range of number : ");
scanf("%d", &sn); // Read the starting range.
printf("Input ending range of number: ");
scanf("%d", &en); // Read the ending range.
printf("\n\nThe Strong numbers are :\n"); // Print a message.
// Loop to process each number in the given range.
for (k = sn; k <= en; k++)
{
n1 = k; // Store the original number for comparison.
s1 = 0; // Reset the factorial sum.
// Loop to process each digit of the number.
for (j = k; j > 0; j = j / 10)
{
fact = 1; // Initialize factorial.
// Loop to calculate factorial of a digit.
for (i = 1; i <= j % 10; i++)
{
fact = fact * i;
}
s1 = s1 + fact; // Accumulate factorial sum.
}
if (s1 == n1)
{
printf("%d ", n1); // Print the strong number.
}
}
printf("\n\n"); // Print two new lines.
}
Sample Output:
Find Strong Numbers within an range of numbers: ------------------------------------------------------ Input starting range of number : 1 Input ending range of number: 200 The Strong numbers are : 1 2 145
Flowchart:
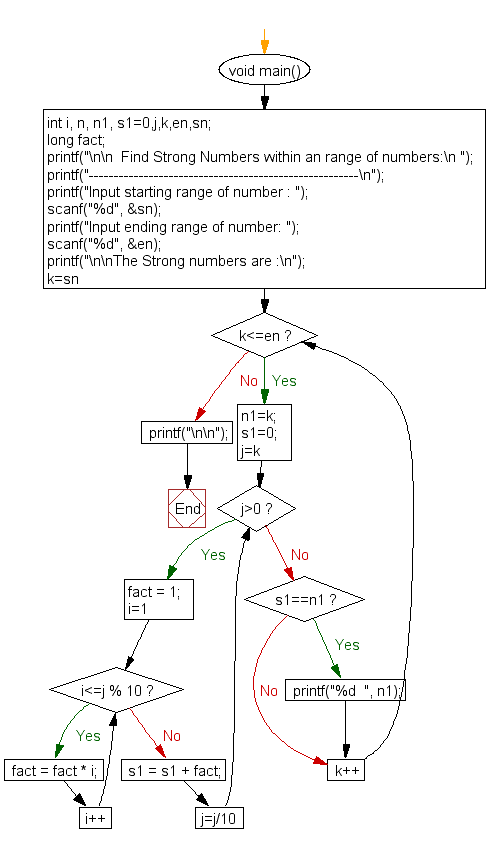
C Programming Code Editor:
Previous: Write a C program to check whether a number is a Strong Number or not.
Next: Write a c program to find out the sum of an A.P. series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics