C Exercises: Find out the sum of an A.P. series
49. Sum of A.P. Series
Write a C program to find the sum of an A.P. series.
An Arithmetic Progression (A.P.) series is a sequence of numbers where the difference between any two consecutive terms is constant. It follows a specific pattern where each term is obtained by adding a fixed number to the previous term. For example, in the series 2, 5, 8, 11, 14, each term increases by 3, indicating a common difference of 3.
In this program, you find the sum of an Arithmetic Progression (A.P.) series:
- Input the first term (a), the common difference (d), and the number of terms (n).
- Apply the formula for the sum of an A.P.: sum = (n/2) [2a + (n - 1) d].
- Output the computed sum.
Visual Presentation:
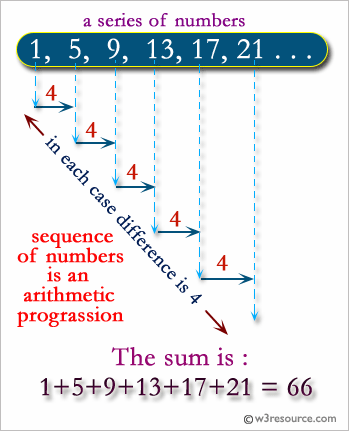
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <math.h> // Include the math header file.
void main(){
int n1, df, n2, i, ln; // Declare variables to store input and results.
int s1 = 0; // Initialize the sum of the arithmetic progression.
printf("\n\nFind out the sum of A.P. series :\n "); // Print a message.
printf("----------------------------------------\n"); // Print a separator.
printf("Input the starting number of the A.P. series: "); // Prompt the user for the starting number.
scanf("%d", &n1); // Read the starting number.
printf("Input the number of items for the A.P. series: "); // Prompt the user for the number of items in the series.
scanf("%d", &n2); // Read the number of items.
printf("Input the common difference of A.P. series: "); // Prompt the user for the common difference.
scanf("%d", &df); // Read the common difference.
// Calculate the sum of the arithmetic progression.
s1 = (n2 * (2 * n1 + (n2 - 1) * df)) / 2;
ln = n1 + (n2 - 1) * df; // Calculate the last term of the series.
printf("\nThe Sum of the A.P. series are : \n"); // Print a message.
// Loop to print the terms of the arithmetic progression.
for (i = n1; i <= ln; i = i + df) {
if (i != ln)
printf("%d + ", i); // Print the term and a plus sign.
else
printf("%d = %d \n\n", i, s1); // Print the last term and the total sum.
}
}
Output:
Find out the sum of A.P. series : ---------------------------------------- Input the starting number of the A.P. series: 1 Input the number of items for the A.P. series: 10 Input the common difference of A.P. series: 4 The Sum of the A.P. series are : 1 + 5 + 9 + 13 + 17 + 21 + 25 + 29 + 33 + 37 = 190
Flowchart:
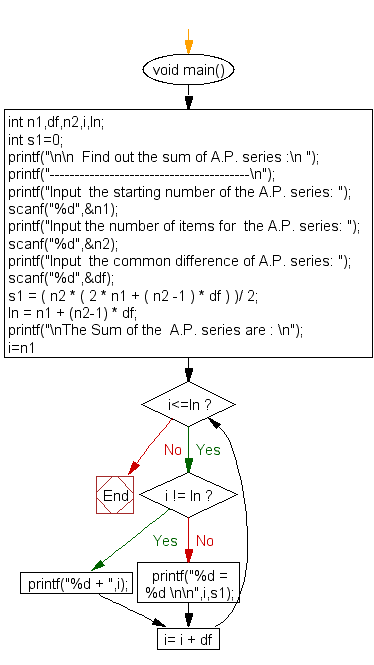
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of an arithmetic progression using the formula and then display the series.
- Write a C program to compute the sum of an A.P. series and determine the average of its terms.
- Write a C program to generate the terms of an A.P. series recursively and compute their sum.
- Write a C program to calculate the sum of an A.P. series with error checking for a zero common difference.
C Programming Code Editor:
Previous: Write a C program to find Strong Numbers within a range of numbers.
Next: Write a program in C to convert a decimal number into octal without using an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.